Kangaroo Rewards - API Reference
API Endpoint
https://api.kangaroorewards.comNote. If you are looking for Users API Reference, it is awailable at User API Reference
The Kangaroo Rewards API uses HTTP methods and a RESTful endpoint structure. The API authorization framework is OAuth 2.0. You format requests in JSON and the APIs return JSON-formatted responses.
Developers can build an app using Kangaroo Rewards API in their language of choice. To simplify integrations, Kangaroo Rewards provides REST Server SDKs and samples available on Github (in PHP).
KangarooRewards-PHP-SDK - the SDK without Oauth2 support (you will need to handle authentication and request an access token using another library, or by making API calls directly to the authentication endpoints)
OAuth2 Kangaroo Rewards - the OAuth2 Client for Kangaroo Rewards API
REST API sample App - a sample app built in PHP using the SDK: KangarooRewards-PHP-SDK and OAuth2 client: OAuth2 Kangaroo Rewards that helps you to build your app faster.
Build Your First App Following These Steps
-
Step 1: Register an app with Kangaroo Rewards
-
Step 2: Implement Authentication
-
Step 3: Access Kangaroo Rewards REST API
Prerequisite. To get started, you will need a Kangaroo Rewards Developer account with which to build and test your app. Sign up for free at: api.kangaroorewards.com/developers
App capabilities
Your app can do these things and more.
-
Access your account information
-
Access Business information including branches, offers, rewards etc.
-
List all Customers and new Customers
-
Reward your customers
-
List Custom Rewards and Catalog Items
-
Redeem based on amount, points or Catalog Item
The following steps show how your application interacts with Kangaroo’s OAuth 2.0 server to obtain a user’s consent to perform an API request on the user’s behalf. Your application must have that consent before it can execute a Kangaroo API request that requires user authorization.
The list below quickly summarizes these steps:
-
Your application identifies the permissions it needs.
-
Your application redirects the user to Kangaroo Rewards along with the list of requested permissions.
-
The user decides whether to grant the permissions to your application.
-
Your application finds out what the user decided.
-
If the user granted the requested permissions, your application retrieves tokens needed to make API requests on the user’s behalf.
Register an app with Kangaroo Rewards
Go to Developer Portal. Click on the “Register a new Application” button. Consider using a service account when registering your app at Developer Portal. i.e. “apps@yourcompany.com” - This will ensure your app continues to work if you or the app creator leaves the company.
Register a New App - Fields | Description |
---|---|
Application name | The app name which will appear on Authorization dialog. |
Authorization callback URL | This must be the page url where the users will be redirected after authorizing your application. Also, The Access Token will be sent to this page |
Application description | Optional. This is what the users will see on Authentication page |
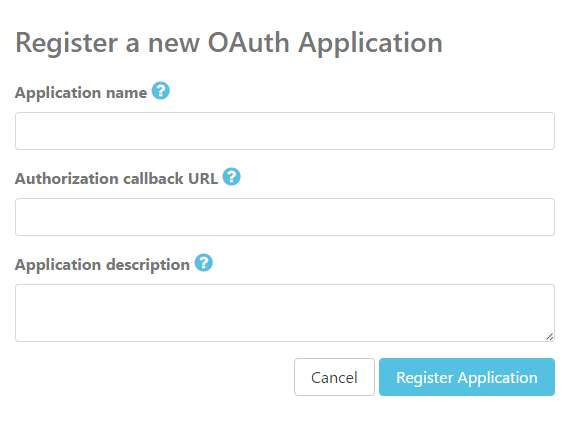
Once the application is created, it will have a Client ID and Client Secret associated to it. The Client ID is public, but Client Secret must not be shared.
Note: By default, the application you recently created is NOT Approved. Please contact Kangaroo Rewards Support and get the application APPROVED before continuing with the Authentication implementation.
After you finished building your app and tested it, you may request authorization from the person you are developing the application. He/She will normally be a Business Owner and will receive an email with authorization link.
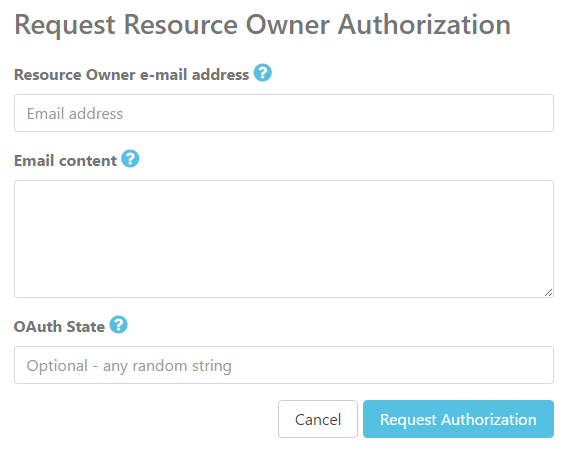
Note: OAuth state is a random string that is optional, but highly recommended to mitigate CSRF attacks. If you decide to use state parameter in your app and request authorization by email, you have to store the state parameter value in your callback page. A sample code for callback page can be found here
Implement Authentication
OAuth 2 is an open standard for authorization that enables third-party applications to obtain limited access to Kangaroo Rewards merchant accounts, by delegating user authentication to Kangaroo Rewards.
All developers need to register their application before getting started. A registered OAuth application is assigned a unique Client ID and Client Secret. The Client Secret should not be shared.
Currently Kangaroo Rewards API supports 2 types of authorization:
NOTE: When creating a new application please make sure to select the corresponding grant type for your application
- For
Authorization Code
flow the Grant Type MUST be Authorization Code- For
Resource Owner Credentials
flow the Grant Type MUST be Password Grant
OAuth - Web Application Flow
The Kangaroo Rewards OAuth API supports the Authorization Code flow, which is suitable for web applications running on a server. This is a description of the flow for third party applications.
This scenario begins by redirecting a browser (full page or popup) to a Kangaroo Rewards URL with a set of query parameters that indicate the type of Kangaroo Rewards API access the application requires. As in other scenarios, Kangaroo Rewards handles user authentication and consent, and the result is an access token
The application may access a Kangaroo Rewards API after it receives the access token.
Note: Your application should always use HTTPS in this scenario.
1. Request Authorization
The URL used when authenticating a user is https://api.kangaroorewards.com/oauth/authorize
. This endpoint is accessible over SSL, and HTTP connections are refused.
Auth Code Request Endpoint
https://api.kangaroorewards.com/oauth/authorize
The set of query string parameters supported by the Kangaroo Authorization Server for client-side applications are:
Parameter | Values | Description |
---|---|---|
client_id | The client ID you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
redirect_uri | The URL in your application where users will be sent after authorization. | Determines where the response is sent. The value of this parameter must exactly match the url listed for this app in the Developer Portal (including the http or https scheme, case, and trailing '/'). |
response_type | code | Must be set to "code" to request an authorization code. |
scope | Space-delimited set of permissions that the application requests. | A space delimited list of scopes. Identifies the Kangaroo API access that your application is requesting. The values passed in this parameter inform the consent screen that is shown to the user. There is an inverse relationship between the number of permissions requested and the likelihood of obtaining user consent. For information about available scopes, see scopes. |
state | Random string | Optional. This parameter is optional but highly recommended. You should store the value of the CSRF token in the user’s session to be validated when they return. |
All of these parameters will be validated by the authorization server.
The user will then be asked to login to the authorization server and approve the client.
An example URL is shown below
https://api.kangaroorewards.com/oauth/authorize?client_id=12345
&redirect_uri=https://example.com/callback
&response_type=code
&scope=admin
2. Handling the OAuth 2.0 server response
If the user approves the client they will be redirected from the authorisation server back to the client (specifically to the redirect URI) with the following parameters in the query string:
-
code with the authorization code
-
state with the state parameter sent in the original request. You should compare this value with the value stored in the user’s session to ensure the authorization code obtained is in response to requests made by this client rather than another client application.
An error response:
https://example.com/callback?error=access_denied&state={CSRF}
An authorization code response:
https://example.com/callback?code=OtHIETVfgY4lQz9IlTx7E3HPmuCVQb8IubZ
After the web server receives the authorization code, it can exchange the authorization code for an access token.
3. Request Access Token
The client will now send a POST request to the authorization server with the following parameters:
Access Token Request Endpoint:
https://api.kangaroorewards.com/oauth/token
Parameter | Values | Description |
---|---|---|
client_id | The client ID you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
client_secret | The client secret you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
redirect_uri | The URL in your application where users will be sent after authorization. | Determines where the response is sent. The value of this parameter must exactly match the url listed for this app in the Developer Portal (including the http or https scheme, case, and trailing '/'). |
grant_type | authorization_code | The authorization code |
code | Authorization code | The code you received as a response to Step 1 |
Example Access Token Endpoint
> POST /oauth/token HTTP/2
> Host: api.kangaroorewards.com
> User-Agent: curl/7.65.1
> Accept: application/x-www-form-urlencoded
> Content-Type: application/x-www-form-urlencoded
> X-Application-Key: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9
> Content-Length: 366
| grant_type=authorization_code&client_id=12345&client_secret=8fysa8dfds9fj283&code=def502009ac5c81&redirect_uri=https://example.com/callback&scope=admin
Example with curl
curl -v -X POST https://api.kangaroorewards.com/oauth/token \
-H "Accept: application/x-www-form-urlencoded" \
-H "Content-Type: application/x-www-form-urlencoded" \
-H "X-Application-Key: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9..." \
-d "grant_type=authorization_code \
&code=def502009ac5c81 \
&client_id=12345 \
&client_secret=8fysa8dfds9fj283 \
&redirect_uri=https://example.com/callback \
&scope=admin"
By default, the response will take the following form:
access_token=eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1N&scope=admin&token_type=Bearer
A successful response is returned as a JSON array, similar to the following:
{
"access_token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImI5ZTk0NTRkYTRmOTY5Y2U2ZTUxMmFlMTRkZTdkMWJhZWQ1NjUyZTcwNmNiOTk2YTE2MjdhMjg0ZDMzMTM2NDNlZmJlZjhiZWU4YWQxMDg4In0",
"refresh_token":"2riNuUlbhlW1MWKb4Jos9FOYgIzz5ZJtK7rQjIgLDu3XsgblD5Xtn44sFieo",
"expires_in":3920,
"token_type":"Bearer"
}
4. Use Access Token Now you may use the access token to make requests, on behalf of the user, from the resource server via the API (endpoint: https://api.kangaroorewards.com).
The access token may be used until it expires (24 hours after being issued) or is otherwise invalidated (e.g. user revoked or refresh token used).
Example Access Token Use
curl -H "Authorization: Bearer 12345678900987654321-abc34135acde13f13530" https://api.kangaroorewards.com/customers
OAuth - Refresh The Access Token Flow
Access tokens have limited lifetimes. If your application needs access to the Kangaroo API beyond the lifetime of a single access token, it can obtain a refresh token. A refresh token allows your application to obtain new access tokens.
If you would like to request a new access token (and new refresh token), you may do so by sending the authorization server a token refresh request. A typical reason for refreshing a token is that the original access token has expired. A refresh token may only be used once, and using it invalidates the access token that it was issued with.
Use Refresh Token grant type
Use the refresh_token in your token refresh request, which is a POST request to the token endpoint with the appropriate parameters.
https://api.kangaroorewards.com/oauth/token
Parameter | Values | Description |
---|---|---|
grant_type | refresh_token |
Must be set to “refresh_token” for an token refresh request. |
refresh_token | def502009d9337d94c8b7d67b05b9d05afb1b6... |
The refresh_token that was received with the original access token. |
client_id | Your client ID | The value passed in this parameter must exactly match the value shown in the Developer Portal |
client_secret | Your client secret | The client secret you obtain from the Developer Portal |
scope | admin |
A list of scopes. Currently we support only one scope, “admin” |
Example Refresh Token Request
Headers
> Content-Type: application/x-www-form-urlencoded
> X-Application-Key: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...
POST https://api.kangaroorewards.com/oauth/token?grant_type=refresh_token
&refresh_token=def502009d9337d94c8b7d67b05b9d05afb1b6
&client_id=12345
&client_secret=8fysa8dfds9fj283
&scope=admin
Token Refresh Response
The refresh token response looks just like the normal access token grant. It includes new access and refresh tokens.
Example Refresh Token Request
curl --request POST \
--url http://api.kangaroorewards.com/oauth/token \
--header 'content-type: application/x-www-form-urlencoded' \
--header 'x-application-key: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9' \
--data 'grant_type=refresh_token&refresh_token=def50200ceef1ff4664d517&client_id=1111&client_secret=cJ57RFk2CibH4o2INJb3L6&scope=admin'
Store the Refresh Token
The application should store the refresh token for future use and use the access token to access the Kangaroo API. Once the access token expires, the application uses the refresh token to obtain a new one.
Note: Save refresh tokens in secure long-term storage and continue to use them as long as they remain valid.
Refresh token expiration
You must write your code to anticipate the possibility that a granted refresh token might no longer work. A refresh token might stop working for one of these reasons:
-
The user has revoked your app’s access.
-
The refresh token has not been used for 30 days.
Note: A Refresh Token will expie in 30 days. After 30 days the Refresh Token MUST be refreshed or a new Refresh Token can be obtained by requesting a new Access Token
OAuth - Password Credentials Grant
The Kangaroo Rewards OAuth API also supports the Password Credentials Grant flow, where the resource owner has a trust relationship with the client.
This grant type is suitable for clients capable of obtaining the resource owner’s credentials (username and password, typically using an interactive form).
The application may access a Kangaroo Rewards API after it receives the access token.
Note: Your application should always use HTTPS in this scenario.
1. Requesting Tokens
Access Token Request Endpoint:
https://api.kangaroorewards.com/oauth/token
Once you have created an application with type password grant
, you may request an access token by issuing a POST request to
the /oauth/token
route with the user’s email address and password. If the request is successful, you will receive an access_token
and refresh_token in the JSON response from the server.
Parameter | Values | Description |
---|---|---|
client_id | The client ID you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
client_secret | The client secret you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
grant_type | password | For password grant |
username | john@example.com | The email address |
password | YourPass$ord | The password |
scope | admin |
Scopes allow the clients to request a specific set of permissions when requesting authorization to access an account |
application_key | The Application Key | The business owner has to provide The Application Key. They can get it from the business portal. |
Note: For oauth/token endpoint the
Content-Type
header must be:application/x-www-form-urlencoded
Example Token Request
$http = new GuzzleHttp\Client;
$response = $http->post('https://api.kangaroorewards.com/oauth/token', [
'form_params' => [
'grant_type' => 'password',
'client_id' => '{YOUR_CLIENT_ID}',
'client_secret' => '{YOUR_CLIENT_SECRET}',
'username' => 'john@example.com',
'password' => 'your-super-password',
'application_key' => '{YOUR_APPLICATION_KEY}',
'scope' => 'admin',
],
]);
$token = json_decode((string) $response->getBody(), true);
print_r($token);
Curl Example:
curl --request POST \
--url https://api.kangaroorewards.com/oauth/token \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data grant_type=password \
--data client_id={YOUR_CLIENT_ID} \
--data client_secret={YOUR_CLIENT_SECRET} \
--data username=john@example.com \
--data password=your-super-password \
--data scope=admin \
--data application_key={YOUR_APPLICATION_KEY}
A successful response is returned as a JSON array, similar to the following:
{
"access_token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImI5ZTk0NTRkYTRmOTY5Y2U2ZTUxMmFlMTRkZTdkMWJhZWQ1NjUyZTcwNmNiOTk2YTE2MjdhMjg0ZDMzMTM2NDNlZmJlZjhiZWU4YWQxMDg4In0",
"refresh_token":"2riNuUlbhlW1MWKb4Jos9FOYgIzz5ZJtK7rQjIgLDu3XsgblD5Xtn44sFieo",
"expires_in":3920,
"token_type":"Bearer"
}
Scopes
Scopes limit the type of access that an access token will allow. The Kangaroo Rewards API currently supports one scope, “admin”.
Name | Description |
---|---|
(no scope) | Is not allowed |
admin | Grants read/write access to business account, i.e. full access. This allows actions that can be requested using the GET, POST, PUT, and DELETE methods |
Access Kangaroo Rewards REST API
The Kangaroo Rewards API allows you to manage resources in a simple, programmatic way using conventional HTTP requests. The endpoints are intuitive and powerful, allowing you to easily make calls to retrieve information.
All of the functionality that you are familiar with in the Kangaroo Rewards App is also available through the API, allowing you to script the complex actions that your situation requires.
Note: The X-Application-Key header is required for all requests
NOTE: All POST, PUT and PATCH requests MUST have the content type header set to
application/json
PUT /users/me/settings/notifications HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyJ0eXAi...
Authorization: Bearer eyJ0eXAiOiJKV1QiL...
Content-Type: application/json; charset=UTF-8
Requests
Method | Usage |
---|---|
GET | For simple retrieval of information about your account, offers, customers etc., you should use the GET method. The information you request will be returned to you as a JSON object. The attributes defined by the JSON object can be used to form additional requests. Any request using the GET method is read-only and will not affect any of the objects you are querying. |
POST | To create a new object, your request should specify the POST method. The POST request includes all of the attributes necessary to create a new object. When you wish to create a new object, send a POST request to the target endpoint. |
PUT | To update the information about a resource in your account, the PUT method is available. |
DELETE | To destroy a resource and remove it from your account, the DELETE method should be used. This will remove the specified object if it is found. If it is not found, the operation will return a response indicating that the object was not found (404 error code). |
HTTP Statuses
Along with the HTTP methods that the API responds to, it will also return standard HTTP statuses, including error codes.
In the event of a problem, the status will contain the error code, while the body of the response will usually contain additional information about the problem that was encountered.
In general, if the status returned is in the 200
range, it indicates that the request was fulfilled
successfully and that no error was encountered.
Return codes in the 400
range typically indicate that there was an issue with the request that was sent.
Among other things, this could mean that you did not authenticate correctly, that you are requesting an action that you do not have authorization for, that the object you are requesting does not exist, or that your request is malformed.
If you receive a status in the 500
range, this generally indicates a server-side problem. This means that
we are having an issue on our end and cannot fulfill your request currently.
Responses
When a request is successful, a response body will typically be sent back in the form of a JSON object. An exception to this is when a DELETE request is processed, which will result in a successful HTTP 204 status and an empty response body.
Example Response
{
"data": {
"name": "john"
. . .
}
}
Meta
In addition to the main resource root, the response MAY also contain a meta object. This object contains information about the response itself. The meta object MIGHT contain a total key that is set to the total number of objects returned by the request. This has implications on the pagination. The meta object MIGHT not be presend or it will only be displayed when it has a value. Currently, the meta object will have a value when a request is made on a collection (like customers).
Pagination
The meta object is returned as part of the response body when pagination is enabled. By default, 100 objects are returned per page. You can request a different page by appending ?page= to the request. For instance, to navigate to page 2, you could add ?page=2 to the end of your query
Example:
{
"data": {
. . .
},
"meta": {
"total": 509
}
}
Rate Limit
The number of requests that can be made through the API is currently limited to 240 per minute per OAuth token or IP. The rate limiting information is contained within the response headers of each request. The relevant headers are:
-
X-RateLimit-Limit - The number of requests that can be made per minute.
-
X-RateLimit-Remaining - The number of requests that remain before you hit your request limit.
-
X-RateLimit-Reset - This represents the time when you are allowed to make requests again. The value
-
is given in Unix epoch time You’ll only get X-RateLimit-Reset if you’ve hit the limit
Example
X-RateLimit-Limit: 239
X-RateLimit-Remaining: 0
X-RateLimit-Reset: 1476571703
Best Practices
-
Read the Documentation: Understand the API’s endpoints, parameters, authentication methods, rate limits, and response formats.
-
Authenticate Properly: Use the correct authentication method (e.g., OAuth2 Password Credentials, Authorization Code) and handle authentication securely.
-
Respect Rate Limits: Adhere to the API’s rate limits to avoid being throttled or blocked. Implement backoff strategies if rate limits are exceeded.
-
Refresh Tokens: When using OAuth for authentication, especially for long-lived sessions or when accessing resources on behalf of a user, utilize refresh tokens. These tokens can be exchanged for new access tokens once they expire, without requiring the user to reauthenticate. This ensures uninterrupted access to the API while maintaining security.
-
Error Handling: Handle errors gracefully by checking for error responses and implementing appropriate error-handling mechanisms.
-
Security: Ensure data security by using
HTTPS
, validating user input, and sanitizing data to prevent injection attacks. -
Versioning: Keep track of API version changes and update your code accordingly to maintain compatibility.
-
Optimize Requests: Minimize unnecessary requests by batching or caching data when possible to reduce latency and improve performance.
-
Monitor Usage: Monitor API usage to identify any issues or performance bottlenecks and optimize accordingly.
-
Handle Pagination: Implement pagination when working with large datasets to retrieve data efficiently in manageable chunks.
-
Feedback and Support: Provide feedback on usability, documentation, and feature requests. Utilize support channels if you encounter issues or have questions.
By following these best practices, you can ensure efficient, secure, and reliable integration with Kangaroo Rewards’ APIs.
Account
Account ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"profile": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
},
"business": {
"id": "22e696103f8fa5eabb02089e01cf89c1",
"name": "Retrorant",
"loyalty_type": "per_visit",
"about": "Retrorant has cuisines in houses across the city.",
"logo": "https://www.kangaroorewards.com/photos/1473392787_57d23093693fe.jpg",
"cover": "https://www.kangaroorewards.com/photos/1465232980_5755ae54d57635.61967004.jpg"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"profile": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
},
"business": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"loyalty_type": {
"type": "string"
},
"about": {
"type": "string"
},
"logo": {
"type": "string"
},
"cover": {
"type": "string"
}
}
}
}
}
}
}
GET/me
This is an object representing your Kangaroo account. You can retrieve it to see properties on the account like its current e-mail address, settings etc.
Example
GET /me HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
NOTE: You could also request more information using include parameter. All possible values:
- offers - include business offers in the response
- products - include products in the response
- giftcards - include gift cards in the response
- catalog_items - include Redemption Catalog in the response
- social_media - include social media links in the response
- branches - include business branches in the response
Example
https://api.kangaroorewards.com/me?include=branches,products,offers
Customers ¶
Customer Collection ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "11e696103f8ff5eabb02089e01cf89b5",
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"phone": "5141234567",
"birth_date": "1963-03-28",
"gender": "male",
"balance": {
"points": 135,
"giftcard": 21.35
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Retrieve all customersGET/customers
Returns a list of your customers
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
created_at | string | Optional. Filter by created date. See Filtering |
updated_at | string | Optional. Filter by updated date. See Filtering |
string | Optional. Filter by email address. See Filtering |
|
phone | string | Optional. Filter by phone number. See Filtering |
include | string | Optional. You can optionally request that the response includes other related resources. To do so, specify include=balance in your request. |
Include related resources
- balance - includes customer’s balance for business in the response
NOTE: The value of the include or relationships parameter MUST be a comma-separated (",") list of relationship names.
Examples
GET /customers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": "11E696103F8FF5EABB02089E01CF89B5",
"email": "john1@example.com",
"phone": "5140000001",
"first_name": "Adam",
"last_name": "Pappas"
},
{
"id": "11E696103F8FD85CBB02089E01CF89B5",
"email": "john2@example.com",
"phone": "5140000002",
"first_name": "Alain",
"last_name": "Trepanier"
}
. . .
],
"cursor": {
"count": 100,
"next": 2,
"next_uri": "/customers?page=2"
},
"meta": {
"total": 145
}
}
Example with balance
GET /customers?include=balance HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": "11E696103F8FF5EABB02089E01CF89B5",
"email": "john1@example.com",
"phone": "5140000001",
"first_name": "Adam",
"last_name": "Pappas",
"balance": {
"points": 100,
"giftcard": 0
}
}
. . .
]
}
Filtering
When submitting a GET request, you can ask it to return only customers that meet specific criteria.
To filter customers, in the query string, specify one or more fields with an operator, followed by a separator (|
)
and the values for the following:
Fields:
-
created_at
- Filter by created date -
updated_at
- Filter by updated date -
email
- Filter by email address -
phone
- Filter by phone number
Operators:
-
gt
- Greater Than -
gte
- Greater Than or Equal -
lt
- Less Than -
lte
- Less Than or Equal -
eq
- Equal
Filter Syntax
A single filter uses the form: field=operator|VALUE
Each URL query parameter must be URL encoded.
Example: Retrieve all customers that have created date greather than 2017-01-27T14:37:16+0000
GET /customers?created_at=gt|2023-01-27T14:37:16+0000
Example: Retrieve all customers that have email address equal to john@example.com
GET /customers?email=eq|john@example.com
Example: Retrieve all customers that have phone number equal to 5141234567
GET /customers?phone=eq|5141234567
Customer Collection ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"first_name": "John",
"last_name": "Doe",
"email": "johndoe@example.com",
"birth_date": "1963-03-28",
"language": "en",
"gender": "male"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
},
"email": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"language": {
"type": "string",
"description": "Communication language. Possible values `en` - English, `fr` - French, `es` - Spanish"
},
"gender": {
"type": "string",
"description": "Customer's gender. Can be male or female"
}
},
"required": [
"email"
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"phone": "5141234567",
"birth_date": "1963-03-28",
"gender": "male",
"balance": {
"points": 135,
"giftcard": 21.35
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"first_name": {
"type": "string",
"description": "First name for the Customer."
},
"last_name": {
"type": "string",
"description": "Last name for the Customer."
},
"email": {
"type": "string"
},
"phone": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"gender": {
"type": "string",
"description": "Customer's gender. Can be male or female"
},
"balance": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"giftcard": {
"type": "number"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"description": "Bad Request",
"message": "Invalid ID supplied"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"description": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"email": [
"The email field is required"
],
"phone": [
"The phone field is required"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"email": {
"type": "array"
},
"phone": {
"type": "array"
}
}
}
Create a customerPOST/customers
Create a customer.
Body parameters
Name | Type | Description |
---|---|---|
string | Required. Required if no phone number provided | |
phone | string | Required. Required if no email provided |
country_code | string | Required with phone. The customer's country, in ISO 3166-1-alpha-2 format. |
first_name | string | Optional. First Name |
last_name | string | Optional. Last Name |
language | string | Optional. Communication language. Possible values en - English, fr - French, es - Spanish |
gender | string | Optional. Possible values male , female |
referral | object | Optional. Referral object |
referral.referral_program_id | integer | Required with `referral`. Referral Program ID |
referral.referrer_id | string | Required with `referral`. Referrer ID (Customer ID) |
Example
POST /customers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{ "email" : "john2@example.com", "first_name": "John", "last_name": "Doe" }
Respone Body
{
"data": {
"id": "11e69610410894b2bb02089e01cf89b5",
"email": "johndoe@example.com",
"phone": "5140000001",
"first_name": "John",
"last_name": "Doe"
}
}
Example. Create a customer from referral.
Note: Referral program is required and must be active.
Referrer id (customer id who referred) is required
Referral record must not exist
POST /customers
{
"email": "john@example.com",
"referral": {
"referral_program_id": 1056,
"referrer_id": "11eaa697ae8f46bd9df9b42e99312ac8"
}
}
Customer Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"phone": "5141234567",
"birth_date": "1963-03-28",
"gender": "male",
"balance": {
"points": 135,
"giftcard": 21.35
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"first_name": {
"type": "string",
"description": "First name for the Customer."
},
"last_name": {
"type": "string",
"description": "Last name for the Customer."
},
"email": {
"type": "string"
},
"phone": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"gender": {
"type": "string",
"description": "Customer's gender. Can be male or female"
},
"balance": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"giftcard": {
"type": "number"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Customer not found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Retrieve a customerGET/customers/{id}{?include}
Returns a single customer.
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
include | string | Optional. You can optionally request that the response includes other resources. To do so, specify include=balance in your request. |
relationships | string | Optional. You can optionally request that the response includes other related resources. To do so, specify relationships=address in your request. |
NOTE: You could also request more information using include or relationships parameter.
Include:
- balance - includes customer’s balance for business in the response
- tier_level - includes customer’s current tier level in the response
- tier_progress - includes customer’s tier progress
- tiers - includes tiers
- referral_programs - includes referral programs and referral link for customer
Relationships:
- tier_levels - includes tier levels in tier resource. Only with include=tiers
- user_type - includes user type resource
- address - includes customer’s address in the response
NOTE: The value of the include or relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /customers/11e69610400894b2bb02089e01cf89b5?include=balance,tier_level HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": {
"id": "11e69610400894b2bb02089e01cf89b5",
"email": "johndoe@example.com",
"phone": "5140000001",
"first_name": "John",
"last_name": "Doe"
},
"included": {
"balance": {
"points": 1000,
"giftcard": 10.00
},
"tier_level": {
"id": 1,
"name": "Platinum",
"reach_spend": 2,
"reach_visits": 2,
"reach_points": 2
}
}
}
Example with customer’s address in the response
GET /customers/11e69610400894b2bb02089e01cf89b5?relationships=address HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Example: include tier progress and tier level
GET /customers/11e69610400894b2bb02089e01cf89b5?include=tier_level,tier_progress,tiers&relationships=tier_levels
Where:
included.tier_level
- the current tier for customer
included.tiers
- the list of tiers
included.tier_progress
- the tier progress for customer at the moment
Response Body
{
"data": {
"id": "11e69610400894b2bb02089e01cf89b5",
"email": "johndoe@example.com",
"phone": "5140000001",
"first_name": "John",
"last_name": "Doe"
...
},
"included": {
"tier_level": {
"id": 1,
"name": "VIP",
"reach_spend": 0,
"reach_visits": 0,
"reach_points": 10
},
"tiers": [
{
"id": 1,
"tiers_sequence": [
"2"
],
"tiers_relation": 0,
"reset_type": 2,
"reset_period_month": 8,
"base_previous_period": 0,
"enabled": true,
"tier_levels": [
{
"id": 1,
"name": "VIP",
"reach_spend": 0,
"reach_visits": 0,
"reach_points": 10
},
{
"id": 2,
"name": "Platinum",
"reach_spend": 0,
"reach_visits": 0,
"reach_points": 20
}
]
}
],
"tier_progress": {
"total_amount": null,
"total_points": 10,
"total_visits": null,
"left_amount": null,
"left_points": 10,
"left_visits": null,
"tiers_relation": 0,
"start_date": "2020-08-01T00:00:00+00:00",
"end_date": "2022-07-31T23:59:59+00:00",
"tier_level": "VIP",
"next_tier_level": "Platinum",
"tier_progress": 0
}
}
}
Example get the referral program and referral link for a customer
GET /customers/{customerId}?include=referral_programs
Response:
{
"data": {
"id": "11ec045006743d2b9d2eb42e99312ac8",
"email": "john@example.com",
"phone": null,
"first_name": "John",
"last_name": "Doe"
},
"included": {
"referral_programs": [
{
"id": 1109,
"rule_name": "BACKEND_REFERRAL_RULES_PURCHASE",
"cond_min_amount": 10,
"referee_earns": 0,
"referer_earns": 0,
"expires_in": 60,
"enabled": true,
"link_referral_enabled": true,
"referral_link": "https://krn.biz/15b9hZaM"
}
]
}
}
- id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
- include
enum
(optional) Example: balance,tierrelated resources to include in the response
Customer Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"first_name": "John",
"last_name": "Doe",
"email": "johndoe@example.com",
"birth_date": "1963-03-28",
"language": "en",
"gender": "male"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
},
"email": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"language": {
"type": "string",
"description": "Communication language. Possible values `en` - English, `fr` - French, `es` - Spanish"
},
"gender": {
"type": "string",
"description": "Customer's gender. Can be male or female"
}
},
"required": [
"email"
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"phone": "5141234567",
"birth_date": "1963-03-28",
"gender": "male",
"balance": {
"points": 135,
"giftcard": 21.35
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"first_name": {
"type": "string",
"description": "First name for the Customer."
},
"last_name": {
"type": "string",
"description": "Last name for the Customer."
},
"email": {
"type": "string"
},
"phone": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"gender": {
"type": "string",
"description": "Customer's gender. Can be male or female"
},
"balance": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"giftcard": {
"type": "number"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"description": "Bad Request",
"message": "Invalid ID supplied"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"description": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Customer not found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"email": [
"The email field is required"
],
"phone": [
"The phone field is required"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"email": {
"type": "array"
},
"phone": {
"type": "array"
}
}
}
Update a customerPUT/customers/{id}
Updates the details of an existing customer.
Body parameters
Name | Type | Description |
---|---|---|
string | Optional. The customer's email address. | |
phone | string | Optional. The customer's phone number. |
country_code | string | Optional. Required only if phone number supplied. The customer's country, in ISO 3166-1-alpha-2 format. |
first_name | string | Optional. First Name |
last_name | string | Optional. Last Name |
language | string | Optional. Communication language. Possible values en - English, fr - French, es - Spanish |
gender | string | Optional. Possible values male , female |
enabled | boolean | Optional. Possible values true , false |
Example
PUT /customers/12e69610400894b2b02089e01cf89b5 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSU...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{ "email" : "johndoe@example.com", "phone": "5140000001", "first_name": "John", "last_name": "Doe" }
Respone Body
{
"data": {
"id": "11e69610410894b2bb02089e01cf89b5",
"email": "johndoe@example.com",
"phone": "5140000001",
"first_name": "John",
"last_name": "Doe"
}
}
Activate or deactivate a customer
PUT /customers/12e69610400894b2b02089e01cf89b5
{
"enabled": true
}
PUT /customers/12e69610400894b2b02089e01cf89b5
{
"enabled": false
}
Update address
PUT /customers/12e69610400894b2b02089e01cf89b5
{
"address": {
"street": "9500 Madisson Avenue",
"city": "Austin",
"region": "Texas",
"country": "United States",
"country_code": "US",
"lat": 47.22,
"long": 33.33,
"zip_code": "78757"
}
}
- id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
Customer Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Delete a customerDELETE/customers/{id}
Deletes the customer with the requested id.
- id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
Customer Transactions ¶
Transaction ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 256559,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000",
"updated_at": "2017-03-06T19:45:47+0000"
},
{
"id": 256571,
"amount": 0,
"points": 50,
"name": "Redeem",
"created_at": "2017-03-06T19:45:47+0000",
"updated_at": "2017-03-06T19:45:47+0000"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 403,
"message": "This action is unauthorized"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Customer Not Found",
"description": "The requested resource doesn't exist"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
List all transactionsGET/customers/{customer_id}/transactions
Returns a list of transactions
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
relationships | enum | Optional. You can optionally request the response to include related resources |
include | string | Optional. You can optionally request that the response includes other resources. Specify include=ecom_coupon in your request. |
created_at | string | Optional. Filter by created date. See Filtering |
updated_at | string | Optional. Filter by updated date. See Filtering |
Examples
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk...
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1991625373,
"amount": 0,
"points": 100,
"name": "Redemption",
"created_at": "2017-10-06T15:55:06+00:00",
"utc_updated": "2017-10-06T15:55:06+00:00"
},
{
"id": 599112524,
"amount": 5,
"points": 50,
"name": "Reward",
"created_at": "2017-03-24T22:13:15+00:00",
"utc_updated": "2017-03-24T22:13:15+00:00"
}
...
],
"links": {
...
},
"meta": {
...
}
}
Relationships
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object for each transaction
- transaction_details - includes transaction details for each transaction, the transaction is not always having transaction_details.
Include
NOTE: You could also request other resource using include parameter. All possible values:
- ecom_coupon - includes ecom coupon object for each transaction
The value of the include or relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?relationships=branch,transaction_details HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1991625373,
"amount": 0,
"points": 100,
"name": "Redemption",
"created_at": "2017-10-06T15:55:06+00:00",
"utc_updated": "2017-10-06T15:55:06+00:00",
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Retrorant",
...
},
"transaction_details": null
}
]
}
Filtering
Supported Fields:
-
created_at
- Filter by created date -
updated_at
- Filter by updated date -
transaction_type
- Filter by transaction type
Example:
Filter by date
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?created_at=between|2020-10-01T23:15:25+00:00,2020-10-31T23:15:25+00:00
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?created_at=gte|2020-10-01T23:15:25+00:00
Filter by type
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?transaction_type=eq|4
- reward
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?transaction_type=eq|5
- redeem
Filter by branch
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/transactions?branch.id=eq|11eaa5c938ec36cc9df9b42e99312ac8
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer Offers ¶
Customer Offer ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Offer Title",
"points": "3000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
},
{
"id": "112",
"title": "Offer Title 2",
"points": "50000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all offersGET/customers/{customer_id}/offers
List all offers for a customer. This includes all types of offers: Points Multiplier, Free Product, Bonus Points, Regular/Basic offer (discount, free item, points) that a customer can choose from. Each offer might have a list of actions for that customer.
-
Basic Offer (coupon) -
type_id: 8,9,10
-
Points Multiplier -
type_id: 1
-
Free Product -
type_id: 2
-
Bonus Points -
type_id: 3
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include the branch object. To do so, specify relationships=branch in your request. |
coupon_type_id | mixed | Optional. This parameter filters offers based on their type, allowing users to specify the category of offers they want to view, such as pending, claimed, or convertible |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [...],
"actions": [
{
"type": "get_coupon"
}
]
}
]
}
Coupon Types
-
coupon_type_id: 74
- Regular coupon percentage, amount or free item - available for redemption -
coupon_type_id: 75
- Regular coupon percentage, amount or free item - redeemed -
coupon_type_id: 91
- Convertible coupon available for redemption -
coupon_type_id: 92
- Convertible coupon redeemed
Filtering by specific type can be done using the coupon_type_id
query string parameter:
/customers/{id}/offers
- Show all offers for a customer, including targeted, claimable, or previously claimed/redeemed.
coupon_type_id=eq|74
- Show only regular offers that are pending to claim/redeem.
coupon_type_id=in|74,91
- Show only regular offers and convertible coupons that are pending to claim/redeem.
coupon_type_id=eq|75
- Show only regular offers that were previously claimed/redeemed.
coupon_type_id=not_in|74,75,91,92
- Show all offers that are not pending to claim and not previously claimed.
Relationships
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object in the response
The value of the relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/offers?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [...],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
]
}
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer Available Coupons ¶
Customer Available Coupons ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 1000,
"qrcode": "66668496",
"coupon_locked": false,
"coupon_type_id": 74,
"expires_at": "2019-10-07T20:03:25+00:00",
"offer": {
"id": 111,
"title": "Offer Title",
"points": "3000",
"type": "discount_percentage",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
}
},
{
"id": 1001,
"qrcode": "62368496",
"coupon_locked": false,
"coupon_type_id": 91,
"expires_at": "2019-10-07T20:03:25+00:00",
"offer": {
"id": 112,
"title": "Offer Title 2",
"points": "50000",
"type": "discount_amount",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all Available CouponsGET/customers/{customer_id}/available-coupons
List all Available Coupons for a customer. Each coupon has a related offer
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/available-coupons HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123456,
"qrcode": "66668496",
"coupon_locked": false,
"coupon_type_id": 74,
"expires_at": "2019-10-07T20:03:25+00:00",
"offer": {
"id": 1234,
"points": 0,
"expires_at": "2028-10-01T03:59:59+0000",
"discount_value": 10,
"multip_factor": 0,
"real_value": 0,
"type": "discount_percentage",
"type_id": 9,
"targeted_offer_flag": 1,
"title": "Coupon 10% OFF",
"description": "",
"images": [...]
}
}
]
}
Coupon Types
-
coupon_type_id: 74 - Regular coupon percentage, amount or free item - available for redemption
-
coupon_type_id: 75 - Regular coupon percentage, amount or free item - redeemed
-
coupon_type_id: 91 - Convertible coupon available for redemption
-
coupon_type_id: 92 - Convertible coupon redeemed
NOTE: Only coupon type ID 74 can be claimed A coupon MUST be assigned to customer before it will be available for redemption In order to assign a coupon to a customer create a transaction with intent:
get_coupon
, customer and offer object
Example assign a coupon to customer
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "get_coupon",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"offer": {
"id": 1234
}
}
Response Body
{
"data": {
"id": 1892267,
"amount": 0,
"points": 0,
"name": "Coupon Tagged",
"created_at": "2019-10-04T16:07:33+00:00",
"updated_at": "2019-10-04T16:07:33+00:00",
"offers": [...],
"coupon": {
"id": 512572,
"qrcode": "12428967",
"coupon_locked": false,
"coupon_type_id": 74,
"expires_at": "2019-10-14T16:07:33+00:00",
"offer": {...}
}
}
}
Example claim a coupon (redeem)
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "claim_coupon",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"offer": {
"id": 1234
},
"coupon": {
"id": 512572
}
}
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer Actions ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"type": "recalculate",
"resource": "tier_level"
}
Headers
Content-Type: application/json
Body
{
"data": {
"tier_progress": {
"total_amount": 0,
"total_points": 100,
"tier_level": "VIP"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"tier_progress": {
"type": "object",
"properties": {
"total_amount": {
"type": "number"
},
"total_points": {
"type": "number"
},
"tier_level": {
"type": "string"
}
}
}
}
}
}
}
POST/customers/{id}/actions
Trigger an action for a customer
Body parameters
Name | Type | Description |
---|---|---|
resource | string | Required One of the following: tier_level , reward |
type | string | Required One of the following: recalculate , calculate , batch_calculate |
intent | string | Required with resource=reward One of the following: reward , reward_store_credit |
amount | number | Optional Used only with resource=reward. |
points | integer | Optional Used only with resource=reward. |
visits | integer | Optional Used only with resource=reward. |
branch.id | string | Optional Used only with resource=reward. |
product_rewards | array | Optional Used only with resource=reward. |
product_rewards.id | integer | Optional Used only with resource=reward. |
product_rewards.quantity | integer | Optional Used only with resource=reward. |
items | array | Optional Used only with resource=reward and type=batch_calculate |
items.amount | number | Optional Used only with resource=reward and type=batch_calculate. |
items.points | integer | Optional Used only with resource=reward and type=batch_calculate. |
items.visits | integer | Optional Used only with resource=reward and type=batch_calculate. |
items.branch.id | string | Optional Used only with resource=reward and type=batch_calculate. |
items.product_rewards | array | Optional Used only with resource=reward and type=batch_calculate. |
items.product_rewards.id | integer | Optional Used only with resource=reward and type=batch_calculate. |
items.product_rewards.quantity | integer | Optional Used only with resource=reward and type=batch_calculate. |
Note: One of the following attributes is required
amount
,points
orvisits
when intent is:reward
Note: If an offer type points multiplier is available and all the conditions are met:
- Must be available at the specified branch
- The amount spent is between minimum and maximum purchase amount configured for the offer
- Offer frequency
the points will be calculated and returned in the response for offer_reward.points attribute
Note: The branch.id is optional and will be used only to calculate the points if an offer is available at the specific branch.
Default: main branch if no branch ID is passed
Example: recalculate tier level
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"type": "recalculate",
"resource": "tier_level"
}
Response Body
{
"data": {
"tier_progress": {
"total_amount": null,
"total_points": null,
"total_visits": 10,
"left_amount": null,
"left_points": null,
"left_visits": 5,
"tiers_relation": 0,
"start_date": "2021-01-01T00:00:00+00:00",
"end_date": "2022-12-31T23:59:59+00:00",
"tier_level": "VIP",
"next_tier_level": "Platinum",
"tier_progress": 1
}
}
}
Example: Calculate points the customer will earn based on amount spent. An offer might be applied if available at the main branch
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"type": "calculate",
"resource": "reward",
"intent": "reward",
"amount": 20
}
Example: Calculate points the customer will earn based on amount spent and product rewards
Response Body
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions
{
"type": "calculate",
"resource": "reward",
"intent": "reward",
"amount": 20,
"product_rewards": [
{
"id": 123,
"quantity": 1
}
]
}
Example: Calculate points the customer will earn based on amount spent and offer applied if available at the specific branch
Response Body
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions
{
"type": "calculate",
"resource": "reward",
"intent": "reward",
"amount": 20,
"branch": {
"id":"11eac6c914dfbc4e8478b42e99312ac8"
}
}
Response:
{
"data": {
"basic_reward": {
"points": 30,
"amount_spent": 30,
"amount_original": 30
},
"product_reward": {
"points": 0
},
"offer_reward": {
"points": 10
},
"total_points": 40
}
}
Example: Calculate store credit the customer would earn
Response Body
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions
{
"type": "calculate",
"resource": "reward",
"intent": "reward_store_credit",
"amount": 20
}
Example: Batch calculate points the customer will earn based on amount spent at the specific branch
Response Body
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/actions
{
"resource": "reward",
"type": "batch_calculate",
"intent": "reward",
"items": [
{
"branch": {
"id": "11eac6c914dfbc4e8478b42e99312ac8"
},
"product_rewards": [
{
"id":296,
"quantity":2
}
],
"amount": 20
}
]
}
Response:
{
"data": [
{
"basic_reward": {
"points": 20,
"amount_spent": 20,
"amount_original": 20
},
"product_reward": {
"points": 0
},
"offer_reward": {
"points": 0
},
"total_points": 20
}
]
}
- id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
Claimable Offers ¶
Claimable Offer ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Offer Title",
"points": "3000",
"priority": 1,
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
},
{
"id": "112",
"title": "Offer Title 2",
"points": "50000",
"priority": 1,
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all claimable offersGET/customers/{customer_id}/claimable-offers?amount={amount}&branch_id={branch_id}&page={page}&per_page={per_page}
Retrieves all claimable offers for a customer, sorted by priority.
An offer is considered claimable if the following conditions are met:
-
Amount
: The purchase amount will be compared against the values specified in the Min Purchase and Max Purchase fields for the offer. -
Branch ID
: The offer must be available at the branch where the customer is shopping. -
Frequency
: The frequency at which the offer can be claimed. This may include various conditions, such as once or on specific days and times. -
Previous Claims
: The customer must not have previously claimed the same offer.
The first eligible offer will apply to the next purchase if all the above conditions are satisfied.
This information helps customers understand which offer will be applied based on various criteria. If multiple offers are available, only one will be applied to the purchase.
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
amount | numeric | Required. The purchase amount |
branch_id | string | Required. The branch ID where the offers are available |
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/claimable-offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"priority": 1,
"multip_factor": 2,
"real_value": 0,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [...]
}
]
}
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
- page
number
(optional) Default: 1 Example: 1The current page.
- per_page
number
(optional) Default: 15 Example: 15The maximum number of results to return.
- amount
number
(required) Example: 3.99The purchase amount
- branch_id
string
(required) Example: e89eb1e9708The branch ID where the offers are available
Customer Rewards ¶
Customer Rewards ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Reward Title",
"points": "3000",
"amount": 10,
"type": "redeem_discount_amount",
"description": "Some Reward Description",
"expires_at": "2027-06-27T02:59:59+00:00"
},
{
"id": "112",
"title": "Reward Title 2",
"points": "50000",
"amount": 20,
"type": "redeem_discount_amount",
"description": "Some Reward Description",
"expires_at": "2027-04-27T02:59:59+00:00"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all rewards for a customerGET/customers/{customer_id}/rewards
List all rewards for a customer.
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
branch.id | string | Optional. The branch ID where the rewards are available. See Filtering |
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123,
"points": 1000,
"publish_at": "2020-06-03T18:41:34+00:00",
"expires_at": "2030-06-04T03:59:59+00:00",
"amount": 10,
"real_value": 0,
"discount_value": 0,
"partner_reward": false,
"redeem_for_gift_card": false,
"type": "redeem_discount_amount",
"title": "10$ Discount",
"description": "",
"images": [...]
}
]
}
Filtering
When submitting a GET request, you can ask it to return only rewards that meet specific criteria.
To filter rewards, in the query string, specify the field with an operator, followed by a separator (|
)
and the values for the following:
Fields:
branch.id
- Filter by branch id, the operator is “eq” or “in”
Operators:
-
in
- Match any value in the list. Separated by comma. -
eq
- Equal
Filter Syntax
A single filter uses the form: field=operator|VALUE
Each URL query parameter must be URL encoded.
Example: Retrieve all rewards that are available in a specific branch
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/rewards?branch.id=eq%7C11ec423d0046912d9800e2865c66d9cc
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer Consents ¶
Customer Consent Collection ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"allow_email": true,
"allow_sms": false,
"allow_push": true
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Retrieve consent recordsGET/customers/{customer_id}/consents
List all consent records for a customer
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer Consent Collection ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"allow_email": true,
"allow_sms": false,
"allow_push": true
}
Headers
Content-Type: application/json
Body
{
"data": {
"allow_email": true,
"allow_sms": false,
"allow_push": true
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"allow_email": {
"type": "boolean"
},
"allow_sms": {
"type": "boolean"
},
"allow_push": {
"type": "boolean"
}
}
}
}
}
Create a consentPOST/customers/{customer_id}/consents
Create a consent record for a customer
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Body parameters
Name | Type | Description |
---|---|---|
allow_sms | boolean | Required true or false |
allow_email | boolean | Required true or false |
allow_push | boolean | Required true or false |
NOTE: In order to update the consent for a customer a new consent must be created
Example:
POST /customers/11eb5b4433edf7bfaaffb42e99312ac8/consents HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"allow_sms": false,
"allow_email": true,
"allow_push": true
}
Response Body
{
"data": {
"id": 123,
"allow_sms": false,
"allow_email": true,
"allow_push": true
}
}
- customer_id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
Customer CRM fields ¶
Customer CRM fields Collection ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
[
{
"name": "first_name",
"label": "First Name",
"value": "John",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": false,
"display_order": 1
},
{
"name": "custom_field_1",
"label": "Custom Field 1",
"value": "test",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": true,
"display_order": 2
}
]
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Retrieve CRM fieldsGET/customers/{customer_id}/crm-fields
List all CRM fields for a customer
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Example:
GET /customers/11eb5b4433edf7bfaaffb42e99312ac8/crm-fields
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"name": "custom_field_1",
"label": "Custom Field 1",
"value": "Custom field value 1",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": true,
"display_order": 1
},
{
"name": "custom_field_2",
"label": "Custom Field 2",
"value": "Custom field value 2",
"is_hidden": false,
"is_required": false,
"field_type": "text",
"is_custom_field": true,
"display_order": 2
}
]
}
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Customer CRM fields Collection ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"first_name": "John",
"last_name": "Doe",
"email": "john@example.com",
"gender": "male",
"birth_date": "1999-01-26",
"promotions_mail": false,
"notes": "Some notes",
"tags": [
{
"tag_name": "High Spender"
},
{
"tag_name": "Social Media Follower"
}
],
"custom_field_1": "Custom field value 1",
"custom_field_2": "Custom field value 2"
}
Headers
Content-Type: application/json
Body
{
"data": [
{
"name": "first_name",
"label": "First Name",
"value": "John",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": false,
"display_order": 1
},
{
"name": "custom_field_1",
"label": "Custom Field 1",
"value": "test",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": true,
"display_order": 2
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Update Customer CRM fields valuesPATCH/customers/{customer_id}/crm-fields-values
Update CRM fields values for a customer
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Body parameters
Name | Type | Description |
---|---|---|
first_name | string | Required or Optional |
last_name | string | Required or Optional |
string | Required or Optional |
|
phone | string | Required or Optional |
country_code | string | Required or Optional |
gender | string | Required or Optional - male, female, nonbinary |
birth_date | string | Required or Optional |
postal_code | string | Required or Optional |
promotions_mail | string | Required or Optional |
notes | string | Required or Optional |
tags | array | Required or Optional |
tags.tag_name | string | Required or Optional |
custom_field_1 | string | Required or Optional |
custom_field_2 | string | Required or Optional |
custom_field_3 | string | Required or Optional |
custom_field_4 | string | Required or Optional |
custom_field_5 | string | Required or Optional |
Example:
PATCH /customers/11eb5b4433edf7bfaaffb42e99312ac8/crm-fields-values
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"first_name": "John",
"last_name": "Doe",
"email": "john@example.com",
"phone": "",
"country_code": "CA",
"gender": "male",
"birth_date": "1999-01-26",
"postal_code" : "123456",
"promotions_mail" : false,
"notes": "Some notes",
"tags": [
{
"tag_name": "High Spender"
},
{
"tag_name": "Social Media Follower"
}
],
"custom_field_1": "Custom field value 1",
"custom_field_2": "Custom field value 2",
"custom_field_3": "Custom field value 3",
"custom_field_4": "Custom field value 4",
"custom_field_5": "Custom field value 5"
}
Response Body
{
"data": [
{
"name": "custom_field_1",
"label": "Custom Field 1",
"value": "Custom field value 1",
"is_hidden": false,
"is_required": true,
"field_type": "text",
"is_custom_field": true,
"display_order": 1
},
{
"name": "custom_field_2",
"label": "Custom Field 2",
"value": "Custom field value 2",
"is_hidden": false,
"is_required": false,
"field_type": "text",
"is_custom_field": true,
"display_order": 2
}
]
}
- customer_id
string
(required) Example: 11e696103f8ff5eabb02089e01cf89b5The unique ID of the customer.
Customer Targeted Offers ¶
Customer Offer ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Offer Title",
"points": "3000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
},
{
"id": "112",
"title": "Offer Title 2",
"points": "50000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all Targeted offersGET/customers/{customer_id}/targeted-offers
List all available offers for a customer.
Path Parameters
Name | Type | Description |
---|---|---|
customer_id | string | Required. The ID of the customer |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include the branch object. To do so, specify relationships=branch in your request. |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
branch.id | string | Optional. The branch ID where the rewards are available. See Filtering |
type_id | integer | Optional. Offer Type. Possible options: 1 - Points Multiplier, 2 - Free Product, 3 - Bonus Points, 8 - Basic Offer (Free product), 9 - Basic Offer (percent-off), 10 - Basic Offer (amount-off). See Filtering |
include | string | Optional. You can optionally request that the response includes other resources. Specify include=coupon in your request. |
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/targeted-offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [...],
}
]
}
Relationships
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object in the response
The value of the include or relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/offers?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [...],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
]
}
Filtering
When submitting a GET request, you can ask it to return only offers that meet specific criteria.
To filter offers, in the query string, specify the field with an operator, followed by a separator (|
)
and the values for the following:
Fields:
-
branch.id
- Filter by branch id, the operator is “eq” or “in” -
type_id
- Filter by offer type, the operator is “eq” or “in”
Operators:
-
in
- Match any value in the list. Separated by comma. -
eq
- Equal
Filter Syntax
A single filter uses the form: field=operator|VALUE
Each URL query parameter must be URL encoded.
Example Retrieve all targeted offers that are available for the ecom branch
GET /customers/12e6a34f8e89eb1e9708089e01cf89b5/targeted-offers?include=coupon&branch.id=eq%7C11ec423d0046912d9800e2865c66d9cc&type_id=in%7C8,9,10,1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data":
[
{
"id": 583,
"points": 0,
"expires_at": "2038-01-18T19:00:00-05:00",
"publish_at": "2021-08-12T00:00:00-04:00",
"is_published": true,
"offer_languages":
[
{
"id": 624,
"language_id": 1,
"offer_title": "*2",
"offer_link": "",
"offer_description": "",
"offer_terms_conditions": "",
"language":
{
"id": 1,
"abbreviation": "en",
"name": "English"
}
}
],
"coupon":
[]
},
{
"id": 1614,
"points": 0,
"expires_at": "2038-01-18T19:00:00-05:00",
"publish_at": "2022-10-05T00:00:00-04:00",
"is_published": true,
"coupon_convertible": false,
"offer_languages":
[
{
"id": 1722,
"language_id": 1,
"offer_title": "20% OFF",
"offer_link": "",
"offer_description": "",
"offer_terms_conditions": "",
"language":
{
"id": 1,
"abbreviation": "en",
"name": "English"
}
}
],
"coupon":
{
"id": 12176,
"qrcode": "48390008",
"coupon_locked": false,
"coupon_redeemed": false,
"coupon_type_id": 74,
"expires_at": "2038-01-19T00:00:00+00:00",
"coupon_claimed_at": "2023-03-31T17:30:56+00:00"
}
}
],
"links":
{
"first": "http://api.kangaroorewards.com/customers/11edb21c343c19d692bfe2865c66d9cc/targeted-offers?page=1",
"last": null,
"prev": null,
"next": null
},
"meta":
{
"current_page": 1,
"from": 1,
"path": "http://api.kangaroorewards.com/customers/11edb21c343c19d692bfe2865c66d9cc/targeted-offers",
"per_page": 15,
"to": 2
}
}
- customer_id
string
(required) Example: 12e6a34f8e89eb1e9708089e01cf89b5ID of the customer
Transactions ¶
Transaction ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 256559,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000",
"updated_at": "2017-03-06T19:45:47+0000"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all transactionsGET/transactions
Returns a list of transactions
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
relationships | enum | Optional. You can optionally request that the response include the customer object. To do so, specify relationships=customer in your request. |
created_at | string | Optional. Filter by created date. See Filtering |
updated_at | string | Optional. Filter by updated date. See Filtering |
transaction_details.external_order_id | string | Optional. Filter by external order ID. See Filtering |
Examples
GET /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1991625373,
"amount": 0,
"points": 100,
"name": "Redemption",
"created_at": "2017-10-06T15:55:06+00:00",
"updated_at": "2017-10-06T15:55:06+00:00"
},
{
"id": 599112524,
"amount": 5,
"points": 50,
"name": "Reward",
"created_at": "2017-03-24T22:13:15+00:00",
"updated_at": "2017-03-24T22:13:15+00:00"
}
...
],
"cursor": {
"count": 30,
"next": 2,
"next_uri": "transactions?page=2"
},
"meta": {
"total": 30
}
}
Relationships
NOTE: You could also request more information using relationship parameter. All possible values:
- customer - includes customer object in the response
- purchase.receipt - includes receipt related to transaction
- transaction_sequences - includes related transactions
- transaction_details - includes transaction details related to transaction
The value of the relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /transactions?relationships=customer HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1991625373,
"amount": 0,
"points": 100,
"name": "Redemption",
"created_at": "2017-10-06T15:55:06+00:00",
"updated_at": "2017-10-06T15:55:06+00:00",
"customer": {
"id": "11e7aa159d83fae9bf8d000c296d46ef",
"email": "john@example.com",
"phone": null,
"first_name": "John",
"last_name": "Doe",
"created_at": "2017-10-05T21:39:07+00:00",
"updated_at": "2017-10-05T21:39:09+00:00"
}
},
...
],
"cursor": {
"count": 30,
"next": 2,
"next_uri": "transactions?page=2"
},
"meta": {
"total": 30
}
}
Example with transaction_sequences
GET /transactions?relationships=transaction_sequences HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
{
"id": 1892256,
"amount": 0.1,
"points": 0,
"name": "Store Credit",
"created_at": "2020-01-16T21:53:01+00:00",
"updated_at": "2020-01-16T21:53:01+00:00",
"transaction_sequences": [
{
"id": 26112,
"initial_transaction_id": 1892256,
"next_transaction_id": 1892257,
"next_transaction": {
"id": 1892257,
"amount": 10,
"points": 10,
"name": "Reward",
"created_at": "2020-01-16T21:53:01+00:00",
"updated_at": "2020-01-16T21:53:01+00:00"
}
}
]
},
Filtering
When submitting a GET request, you can ask it to return only transactions that meet specific criteria.
To filter transactions, in the query string, specify one or more fields with an operator, followed by a separator (|
)
and the values for the following:
Fields:
-
created_at
- Filter by created date -
updated_at
- Filter by updated date -
transaction_details.external_order_id
- Filter by external order ID -
customer.id
- Filter by customer id, the operator is “eq” or “in”
Operators:
-
gt
- Greater Than -
gte
- Greater Than or Equal -
lt
- Less Than -
lte
- Less Than or Equal -
in
- Match any value in the list. Separated by comma. -
between
- Match any value in a range. Separated by comma. -
eq
- Equal
Filter Syntax
A single filter uses the form: field=operator|VALUE
Each URL query parameter must be URL encoded.
Example: Retrieve all transactions that have created date greather than 2017-01-27T14:37:16+0000
GET /transactions?created_at=gt%7C2017-01-27T14%3A37%3A16%2B0000&transaction_details.external_order_id=in%7CORD0001,ORD0012
Transaction Types
name | id |
Offer Claimed | 2 |
Reward | 4 |
Redemption | 5 |
Offer Shared on FB | 15 |
Offer Shared on TW | 16 |
Signup Bonus | 33 |
Offer Shared on FB | 37 |
Offer Shared on TW | 38 |
Offer Shared on FB | 41 |
Offer Shared on TW | 42 |
Redemption - Catalog | 43 |
Transaction Canceled - Clerk | 44 |
Offer Canceled - Clerk | 45 |
Giftcard Shared on FB | 52 |
Giftcard Shared on TW | 53 |
Giftcard Purchased - Points | 55 |
Giftcard Refunded - PayPal | 56 |
Points Transfer - Sender | 58 |
Points Transfer - Receiver | 59 |
Redemption on behalf of Client - Custom | 62 |
Redemption on behalf of Client - Catalog | 63 |
Refund - Points | 64 |
AutoClaim - Points | 67 |
MultiAutoClaim - Points | 68 |
Follow-My-Store Bonus | 69 |
Giftcard Purchase - At Store | 70 |
Coupon Tagged | 74 |
Coupon claimed | 75 |
Referred rewarded | 76 |
Referrer rewarded | 77 |
Adjust Increase Points | 80 |
Adjust Decrease Points | 82 |
Giftcard Purchased - Amount | 84 |
Giftcard Purchase - At Store | 85 |
Amount Transfer - Sender | 86 |
Amount Transfer - Receiver | 87 |
Giftcard Payment | 88 |
Convertible Coupon created | 91 |
Convertible Coupon redeemed | 92 |
Cash-back | 102 |
Refund Cash-back | 103 |
Refund - Redeemed Points | 107 |
Transaction ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json
Content-Type: application/json
Body
{
"intent": "reward",
"amount": 5.25,
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "string"
},
"amount": {
"type": "number"
},
"customer": {
"type": "object",
"properties": {
"id": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 256559,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000",
"updated_at": "2017-03-06T19:45:47+0000"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"amount": {
"type": "number"
},
"points": {
"type": "number"
},
"name": {
"type": "string"
},
"created_at": {
"type": "string"
},
"updated_at": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Invalid Employee ID",
"link": "https://api.kangaroorewards.local/docs"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
},
"link": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Not found",
"description": "Referral not found for customer"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"customer.id": [
"The selected customer.id is invalid."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"customer.id": {
"type": "array"
}
}
}
Headers
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json
Content-Type: application/json
Body
{
"intent": "reward",
"amount": 5.25,
"customer": {
"email": "john@example.com"
},
"product_rewards": [
{
"id": 123,
"quantity": 3
},
{
"id": 124,
"quantity": 1
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "string"
},
"amount": {
"type": "number"
},
"customer": {
"type": "object",
"properties": {
"email": {
"type": "string"
}
}
},
"product_rewards": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 256559,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000",
"customer": {
"id": "11e6fd0b9882cd0d84a5089e01cf89b5",
"email": "john@example.com",
"balance": {
"points": 259,
"amount": 2.59
}
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"amount": {
"type": "number"
},
"points": {
"type": "number"
},
"name": {
"type": "string"
},
"created_at": {
"type": "string"
},
"customer": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"email": {
"type": "string"
},
"balance": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"amount": {
"type": "number"
}
}
}
}
}
}
}
}
}
Create a transactionPOST/transactions
A Transaction is a single event where a customer earns or spends points.
You can create a transaction in one of two ways: by Customer ID or customer’s email address/phone number. If that customer exists, we’ll simply add that Transaction for that customer. If the customer doesn’t exist, we’ll create a new customer, award the Transaction, and then send the customer a welcome email/SMS.
In addition, you can retrieve a specific transaction or a list of transactions.
Use /transactions
resource to create a reward, redeem or return transaction.
A reward
transaction will increase the balance for the customer based on the amount included in the JSON request body.
To create a reward transaction, set the amount, points, or visits. Use visits
when “Claim Points” option is active.
Include product_rewards
object when you want to apply “À La Carte Rewards” (Product Rewards) for reward
transaction only.
The total points earned is calculated based on the current Earning Rule value configured in Business Portal. If there are offers to apply, ex. Points Multiplier than it will automatically multiply the points and update the customer’s balance.
A redeem
transaction will decrease the balance for the customer based on the amount included.
To create a redeem transaction, set the amount or points.
Include catalog_items
object when you want to redeem a “Redemption Catalog” item for redeem
transaction only.
The customer
object is required for all transaction types, but only id, email or phone field is required.
The employee.pin_code
is required only when redeeming on behalf of the customer or when the rule: "Employee must enter his ID when Purchase Amount exceeds …" is active
Body parameters
Name | Type | Description |
---|---|---|
intent | string | Set the intent to: reward , redeem , return , get_coupon , claim_coupon , purchase_giftcard_prepaid , giftcard_payment , adjust_points_balance , redeem_ecom_coupon , claim_ecom_coupon , cancel_ecom_coupon |
amount | number | Required if none of points , visits are present |
points | number | Required if none of amount , visits are present |
visits | number | Required if none of amount , points are present |
subtotal | number | Required if intent is redeem_ecom_coupon and catalog_item is not present. |
catalog_item | object | Required if intent is redeem_ecom_coupon and amount is not present. |
catalog_item.id | string | Required if catalog_item is present |
customer | object | Required. The customer object and at least id , email or phone must be provided |
customer.id | string | Required if none of customer.email , customer.phone are present |
customer.email | string | Required if none of customer.id , customer.phone are present |
customer.phone | string | Required if none of customer.id , customer.email are present |
customer.country_code | string | Required when customer.phone is present |
employee | object | Optional. The employee object that will be attached to the transaction |
employee.id | string | Optional. If provided, the Employee will be attached to the transaction |
employee.pin_code | number | Optional. Required only if the rule: "Employee must enter his ID when Purchase Amount exceeds …" is active. It will override the employee.id |
branch | object | Optional. To set the physical location where the transaction took place. By default it will be the main branch |
branch.id | string | Optional. The branch ID where the transaction took place |
catalog_items | object | Optional. The Catalog Item to be redeemed |
catalog_items.id | number | Required with Catalog Item. The Catalog Item ID |
product_rewards | object | Optional. The Product Rewards |
product_rewards.id | number | Required with product_reward. The Product Reward ID |
product_rewards.quantity | number | Required with product_reward. Quantity |
receipt | object | Optional. The receipt object that will be attached to the transaction |
receipt.number | string | Optional. If provided, the receipt number will be attached to the transaction |
offer | object | Optional. Required when intent is get_coupon or claim_coupon . The Offer to assign a coupon or when redeeming a coupon |
offer.id | number | Required with Offer. The Offer ID |
coupon | object | Optional. Required when intent is claim_coupon . The coupon to be redeemed (claimed) |
coupon.id | number | Required with coupon. The Coupon ID |
transaction_details | object | Optional. The transaction detail object that will be attached to the transaction |
transaction_details.external_order_id | string | Optional. The external order ID |
transaction_details.custom_field_1 | string | Optional. max:255, min:1 |
transaction_details.custom_field_2 | string | Optional. max:255, min:1 |
transaction_details.custom_field_3 | string | Optional. max:255, min:1 |
transaction_details.custom_field_4 | string | Optional. max:255, min:1 |
transaction_details.custom_field_5 | string | Optional. max:255, min:1 |
Note: Transaction intent is required and must be one of the following:
reward
- Reward points or amount. When using amount, it will be converted to points based on the reward rulesredeem
- Redeem points, amount or catalog itemreturn
- Return pointsget_coupon
- When the customers wants to get a couponclaim_coupon
- When the customer wants to claim (redeem) a couponpurchase_giftcard_prepaid
- Purchase a gift card in store. It will create a “Giftcard Purchase - At Store” transaction and gift card balance will be increasedgiftcard_payment
- Pay with gift card balance. It will create a “Giftcard Payment” transaction and the gift card balance will be depletedreward_store_credit
- Award Store Credit to customers. It will increase gift card balanceadjust_points_balance
- Adjust points balance. Points value can be positive (increase balance) or negative (decrease balance)reward_referral
- Reward referee and referrer based referral program conditionsredeem_ecom_coupon
- Redeem points, amount or catalog item and generate ecom coupon code (The business must have ecom integration connected to the Kangaroo Rewards, currently only available on Shopify integration)claim_ecom_coupon
- When the customer wants to redeem an offer and generate ecom coupon code (The business must have ecom integration connected to the Kangaroo Rewards, currently only available on Shopify integration)cancel_ecom_coupon
- When the customer wants to cancel the redeemed ecom coupon (The business must have ecom integration connected to the Kangaroo Rewards, currently only available on Shopify integration)
Note: One of the following attributes is required
amount
,points
orvisits
when intent is one of the following:reward
,redeem
,return
Note: To apply visits aggregation set
visits
value greater than 1. The Employee PIN code is also required.
Note: Customer object is required and at least
id
,phone
is required, country_code is required when phone number is used.
Note: Branch id is required and an ecom_coupon object will be returned in the response when intent is one of the following:
redeem_ecom_coupon
,claim_ecom_coupon
,cancel_ecom_coupon
. The branch must be connected to an ecom integration.
Example reward amount and a customer with ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"amount": 5.25,
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
}
}
Response Body
{
"data": {
"id": 256560,
"created_at": "2017-03-06T20:19:24+0000",
"amount": 5.25,
"points": 5,
"name": "Reward"
}
}
Example reward points and a customer with email address
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"points": 100,
"customer": {
"email": "john@example.com"
}
}
Note: When
employee.pin_code
is supplied, the Employee that has that Pin Code will override the Employee attached to the transaction
Example with Employee PIN Code required and Product Rewards
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"amount": 3,
"customer": {
"phone": "6043345025"
},
"employee":{
"pin_code": "3333"
},
"product_rewards": [
{
"id": 123,
"quantity": 3
},
{
"id": 124,
"quantity": 1
}
]
}
Response Body with relations
{
"data": {
"id": 256775,
"created_at": "2017-03-08T21:15:54+0000",
"amount": 3,
"points": 3,
"name": "Reward",
"offers": [
{
"id": 558,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 25,
"type": "free_product",
"title": "Free product",
"description": "Free product"
}
],
"customer": {
"id": "11e6fd0b9882cd0d84a5089e01cf89b5",
"email": "",
"phone": "6043345025",
"first_name": "John",
"last_name": "Doe",
"member_since": "2017-03-08T21:15:54+0000",
"balance": {
"points": 259,
"amount": 2.59
}
}
}
}
Note: You could pass the
branch.id
- wich will define where the transaction took place.
Example with Branch ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"amount": 3,
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"branch":{
"id": "11e7038ade3780aca64e089e01cf89b5"
}
}
Note: To attach an Employee to the transaction, you must set the
employee.id
in the payload
Example with Employee ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"amount": 3,
"customer": {
"id": "31e692103f8ef5eabd02089e01cf89b4"
},
"branch":{
"id": "11e696101aab59a4bb02089e01cf89b5"
},
"employee":{
"id": "21e3038a5e3780aca24e089e01cf84b2"
}
}
Example with Receipt Number
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"points": 100,
"customer": {
"id": "11e8bb6776ea7699b48e089e01cf89b5"
},
"receipt": {
"number": "12345678"
}
}
Example Redeem a Catalog Item
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "redeem",
"customer": {
"id": "11e6fd0b9882cd0d84a5089e01cf89b5",
"pin_code": 1111
},
"catalog_items": [
{
"id": 123,
"quantity": 1
}
]
}
Example redeem with Employee’s PIN Code
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "redeem",
"points": 10,
"customer": {
"id": "11e6fd0b9882cd1d84a5089e01cf89b"
},
"employee":{
"pin_code": "1111"
}
}
Example Return Amount
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "return",
"amount": 2.25,
"customer": {
"id": "11e6fd0b9882cd0d84a5089e01cf89b5",
"pin_code": 1111
}
}
Example assign a coupon to customer (Get coupon)
Note: Only offers with type_id 8, 9, 10 are coupons and can be assigned to a customer
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "get_coupon",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"offer": {
"id": 1234
}
}
Response Body
{
"data": {
"id": 1892267,
"amount": 0,
"points": 0,
"name": "Coupon Tagged",
"created_at": "2019-10-04T16:07:33+00:00",
"updated_at": "2019-10-04T16:07:33+00:00",
"offers": [...],
"coupon": {
"id": 512572,
"qrcode": "12428967",
"coupon_locked": false,
"coupon_type_id": 74,
"expires_at": "2019-10-14T16:07:33+00:00",
"offer": {...}
}
}
}
Example claim a coupon (redeem)
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "claim_coupon",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"offer": {
"id": 1234
},
"coupon": {
"id": 512572
}
}
Example purchase gift card prepaid (In store)
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "purchase_giftcard_prepaid",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"amount": 10
}
Example purchase gift card prepaid (In store)
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "purchase_giftcard_prepaid",
"customer": {
"email": "john@example.com"
},
"amount": 10
}
Example Pay with gift card balance
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "giftcard_payment",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"amount": 10
}
Example award Store Credit to customer
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward_store_credit",
"customer": {
"id": "11e8bb6776ea7699b48e089e01cf89b5"
},
"amount": 100
}
Example: Adjust(increase) points balance and a customer with ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "adjust_points_balance",
"points": 100,
"comments": "Add optional comments",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
}
}
Example: Adjust(decrease) points balance and a customer with ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "adjust_points_balance",
"points": -50,
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
}
}
Example: Reward referral request
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward_referral",
"amount": 40.99,
"customer": {
"id": "11eaa697ae8f46bd9df9b42e99312ac8"
}
}
Note: The response might return status 202 without a created transaction resource When reponse status code is 202 there won’t be a data object with a transaction resource because the referral condition not met
It might also return a 404 error if the referral record not found for customer or no active referral program exists
Example: Refund redeemed points
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "refund_redeemed_points",
"points": 100,
"customer": {
"id": "11eab58b792394f48b7fb42e99312ac8"
}
}
Note: This transaction will increase the points balance Also, it will not affect the tier level
Example with External order ID
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "reward",
"points": 100,
"customer": {
"id": "11e8bb6776ea7699b48e089e01cf89b5"
},
"transaction_details": {
"external_order_id": "ORD0001",
"custom_field_1": "34-28599475251",
"custom_field_2": null,
"custom_field_3": null,
"custom_field_4": null,
"custom_field_5": null
}
}
Example redeem amount and generate an ecom coupon
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "redeem_ecom_coupon",
"amount": 5.25,
"subtotal": 25.69,
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5"
}
}
Example redeem a catalog item and generate an ecom coupon
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "redeem_ecom_coupon",
"catalog_item": {
"id": "490"
},
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5"
}
}
Example claim an offer and generate an ecom coupon
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "claim_ecom_coupon",
"offer": {
"id": 1614
},
"coupon": {
"id": 11553
},
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5"
}
}
Example cancel an ecom coupon
POST /transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"intent": "cancel_ecom_coupon",
"code": "dpeLsnS",
"customer": {
"id": "11e696103f8ff5eabb02089e01cf89b5"
},
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5"
}
}
Transaction Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Invalid Employee ID",
"link": "https://api.kangaroorewards.local/docs"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
},
"link": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Cancel a transactionDELETE/transactions/{id}
You can cancel a transaction any time. When canceling a transaction, the points will be depleted from the customer’s balance When cancelling a points multiplier transaction, the parent transaction will also be cancelled
NOTE: Only transaction type_id: 2, 4 can be cancelled
The customer must have enough points balance
- id
number
(required) Example: 123The unique ID of the transaction.
Transaction Details ¶
Transaction Details Resource ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": {
"external_order_id": "Hello, world!",
"custom_field_1": "Hello, world!",
"custom_field_2": "Hello, world!",
"custom_field_3": "Hello, world!",
"custom_field_4": "Hello, world!",
"custom_field_5": "Hello, world!"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"external_order_id": {
"type": "string"
},
"custom_field_1": {
"type": "string"
},
"custom_field_2": {
"type": "string"
},
"custom_field_3": {
"type": "string"
},
"custom_field_4": {
"type": "string"
},
"custom_field_5": {
"type": "string"
}
}
}
}
}
UpdatePATCH/transactions/{id}/details
Update transaction details
Body parameters
Name | Type | Description |
---|---|---|
transaction_details.external_order_id | string | Optional. The external order ID |
transaction_details.custom_field_1 | string | Optional. max:255, min:1 |
transaction_details.custom_field_2 | string | Optional. max:255, min:1 |
transaction_details.custom_field_3 | string | Optional. max:255, min:1 |
transaction_details.custom_field_4 | string | Optional. max:255, min:1 |
transaction_details.custom_field_5 | string | Optional. max:255, min:1 |
Examples
PATCH /transactions/1901934/details
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"external_order_id": "ORD374938098",
"custom_field_1": "83648-3836"
}
Response Body
{
"data": {
"external_order_id": "ORD374938098",
"custom_field_1": "83648-3836",
"custom_field_2": null,
"custom_field_3": null,
"custom_field_4": null,
"custom_field_5": null
}
}
- id
number
(required) Example: 1234The Transaction ID.
Rewards ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 123,
"title": "$10 Discount",
"points": 1000
},
{
"id": 124,
"title": "$20 Discount",
"points": 3000
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
GET/rewards
List Catalog Items.
Example
GET /rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 12456,
"points": 1000,
"expires_at": "2026-04-02T03:59:59+00:00",
"amount": 25,
"real_value": 0,
"discount_value": 0,
"partner_reward": false,
"redeem_for_gift_card": false,
"type": "redeem_discount_amount",
"title": "Cash back 25$",
"description": "Cash back 25$",
"external_id": "123456789",
"images": []
}
]
}
Note: In order to redeem points for cashback create a transaction and use a catalog item that has
redeem_for_gift_card
attribute true. This will convert points to gift card balance (wallet balance)
Reward Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": {
"id": 123,
"title": "$20 Discount",
"points": 3000,
"amount": 25,
"partner_reward": false,
"redeem_for_gift_card": false,
"external_id": "123456789"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"title": {
"type": "string"
},
"points": {
"type": "number"
},
"amount": {
"type": "number"
},
"partner_reward": {
"type": "boolean",
"description": "When the item is a partner reward"
},
"redeem_for_gift_card": {
"type": "boolean",
"description": "When redeeming this item it will convert points to giftcard balance"
},
"external_id": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"code": 404,
"error": "Not found",
"message": "Catalog Item not found"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"code": {
"type": "number"
},
"error": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
GET/rewards/{id}
Returns a single catalog item.
Path Parameters
Name | Type | Description |
---|---|---|
id | number | Catalog Item ID |
- id
number
(required) Example: 123The unique ID.
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Body
{
"type_id": 18,
"points": 100,
"shipping_option": 1,
"targeted_flag": 2,
"redemption_user_tiers": [
{
"id": 1238
}
],
"publish_at": "2020-04-01T03:59:59+0000",
"expires_at": "2020-04-01T03:59:59+0000",
"never_expires_flag": 0,
"amount": 0,
"discount_value: 10 (number) - Required if type_id is 18, the discount percentage %": "Hello, world!",
"real_value: 0 (number) - Required if type_id is 15, the free product's price": "Hello, world!",
"partner_reward": false,
"external_id": "123456789",
"redeem_for_gift_card: false (boolean) - Required if type_id is 16, if it's converted to Gift Card": "Hello, world!",
"reward_languages": [
{
"language_id": 1,
"title": "10% off",
"description": "Enjoy the 10% off",
"terms_conditions": "Offer terms and conditions here",
"link": "https://example.com/some-offer-link"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
],
"available_at_branches": [
{
"id": "1es8uwrs8sd9fsf9"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type_id": {
"type": "number",
"description": "The reward type ID 15,16,18"
},
"points": {
"type": "number",
"description": "The points for redeeming this reward"
},
"shipping_option": {
"type": "number",
"description": "1 Free shipping, 2 The discount will be applied to shipping, 3 The discount won't be applied to shipping,"
},
"targeted_flag": {
"type": "number",
"description": "0 Available for everyone, 1 Targeted, 2 Specific tier"
},
"redemption_user_tiers": {
"type": "array"
},
"publish_at": {
"type": "string",
"description": "When the reward will be published"
},
"expires_at": {
"type": "string",
"description": "When the reward will be expired"
},
"never_expires_flag": {
"type": "number",
"description": "1 the reward will never expire, 0 it will expire"
},
"amount": {
"type": "number",
"description": "Required if type_id is 16, the discount amount for cashback"
},
"discount_value: 10 (number) - Required if type_id is 18, the discount percentage %": {
"type": "string"
},
"real_value: 0 (number) - Required if type_id is 15, the free product's price": {
"type": "string"
},
"partner_reward": {
"type": "boolean",
"description": "When the item is a partner reward"
},
"external_id": {
"type": "string"
},
"redeem_for_gift_card: false (boolean) - Required if type_id is 16, if it's converted to Gift Card": {
"type": "string"
},
"reward_languages": {
"type": "array"
},
"images": {
"type": "array"
},
"available_at_branches": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 123,
"title": "$20 Discount",
"points": 3000,
"amount": 25,
"partner_reward": false,
"redeem_for_gift_card": false,
"external_id": "123456789"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"title": {
"type": "string"
},
"points": {
"type": "number"
},
"amount": {
"type": "number"
},
"partner_reward": {
"type": "boolean",
"description": "When the item is a partner reward"
},
"redeem_for_gift_card": {
"type": "boolean",
"description": "When redeeming this item it will convert points to giftcard balance"
},
"external_id": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"type_id": [
"Type id is required."
],
"images": [
"The images may not have more than 4 items."
],
"reward_languages": [
"Reward languages is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type_id": {
"type": "array"
},
"images": {
"type": "array"
},
"reward_languages": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Expiration date cannot be prior to publish date."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Create a rewardPOST/rewards/
A reward also called redemption catalog can have 3 main types:
-
Free Product type_id: 15
-
Cashback type_id: 16
-
Percentage type_id: 18
A reward can be for all customers, only for targeted customers, or only for specific tier customers. A reward can only avaliable for the specific branches or all branches.
NOTE: Reward images MUST be uploaded first using POST /rewards/images endpoint, before creating a reward Images must be uploaded to Kangaroo Rewards. External images are not allowed
Body parameters common for all reward types
Name | Type | Description |
---|---|---|
type_id | integer | Required Reward Type. Possible options: 15 - Free Product, 16 - Cashback, 18 - Percentage |
shipping_option | integer | Shipping option, only applicable in the ecom branch. Possible options: null, 1 Free shipping, 2 The discount will be applied to shipping, 3 The discount won’t be applied to shipping |
targeted_flag | integer | Required (0,1,2) Default 1 - targeted customers, 0 - available for everyone, 2 - available for tier customers |
never_expires_flag | integer | Optional Default 0 - expires_at is required, 1 - the reward will never expire |
publish_at | string | Optional Default current date and time. ISO 8601 date. Example: 2000-07-01T00:00:00+00:00 |
expires_at | string | Required if never_expires_flag = 0. ISO 8601 date. Example: 2000-07-01T00:00:00+00:00 |
redemption_user_tiers | array | Required if targeted_flag = 2. |
redemption_user_tiers.*.id | integer | Required The tier ID. |
points | integer | Required The points for redeeming this reward. |
partner_reward | boolean | This is a Partner Reward or not, only work for the business who enable the partner reward feature. |
external_id | string |
Optional External Id |
images | array | Required Up to 4 items |
images.*.original | string,url | Required The uploaded image path, max: 255 |
images.*.large | string,url | Required The uploaded image path, max: 255 |
images.*.*medium | string,url | Required The uploaded image path, max: 255 |
images.*.thumbnail | string,url | Required The uploaded image path, max: 255 |
available_at_branches | array | Required - A list of branches where the reward is available at. At least one branch is required |
available_at_branches.*.id | string | Required Branch ID |
reward_languages | array | Required - At least English is required, language_id = 1 |
reward_languages.*.language_id | integer | Required - 1 - English, 2 - French, 3 - Spanish |
reward_languages.*.title | string | Required - Reward title in that language. max: 255 |
reward_languages.*.description | string | Optional - Reward description in that language. max 500 |
reward_languages.*.terms_conditions | string | Optional - Terms and conditions. max: 1000 |
reward_languages.*.link | string,url | Optional - An external link for reward. max: 100 |
Body parameters specific for reward type is free product only
All common +
Name | Type | Description |
---|---|---|
real_value | numeric | Required. The free item’s amount, it’s used as redeeming amount. |
Body parameters specific for reward type is cashback only
All common +
Name | Type | Description |
---|---|---|
amount | numeric | Required. The cashback amount, it’s used as redeeming amount. |
redeem_for_gift_card | boolean | Required. Convert this reward to Gift Card. |
Body parameters specific for reward type is pecentage only
All common +
Name | Type | Description |
---|---|---|
discount_value | integer | Required. The discount percentage. |
Example creating a free product reward
POST /rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"type_id": 15,
"points": 50,
"targeted_flag": 2,
"redemption_user_tiers": [
{
"id": 51
},
{
"id": 52
}
],
"expires_at": "2020-07-17T18:10:05-00:00",
"never_expires_flag": 0,
"real_value": 13.99,
"reward_languages": [
{
"language_id": 1,
"title": "Free Product",
"description": "Available only for Platinum and Gold customer",
"terms_conditions": "",
"link": "https://example.com/some-reward"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Example creating a percentage reward
POST /rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"type_id": 18,
"points": 50,
"targeted_flag": 0,
"never_expires_flag": 1,
"discount_value": 10,
"reward_languages": [
{
"language_id": 1,
"title": "10% OFF",
"description": "",
"terms_conditions": "",
"link": "https://example.com/some-reward"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Example creating a cashback reward
POST /rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"type_id": 16,
"points": 50,
"targeted_flag": 1,
"never_expires_flag": 1,
"amount": 25.00,
"redeem_for_gift_card": false,
"reward_languages": [
{
"language_id": 1,
"title": "$25 OFF",
"description": "",
"terms_conditions": "",
"link": "https://example.com/some-reward"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Product Rewards ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "123",
"points": 1000,
"product": {
"id": 123,
"title": "Product Reward 1",
"description": "Product Reward Description"
}
},
{
"id": "124",
"points": 3000,
"product": {
"id": 124,
"title": "Product Reward 2",
"description": "Product Reward Description"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
GET/product-rewards
List Product Rewards.
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
relationships | enum | Optional. You can optionally request that the response include the product object. To do so, specify relationships=product in your request. |
products.product_sku | string | Optional. Filter products by SKU |
Example
GET /product-rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123,
"points": 300
}
]
}
Example with Product
GET /product-rewards?relationships=product HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123,
"points": 300,
"product": {
"id": 120348,
"title": "Product Reward 1",
"description": "",
"images": []
}
}
]
}
Example filter by product SKU
To filter on a field, simply add that field and its value to the query.: products.product_sku=eq|EBCS345
The operator eq
translates to =
GET /product-rewards?relationships=product&products.product_sku=eq|EBCS345 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
- relationships
string
(optional) Default: nullInclude relationships in the response. Example
relationships=product
Offers ¶
Offer ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Body
{
"type_id": 8,
"offer_frequency_id": 1,
"targeted_offer_flag": 1,
"publish_at": "2020-04-01T03:59:59+0000",
"expires_at": "2020-04-01T03:59:59+0000",
"never_expires_flag": 1,
"discount_value": 10,
"offer_languages": [
{
"language_id": 1,
"title": "Free coffee",
"description": "Come on Monday and get a free coffee",
"offer_terms_conditions": "Offer terms and conditions here",
"offer_link": "https://example.com/some-offer-link"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
],
"available_at_branches": [
{
"id": "1es8uwrs8sd9fsf9"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type_id": {
"type": "number",
"description": "The offer type ID 8,8,10"
},
"offer_frequency_id": {
"type": "number",
"description": "Daily, weekly, specific day and time"
},
"targeted_offer_flag": {
"type": "number",
"description": "0 Available for everyone, 1 Targeted"
},
"publish_at": {
"type": "string",
"description": "When the offer will be published"
},
"expires_at": {
"type": "string"
},
"never_expires_flag": {
"type": "number",
"description": "1 the offer will never expire, 0 it will expire"
},
"discount_value": {
"type": "number"
},
"offer_languages": {
"type": "array"
},
"images": {
"type": "array"
},
"available_at_branches": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type_id": 8,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png",
"default": true
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"points": {
"type": "number"
},
"expires_at": {
"type": "string"
},
"discount_value": {
"type": "number"
},
"multip_factor": {
"type": "number"
},
"real_value": {
"type": "number"
},
"type_id": {
"type": "number",
"description": "The offer type ID"
},
"type": {
"type": "string"
},
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"images": {
"type": "array"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"type_id": [
"Type id is required."
],
"images": [
"The images may not have more than 4 items."
],
"offer_languages": [
"Offer languages is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type_id": {
"type": "array"
},
"images": {
"type": "array"
},
"offer_languages": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Expiration date cannot be prior to publish date."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Create offerPOST/offers
An offer contains information about a free product, percent-off or amount-off discount you might want to apply to a customer. Currently 4 main types of offers can be created:
-
Basic Offer (coupon) type_id: 8,9,10
-
Points Multiplier type_id: 1
-
Free Product type_id: 2
-
Bonus Points type_id: 3
Basic Offers can be claimed as coupoons and after that, the coupon can be redeemed. See #Offer Types
NOTE: Offer images MUST be uploaded first using POST /offers/images endpoint, before creating an offer Images must be uploaded to Kangaroo Rewards. External images are not allowed
Body parameters common for all offer types
Name | Type | Description |
---|---|---|
type_id | integer | Required Offer Type. Possible options: 1 - Points Multiplier, 2 - Free Product, 3 - Bonus Points, 8 - Basic Offer (Free product), 9 - Basic Offer (percent-off), 10 - Basic Offer (amount-off) |
targeted_offer_flag | integer | Required (0,1) Default 1 - targeted customers, 0 - available for everyone |
never_expires_flag | integer | Optional Default 0 - expires_at is required, 1 - the offer will never expire |
publish_at | string | Optional Default current date and time. ISO 8601 date. Example: 2000-07-01T00:00:00+00:00 |
expires_at | string | Required if never_expires_flag = 0. ISO 8601 date. Example: 2000-07-01T00:00:00+00:00 |
images | array | Required Up to 4 items |
images.*.original | string,url | Required The uploaded image path, max: 255 |
images.*.large | string,url | Required The uploaded image path, max: 255 |
images.*.*medium | string,url | Required The uploaded image path, max: 255 |
images.*.thumbnail | string,url | Required The uploaded image path, max: 255 |
available_at_branches | array | Required - A list of branches where the offer is available at. At least one branch is required |
available_at_branches.*.id | string | Required Branch ID |
offer_languages | array | Required - At least English is required, language_id = 1 |
offer_languages.*.language_id | integer | Required - 1 - English, 2 - French, 3 - Spanish |
offer_languages.*.offer_title | string | Required - Offer title in that language. max: 255 |
offer_languages.*.offer_description | string | Optional - Offer description in that language. max 500 |
offer_languages.*.offer_terms_conditions | string | Optional - Terms and conditions. max: 1000 |
offer_languages.*.offer_link | string,url | Optional - An external link for offer. max: 100 |
Body parameters specific for Basic Offer only
All common +
Name | Type | Description |
---|---|---|
coupon_auto_get | integer | Optional (0,1) Default 0 - Must click “Get Coupon” on the App, 1 - The coupon will be auto-claimed |
coupon_perm_available | integer | Optional (0,1) Default 0 - Not permanently available, 1 - Permanently available |
coupon_expires_in | integer | Optional - The number of days the coupon must be claimed before it will expire. Default 0 - never expires, > 0 Coupon must be claimed within x days |
discount_value | numeric | Required if type_id is 9 or 10 - amount off > 0 or percent off > 0 and <=100 |
Body parameters specific for Points Multiplier Offer
All common +
Name | Type | Description |
---|---|---|
offer_frequency_id | integer | Required Default 1. Possible options: 1 - Daily, 2 - Weekly, 3 - Specific day and time, 4 - Once |
multip_factor | numeric | Required Multiplication factor > 0. Example: 2 - 2x the points, 1.5 - 1.5x the points |
min_purchase | numeric | Required - Minimum purchase amount >= 0 |
max_purchase | numeric | Required - Maximum purcase amount, cannot be less than min purchase amount |
apps_only | boolean | Optional Default false. If true - Customers must log in with QR code to be eligible |
freq_details | array | Required when offer frequency id is 3 (Specific day and time). Must be an array in the following format: [“1”,“2”,“3”,“4”,“5”,“6”,“7”] |
peak_from | string,time | Required with frequncy details. 24-hour format of an hour with leading zeros and minutes with leading zeros. Min: 00:00, max: 23:59. Must be before peak_to Ex: “09:00” |
peak_to | string,time | Required with frequncy details. 24-hour format of an hour with leading zeros and minutes with leading zeros. Min: 00:00, max: 23:59. Must be after peak_from Ex: “17:45” |
NOTE: freq_details field must be an array in the following format: [“1”,“2”,“3”,“4”,“5”,“6”,“7”] containing the days of the week from Monday to Sunday as numbers. Where 1 - Monday, 2 - Tuesday … 7 - Sunday Example: [“1”,“2”] - available only on Monday and Tuesday Example: [“5”] - available only on Friday
Body parameters specific for Free Product Offer
All common + Points Multiplier Offer (exept multip_factor) +
Name | Type | Description |
---|---|---|
offer_languages.*.offer_product | string | Required - Product description. max: 255 |
Body parameters specific for Bonus Points Offer
All common + Points Multiplier Offer (exept multip_factor) +
Name | Type | Description |
---|---|---|
points | integer | Required - Bonus points to offer |
Example creating a Basic Offer
POST /offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"type_id": 9,
"targeted_offer_flag": 0,
"expires_at": "2020-07-17T18:10:05-00:00",
"never_expires_flag": 0,
"discount_value": 10,
"offer_languages": [
{
"language_id": 1,
"offer_title": "10% OFF today",
"offer_description": "Available only for today",
"offer_terms_conditions": "",
"offer_link": "https://example.com/some-offer"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Example creating a Points Multiplier Offer
POST /offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"type_id": 1,
"offer_frequency_id" : 3,
"multip_factor": 2,
"min_purchase": 10,
"max_purchase": 1000,
"apps_only": false,
"freq_details": ["1","2","5"],
"peak_from": "09:00",
"peak_to": "17:30",
"targeted_offer_flag": 0,
"expires_at": "2020-07-17T18:10:05-04:00",
"never_expires_flag": 0,
"offer_languages": [
{
"language_id": 1,
"offer_title": "2x the points on Monday and Tuesday"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Offer Title",
"points": "3000",
"type_id": 1,
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
},
{
"id": "112",
"title": "Offer Title 2",
"points": "50000",
"type_id": 2,
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all offersGET/offers
List all offers, including expired and targeted
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include the branch object. To do so, specify relationships=branch in your request. |
page | int | Optional. The page number |
per_page | int | Optional. Items per page |
products.product_sku | string | Optional. Filter by product SKU |
pos_items_group.tag_name | string | Optional. Filter by product tag name |
-
type_id: 1 - Points Multiplier
-
type_id: 2 - Free product
-
type_id: 3 - Bonus points
-
type_id: 8 - Basic offer (coupon). Type free product (can be used as a coupon)
-
type_id: 9 - Basic offer (coupon). Type discount in % (can be used as a coupon)
-
type_id: 10 - Basic offer (coupon). Type discount in $ (can be used as a coupon)
-
type_id: 14 - Gift card
NOTE: Only offers type ID 8,9,10 can be used as coupons A coupon MUST be assigned to customer before it will be available for redemption In order to assign a coupon to a customer create a transaction with intent:
get_coupon
, customer and offer object
Example
GET /offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [...]
}
]
}
Relationships
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object in the response
The value of the relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /offers?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
]
}
Filter offers by product SKU
GET /offers?products.product_sku=eq|SKERR23423SF9SD934I
Filter offers by product tag name
GET /offers?pos_items_group.tag_name=eq|promo
Offer ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type_id": 8,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png",
"default": true
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"points": {
"type": "number"
},
"expires_at": {
"type": "string"
},
"discount_value": {
"type": "number"
},
"multip_factor": {
"type": "number"
},
"real_value": {
"type": "number"
},
"type_id": {
"type": "number",
"description": "The offer type ID"
},
"type": {
"type": "string"
},
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"images": {
"type": "array"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Offer 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Retrieve an offerGET/offers/{offer_id}
Returns a single offer.
Path Parameters
Name | Type | Description |
---|---|---|
offer_id | number | Required. The ID of the offer |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include related objects. To do so, specify for example: relationships=branch in your request. |
Example
GET /offers/{offer_id}?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [
{
"default": true,
"large": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
}
}
- offer_id
string
(required) Example: 123The unique ID of the offer.
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Body
{
"offer_frequency_id": 1,
"targeted_offer_flag": 1,
"publish_at": "2020-04-01T03:59:59+0000",
"expires_at": "2020-04-01T03:59:59+0000",
"never_expires_flag": 1,
"discount_value": 10,
"offer_languages": [
{
"language_id": 1,
"title": "Free coffee",
"description": "Come on Monday and get a free coffee",
"offer_terms_conditions": "Offer terms and conditions here",
"offer_link": "https://example.com/some-offer-link"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
],
"available_at_branches": [
{
"id": "1es8uwrs8sd9fsf9"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"offer_frequency_id": {
"type": "number",
"description": "Daily, weekly, specific day and time"
},
"targeted_offer_flag": {
"type": "number",
"description": "0 Available for everyone, 1 Targeted"
},
"publish_at": {
"type": "string",
"description": "When the offer will be published"
},
"expires_at": {
"type": "string"
},
"never_expires_flag": {
"type": "number",
"description": "1 the offer will never expire, 0 it will expire"
},
"discount_value": {
"type": "number"
},
"offer_languages": {
"type": "array"
},
"images": {
"type": "array"
},
"available_at_branches": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 552,
"points": 0,
"expires_at": "2017-04-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 2,
"real_value": 0,
"type_id": 8,
"type": "points_multiplier",
"title": "Multiply your points",
"description": "Come on Monday and Multiply your points",
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png",
"default": true
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"points": {
"type": "number"
},
"expires_at": {
"type": "string"
},
"discount_value": {
"type": "number"
},
"multip_factor": {
"type": "number"
},
"real_value": {
"type": "number"
},
"type_id": {
"type": "number",
"description": "The offer type ID"
},
"type": {
"type": "string"
},
"title": {
"type": "string"
},
"description": {
"type": "string"
},
"images": {
"type": "array"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"type_id": [
"Type id is required."
],
"images": [
"The images may not have more than 4 items."
],
"offer_languages": [
"Offer languages is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"type_id": {
"type": "array"
},
"images": {
"type": "array"
},
"offer_languages": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Expiration date cannot be prior to publish date."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Offer 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Update an offerPUT/offers/{offer_id}
Update an offer.
Path Parameters
Name | Type | Description |
---|---|---|
offer_id | number | Required. The ID of the offer |
NOTE:
type_id
cannot be changed.
All fields can be updated similar to creating excepttype_id
andoffer_id
On update all fields are optional.
Example updating an offer
PUT /offers/123 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"targeted_offer_flag": 0,
"expires_at": "2020-07-17T18:10:05-00:00",
"never_expires_flag": 0,
"discount_value": 10,
"offer_languages": [
{
"language_id": 1,
"offer_title": "10% OFF today",
"offer_description": "Available only for today",
"offer_terms_conditions": "",
"offer_link": "https://example.com/some-offer"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Example updating only title
PUT /offers/123 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"offer_languages": [
{
"language_id": 1,
"offer_title": "10% OFF today"
}
}
- offer_id
string
(required) Example: 123The unique ID of the offer.
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Offer 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Delete an offerDELETE/offers/{offer_id}
Delete an offer
Path Parameters
Name | Type | Description |
---|---|---|
offer_id | number | Required. The ID of the offer |
Example
DELETE /offers/123 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
- offer_id
string
(required) Example: 123The unique ID of the offer.
Offer Images ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: multipart/form-data;boundary=----WebKitFormBoundary8M3sSU13ul5lXSJm
Body
------WebKitFormBoundary8M3sSU13ul5lXSJm
Content-Disposition: form-data; name="image_original"; filename="image.jpg"
Content-Type: image/jpeg
content of image...
------WebKitFormBoundary8M3sSU13ul5lXSJm
Content-Disposition: form-data; name="image_large"; filename="image1.jpg"
Content-Type: image/jpeg
content of image...
------WebKitFormBoundary8M3sSU13ul5lXSJm
Content-Disposition: form-data; name="image_medium"; filename="image2.jpg"
Content-Type: image/jpeg
content of image...
------WebKitFormBoundary8M3sSU13ul5lXSJm
Content-Disposition: form-data; name="image_thumbnail"; filename="image3.jpg"
Content-Type: image/jpeg
content of image...
------WebKitFormBoundary8M3sSU13ul5lXSJm--
Headers
Content-Type: application/json
Body
{
"data": {
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image1.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image1.png"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"original": {
"type": "string"
},
"large": {
"type": "string"
},
"medium": {
"type": "string"
},
"thumbnail": {
"type": "string"
}
}
}
}
}
POST/offers/images
Uploading new images. Supported image format: jpeg, jpg, png, gif
NOTE: Images must be uploaded to Kangaroo Rewards. Only binary format is supported. External images are NOT allowed: a remote HTTP or HTTPS URL, a private storage URL, a base-64 data URI, an FTP URL, or a Cloud Storage URL.
Large, medium and thubnail images MUST be cropped before uploading with Aspect ratio: 1.7:1
Large - Minimum dimensions: 800 x 470 px
Medium - Minimum dimensions: 606 x 356 px
Thumbnail - Minimum dimensions: 450 x 264 px
Body parameters common for all offer types
Name | Type | Description |
---|---|---|
image_original | binary | Required original image. max: 10MB |
image_large | binary | Required large size image. max: 1MB. Minimum dimensions: 450 x 264 px |
image_medium | binary | Required medium size image. max: 512KB. Minimum dimensions: 450 x 264 px |
image_thumbnail | binary | Required thumbnail size image. max: 256KB. Minimum dimensions: 450 x 264 px |
Example
POST /offers/images HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: multipart/form-data; boundary=AaB03x
--AaB03x
Content-Disposition: form-data; name="image_original" filename="image.png"
content of image.png ...
--AaB03x
Content-Disposition: form-data; name="image_large" filename="image1.png"
content of image1.png ...
--AaB03x
Content-Disposition: form-data; name="image_medium" filename="image2.png"
content of image2.png ...
--AaB03x
Content-Disposition: form-data; name="image_thumbnail" filename="image3.png"
content of image3.png ...
Response Body
{
"data": {
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
}
Products ¶
Product ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"promote_flag": 1,
"product_sku": "AB1234",
"product_actual_price": 10.5,
"product_real_price": 10.5,
"product_languages": [
{
"language_id": 1,
"product_title": "Product A",
"product_description": "Product A description.",
"product_terms_conditions": "Product A terms and conditions.",
"product_link": "https://example.com/some-product-link"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 1,
"title": "Product A",
"description": "Product A description",
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
],
"product_sku": "AB123",
"actual_price": 10.5,
"real_price": 10.5,
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"product_languages": [
{
"language_id": 1,
"title": "Product A",
"description": "Product A description.",
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"language": [
{
"id": 1,
"abbreviation": "en",
"name": "English"
}
]
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The product ID 1,2,3"
},
"title": {
"type": "string",
"description": "Product title"
},
"description": {
"type": "string",
"description": "Product description"
},
"images": {
"type": "array"
},
"product_sku": {
"type": "string"
},
"actual_price": {
"type": "number"
},
"real_price": {
"type": "number"
},
"terms_conditions": {
"type": "string"
},
"link": {
"type": "string"
},
"product_languages": {
"type": "array"
}
}
}
}
}
Create productPOST/products
Create product
Body parameters
Name | Type | Description |
---|---|---|
promote_flag | integer | Optional (0,1) Default 0 |
product_sku | string | Optional Product stock-keeping unit. Min: 3 characters, max: 100 characters |
product_actual_price | numeric | Optional Product actual price. Default 0 |
product_real_price | numeric | Optional Product real price. Default 0 |
images | array | Optional Up to 4 items |
images.*.original | string,url | Optional The uploaded image path, max: 255 |
images.*.large | string,url | Optional The uploaded image path, max: 255 |
images.*.medium | string,url | Optional The uploaded image path, max: 255 |
images.*.thumbnail | string,url | Optional The uploaded image path, max: 255 |
available_at_branches | array | Required - A list of branches where the product is available at. At least one branch is required |
available_at_branches.*.id | string | Required Branch ID |
product_languages | array | Required - At least English is required, language_id = 1 |
product_languages.*.language_id | integer | Required - 1 - English, 2 - French, 3 - Spanish |
product_languages.*.product_title | string | Required - Product title in that language. max: 255 |
product_languages.*.product_description | string | Optional - Product description in that language. max 500 |
product_languages.*.product_terms_conditions | string | Optional - Product terms and conditions. max: 1000 |
product_languages.*.product_link | string,url | Optional - An external link for product. max: 100 |
Example: Create product
POST /products HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
X-Application-Key: eyXai0XD7SLLzaOeJW...
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"promote_flag": 1,
"product_sku": "AB1234",
"product_actual_price": 10.50,
"product_real_price": 10.50,
"product_languages": [
{
"language_id": 1,
"product_title": "Product A",
"product_description": "Product A description.",
"product_terms_conditions": "Product A terms and conditions.",
"product_link": "https://example.com/some-product-link"
}
],
"available_at_branches": [
{
"id": "11eaa5c938ec36cc9df9b42e99312ac8"
},
{
"id": "12eac6c914dfbc4e8478b42e99312ac5"
}
],
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
]
}
Response Body
{
"data": {
"id": 1,
"title": "Product A",
"description": "Product A description.",
"images": [
{
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
"path": "https://www.kangaroorewards.com/photos/image.png",
"default": true,
}
],
"product_sku": "AB1234",
"actual_price": 10.50,
"real_price": 10.50,
"terms_conditions": "",
"link": "https://example.com/some-product-link",
"product_languages": [
{
"language_id": 1,
"title": "Product A",
"description": "Product A description.",
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"language": {
"id":1,
"abbreviation": "en",
"name": "English"
}
}
]
}
}
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 1,
"title": "Product A",
"description": "Product A description",
"images": [
{
"original": "https://www.kangaroorewards.com/photos/image.png",
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
}
],
"product_sku": "AB123",
"actual_price": 10.5,
"real_price": 10.5,
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"product_languages": [
{
"language_id": 1,
"title": "Product A",
"description": "Product A description.",
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"language": [
{
"id": 1,
"abbreviation": "en",
"name": "English"
}
]
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all productsGET/products
List all products
Example
GET /products HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1,
"title": "Product A",
"description": "Product A description",
"images": [
{
"large": "https://www.kangaroorewards.com/photos/image1.png",
"medium": "https://www.kangaroorewards.com/photos/image2.png",
"thumbnail": "https://www.kangaroorewards.com/photos/image3.png"
"path": "https://www.kangaroorewards.com/photos/image.png",
"default": true,
}
],
"product_sku": "AB123",
"actual_price": 10.50,
"real_price": 10.50,
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"product_languages": [
{
"language_id": 1,
"title": "Product A",
"description": "Product A description.",
"terms_conditions": "Product A terms and conditions.",
"link": "https://example.com/some-product-link",
"language": {
"id":1,
"abbreviation": "en",
"name": "English"
}
}
]
}
]
}
Branches ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "11e696103f8ff5eabb02089e01cf89b5",
"name": "Retrorant (Gauchetiere)",
"address": {
"formatted": "1060 Boulevard Robert-Bourassa",
"city": "Montreal",
"region": "Quebec",
"country": "Canada",
"lat": 45.485169,
"long": -73.580989
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
GET/branches
This endpoint retrieves a list of all physical branches associated with the business. By default, virtual branches are excluded from the response.
To include virtual branches in the result, use the include
parameter.
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
include | string | Optional. Optionally include extra resources in the response. Ex: virtual_branch |
Example
GET /branches HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": "11e696103f8ff5eabb02089e01cf89b5",
"name": "Retrorant",
"address": {
"formatted": "1060 Boulevard Robert-Bourassa",
"city": "Montreal",
"region": "Quebec",
"country": "Canada",
"lat": 45.485169,
"long": -73.580989
}
}
]
}
Example request including virtual branches (E-commerce)
GET /branches?include=virtual_branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Tiers ¶
Resource ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 123,
"tiers_sequence": [
"1",
"2",
"3"
],
"tiers_relation": 0,
"reset_type": 1,
"reset_period_month": 1,
"base_previous_period": 0,
"enabled": false,
"tier_levels": []
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
GET/tiers
List Tiers.
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
relationships | enum | Optional. You can optionally request that the response include related resources. To do so, specify relationships=tier_levels in your request. |
Tiers sequence
The tiers_sequence
value is an array with numbers: 1,2,3
-
1 - Spend
-
2 - Points
-
3 - Visits
Tiers criterion
When tiers_relation
is 0 - At least one selected criterion
When tiers_relation
is 1 - Tiers combine all selected criterion
Example
GET /tiers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 32,
"tiers_sequence": [
"2",
"1",
"3"
],
"tiers_relation": 0,
"reset_type": 1,
"reset_period_month": 1,
"base_previous_period": 0,
"enabled": true
}
]
}
Example with Tier Levels
GET /tiers?relationships=tier_levels HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 32,
"tiers_sequence": [
"1",
"2",
"3"
],
"tiers_relation": 0,
"reset_type": 1,
"reset_period_month": 1,
"base_previous_period": 0,
"enabled": true,
"tier_levels": [
{
"id": 25,
"name": "VIP",
"reach_spend": 1,
"reach_visits": 1,
"reach_points": 1
},
{
"id": 26,
"name": "Platinum",
"reach_spend": 2,
"reach_visits": 2,
"reach_points": 2
},
{
"id": 27,
"name": "Gold",
"reach_spend": 3,
"reach_visits": 3,
"reach_points": 3
}
]
}
],
}
Referral Programs ¶
Referral program ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 123,
"cond_min_amount": 3.99,
"referee_earns": 100,
"referer_earns": 10,
"expires_in": 0,
"enabled": false,
"link_referral_enabled": true
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List referral programsGET/referral-programs
List Referral Programs.
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
Example
GET /referral-programs HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1056,
"rule_name": "REFERRAL_RULES_PURCHASE",
"cond_min_amount": 5,
"referee_earns": 10,
"referer_earns": 50,
"expires_in": 60,
"enabled": true,
"link_referral_enabled": true,
"referral_link": ""
}
]
}
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Body
{
"cond_min_amount": 10,
"referee_earns": 150,
"referer_earns": 50,
"expires_in": 30,
"enabled": true,
"link_referral_enabled": true
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"cond_min_amount": {
"type": "number",
"description": "The minimum purchase amount condition."
},
"referee_earns": {
"type": "number",
"description": "How many points the referee earns"
},
"referer_earns": {
"type": "number",
"description": "How many points the referer earns"
},
"expires_in": {
"type": "number",
"description": "Expires in number of days"
},
"enabled": {
"type": "boolean"
},
"link_referral_enabled": {
"type": "boolean",
"description": "Is referral link enabled"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 123,
"cond_min_amount": 3.99,
"referee_earns": 100,
"referer_earns": 10,
"expires_in": 0,
"enabled": false,
"link_referral_enabled": true
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"cond_min_amount": {
"type": "number"
},
"referee_earns": {
"type": "number",
"description": "How many points the referee earns"
},
"referer_earns": {
"type": "number",
"description": "How many points the referrer earns"
},
"expires_in": {
"type": "number",
"description": "Expires in number of days"
},
"enabled": {
"type": "boolean",
"description": "Active or Inactive"
},
"link_referral_enabled": {
"type": "boolean",
"description": "Is referral link enabled"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"cond_min_amount": [
"The cond min amount field is required."
],
"expires_in": [
"The expires in field is required."
],
"referee_earns": [
"The referee earns field is required."
],
"referer_earns": [
"The referer earns field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"cond_min_amount": {
"type": "array"
},
"expires_in": {
"type": "array"
},
"referee_earns": {
"type": "array"
},
"referer_earns": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Create a new referral programPOST/referral-programs
Create a referral program. Currently the business can only have one referral program enabled. So this request will disable the current referral program.
Name | Type | Description |
---|---|---|
cond_min_amount | numeric | Required The minimum purchase amount condition. |
expires_in | integer | Required Expires in number of days. |
referee_earns | integer | Required How many points the referee earns. |
referer_earns | integer | Required How many points the referer earns. |
link_referral_enabled | boolean | Optional Default true. Is referral link enabled. |
enabled | boolean | Optional Default true |
Example creating a referral program
POST /referral-programs HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"cond_min_amount": 30.00,
"referee_earns": 50,
"referer_earns": 10,
"expires_in": 35,
"enabled": true,
"link_referral_enabled": true
}
Business Rules ¶
Business Rules ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": {
"earning": {
"reward_ratio": 10,
"reward_milestone_amount": 1,
"reward_points": 100,
"reward_amount": 1,
"welcome_points": 100,
"round_up_points": true,
"tiers_earning_override": true,
"tiers_earning_rules": [
{
"id": 78,
"amount": 1,
"points": 15
}
]
},
"redeeming": {
"redemption_ratio": 2,
"redemption_amount": 1
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"earning": {
"type": "object",
"properties": {
"reward_ratio": {
"type": "number",
"description": "The number of points customer will earn for each $1"
},
"reward_milestone_amount": {
"type": "number",
"description": "For each $1"
},
"reward_points": {
"type": "number",
"description": "ALIAS"
},
"reward_amount": {
"type": "number",
"description": "ALIAS"
},
"welcome_points": {
"type": "number",
"description": "Number of welcome points"
},
"round_up_points": {
"type": "boolean",
"description": "Round up earning points."
},
"tiers_earning_override": {
"type": "boolean",
"description": "Override earning rules based on Tiers."
},
"tiers_earning_rules": {
"type": "array",
"description": "Earning rules based on Tiers."
}
}
},
"redeeming": {
"type": "object",
"properties": {
"redemption_ratio": {
"type": "number",
"description": "Redemption ratio. Only for E-comm"
},
"redemption_amount": {
"type": "number",
"description": "Redemption amount. Only for E-comm"
}
}
}
}
}
}
}
Retrieve Business RulesGET/rules
Retrieving existing business rules.
Example
GET /rules HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": {
"earning": {
"reward_ratio": 100,
"reward_amount": 1,
"reward_points": 100,
"reward_milestone_amount": 1,
"welcome_points": 100
},
"redeeming": {
"redemption_ratio": 2,
"redemption_amount": 1
},
"currency": null
}
}
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Body
{
"reward_ratio": 10,
"reward_milestone_amount": 1,
"redemption_ratio": 2,
"redemption_amount": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"reward_ratio": {
"type": "number",
"description": "The number of points customer will earn for each $1"
},
"reward_milestone_amount": {
"type": "number",
"description": "For each $1"
},
"redemption_ratio": {
"type": "number",
"description": "Redemption ratio. Only for E-comm"
},
"redemption_amount": {
"type": "number",
"description": "Redemption amount. Only for E-comm"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"earning": {
"reward_ratio": 10,
"reward_milestone_amount": 1,
"reward_points": 100,
"reward_amount": 1,
"welcome_points": 100,
"round_up_points": true,
"tiers_earning_override": true,
"tiers_earning_rules": [
{
"id": 78,
"amount": 1,
"points": 15
}
]
},
"redeeming": {
"redemption_ratio": 2,
"redemption_amount": 1
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"earning": {
"type": "object",
"properties": {
"reward_ratio": {
"type": "number",
"description": "The number of points customer will earn for each $1"
},
"reward_milestone_amount": {
"type": "number",
"description": "For each $1"
},
"reward_points": {
"type": "number",
"description": "ALIAS"
},
"reward_amount": {
"type": "number",
"description": "ALIAS"
},
"welcome_points": {
"type": "number",
"description": "Number of welcome points"
},
"round_up_points": {
"type": "boolean",
"description": "Round up earning points."
},
"tiers_earning_override": {
"type": "boolean",
"description": "Override earning rules based on Tiers."
},
"tiers_earning_rules": {
"type": "array",
"description": "Earning rules based on Tiers."
}
}
},
"redeeming": {
"type": "object",
"properties": {
"redemption_ratio": {
"type": "number",
"description": "Redemption ratio. Only for E-comm"
},
"redemption_amount": {
"type": "number",
"description": "Redemption amount. Only for E-comm"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"reward_milestone_amount": [
"The reward milestone amount must be at least 1."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"reward_milestone_amount": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"message": "This action is unauthorized."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 400,
"message": "Bad Request",
"description": "Expiration date cannot be prior to publish date."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Update Business RulesPATCH/rules
Update business rules.
Body Parameters
Name | Type | Description |
---|---|---|
reward_ratio | numeric | Optional. The number of points customer will earn for each $1. Positive number. Minimum: 0 |
reward_milestone_amount | numeric | Optional. For each $x. Positive number. Minimum: 1 |
redemption_ratio | numeric | Optional. Redemption ratio. Only for E-comm |
redemption_amount | numeric | Optional. Redemption amount. Only for E-comm |
round_up_points | boolean | Optional. Round up earning points. The reward points calculation involves taking the floor value of the amount spent divided by the reward milestone amount, then multiplying by the reward ratio to get the reward points. If the round-up earning points feature is enabled, then round up the calculated reward points. |
tiers_earning_override | boolean | Optional. Override earning rules based on Tiers |
tiers_earning_rules | array | Optional. |
tiers_earning_override.*.id | integer | Required when tiers_earning_rules is present. Tier level Id. |
tiers_earning_override.*.amount | numeric | Optional. For each $x. |
tiers_earning_override.*.points | integer | Optional. The number of points customer will earn for $amount |
Example:
PATCH /rules HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC...
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW...
Content-Type: application/json
{
"reward_ratio": 100,
"reward_milestone_amount": 1,
"redemption_ratio": 20,
"redemption_amount": 1,
"round_up_points": true,
"welcome_points": 100,
"tiers_earning_override": true,
"tiers_earning_rules": [
{
"id": 78,
"amount": 1,
"points": 15
},
{
"id": 79,
"amount": 1,
"points": 20
},
{
"id": 475,
"amount": 1,
"points": 25
}
]
}
Workflow Main Triggers ¶
Workflow Main Trigger ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 1,
"trigger_name": "Created Account",
"description": "Created Account",
"conditions": [
{
"id": 1,
"type": "list",
"label": "Branch",
"url": "https://api.kangaroorewards.com/branches?include=virtual_branch",
"relations": "null"
},
{
"id": 2,
"type": "list",
"label": "Specific Day",
"url": "null",
"relations": [
{
"label": "Monday",
"value": 1
},
{
"label": "Tuesday",
"value": 2
},
{
"label": "Wednesday",
"value": 3
},
{
"label": "Thursday",
"value": 4
},
{
"label": "Friday",
"value": 5
},
{
"label": "Saturday",
"value": 6
},
{
"label": "Sunday",
"value": 7
}
]
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all Main TriggersGET/workflow-main-triggers
Returns a list of workflow triggers
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
Response
conditions: The available conditions for the trigger.
conditions.*.url: The URL that provides selectable options for the condition.
conditions.*.relations: The selectable options for the condition.
Examples
GET /workflow-main-triggers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1,
"trigger_name": "Created Account",
"description": "",
"conditions": [
{
"id": 1,
"type": "list",
"label": "Branch",
"url": "https://api.kangaroorewards.com/branches?include=virtual_branch",
"relations": null
},
{
"id": 2,
"type": "list",
"label": "Specific Day",
"url": null,
"relations": [
{
"label": "Monday",
"value": 1
},
{
"label": "Tuesday",
"value": 2
},
{
"label": "Wednesday",
"value": 3
},
{
"label": "Thursday",
"value": 4
},
{
"label": "Friday",
"value": 5
},
{
"label": "Saturday",
"value": 6
},
{
"label": "Sunday",
"value": 7
}
]
}
]
}
]
}
Workflow Main Actions ¶
Workflow Main Action ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"data": [
{
"id": 1,
"action_name": "Email",
"description": "Email"
},
{
"id": 2,
"action_name": "Send SMS",
"description": "Send SMS"
},
{
"id": 3,
"action_name": "Reward Points",
"description": "Reward Points"
},
{
"id": 4,
"action_name": "Wait",
"description": "Delay"
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all main actionsGET/workflow-main-actions
Returns a list of workflow actions
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
Examples
GET /workflow-main-actions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 1,
"action_name": "Email",
"description": ""
},
{
"id": 2,
"action_name": "Send SMS"
"description": ""
},
{
"id": 3,
"action_name": "Reward Points"
"description": ""
},
{
"id": 4,
"action_name": "Wait"
"description": ""
}
]
}
Workflows ¶
Workflow ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 14,
"name": "Completed profile",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"expires_at": "2024-12-14T01:01:01+00:00",
"workflow_version": {
"id": 47,
"workflow_id": 14,
"external_workflow_revision_id": "000017-5a5",
"created_at": "2024-02-20T01:37:52.000000Z"
},
"triggers": [
{
"id": 29,
"main_trigger_id": 3,
"created_at": "2024-02-20T01:14:40+00:00"
}
],
"actions": [
{
"action_key: `Reward_Points_17072356685213_45745`": "",
"parent_id": 61,
"id": 61,
"main_action_id": 4,
"name": "null",
"action_key: `Wait_17072356685358_92300`": "",
"parent_id null": null,
"created_at": "2024-02-20T01:37:52+00:00",
"conditions": "null",
"relations": []
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all workflowsGET/workflows
Returns a list of workflows
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15; Maximum value 100 |
include | string | Optional. You can optionally include=triggers,actions,action_stats in your request. |
Examples
GET /workflows?include=triggers,actions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
Response Body
{
"data": [
{
"id": 14,
"name": "Completed profile",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"expires_at": "2024-12-14T01:01:01+00:00",
"workflow_version": {
"id": 47,
"workflow_id": 14,
"external_workflow_revision_id": "000017-5a5",
"created_at": "2024-02-20T01:37:52.000000Z"
},
"triggers": [
{
"id": 29,
"main_trigger_id": 3,
"created_at": "2024-02-20T01:14:40+00:00"
}
],
"actions": [
{
"id": 35,
"main_action_id": 3,
"name": null,
"action_key": "Reward_Points_17072356685213_45745",
"parent_id": 61,
"created_at": "2024-02-20T01:37:52+00:00",
"conditions": null,
"relations": [
{
"label": "point",
"value": 10,
"entity_id": null,
"entity_type": "points"
}
],
"stats": {
"completed": 3,
"failed": 0,
"in_progress": 0
}
},
{
"id": 61,
"main_action_id": 4,
"name": null,
"action_key": "Wait_17072356685358_92300",
"parent_id": null,
"created_at": "2024-02-20T01:37:52+00:00",
"conditions": null,
"relations": null,
"stats": {
"completed": 3,
"failed": 0,
"in_progress": 1
}
}
]
}
],
"links": {
"first": "http://api.kangaroorewards.com/workflows?page=1",
"last": null,
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"path": "http://api.kangaroorewards.com/workflows",
"per_page": 15,
"to": 1
}
}
Workflow ¶
Headers
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json
Content-Type: application/json
Body
{
"name": "Example Flow",
"published_at": "2024",
"expires_at": "2028",
"targeted_flag": 0,
"audience_filters: (array) - Required when targeted_flag is 1. This property represents an array of marketing filters.": "Hello, world!",
"tiers": [
"1",
"2",
"3"
],
"triggers": [
{
"main_trigger_id": 1,
"frequency": {
"freq": "hourly",
"interval": 1
},
"conditions": [
{
"id": 1,
"operator": "eq",
"value": "Hello, world!"
}
]
}
],
"actions": [
{
"main_action_id": 1,
"name": "Example action",
"action_key": "abc123",
"enabled": true,
"temp_id": 1,
"temp_parent_id": 2,
"frequency": {
"freq": "hourly",
"interval": 1
},
"relations": {
"entity_id": 1,
"entity_type": "Transaction",
"value": "123",
"label": "Transaction Type"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"name": {
"type": "string",
"description": "Required, between 1 and 255 characters in length."
},
"published_at": {
"type": "string",
"description": "03-14T09:15:22Z (string, required) - Required, follows the ISO 8601 date format."
},
"expires_at": {
"type": "string",
"description": "03-14T09:15:22Z (string) - Optional, follows the ISO 8601 date format."
},
"targeted_flag": {
"type": "number",
"description": "Required, can have a value of 0, 1, or 2. 0 represents availability for everyone, 1 for targeted customers, and 2 for tier customers."
},
"audience_filters: (array) - Required when targeted_flag is 1. This property represents an array of marketing filters.": {
"type": "string"
},
"tiers": {
"type": "array"
},
"triggers": {
"type": "array",
"description": "Required. This property represents an array of triggers."
},
"actions": {
"type": "array",
"description": "Required. Represents an array of actions."
}
},
"required": [
"name",
"targeted_flag",
"triggers",
"actions"
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 14,
"name": "Completed profile",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"expires_at": "2028-12-14T01:01:01+00:00",
"workflow_version": {
"id": 47,
"workflow_id": 14,
"external_workflow_revision_id": "000017-5a5",
"created_at": "2024-02-20T01:37:52.000000Z"
},
"triggers": [
{
"id": 29,
"main_trigger_id": 3,
"created_at": "2024-02-20T01:14:40+00:00"
}
],
"actions": [
{
"action_key: `Reward_Points_17072356685213_45745`": "",
"id": 61,
"main_action_id": 4,
"name": "null",
"action_key: `Wait_17072356685358_92300`": "",
"parent_id": "null",
"created_at": "2024-02-20T01:37:52+00:00",
"conditions": "null",
"relations": "null"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The ID"
},
"name": {
"type": "string",
"description": "The name\\"
},
"created_at": {
"type": "string",
"description": "The creation timestamp"
},
"published_at": {
"type": "string",
"description": "The publication timestamp"
},
"expires_at": {
"type": "string",
"description": "The expiration timestamp"
},
"workflow_version": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The workflow version ID"
},
"workflow_id": {
"type": "number",
"description": "The workflow ID"
},
"external_workflow_revision_id": {
"type": "string",
"description": "The external workflow revision ID"
},
"created_at": {
"type": "string",
"description": "The workflow version creation timestamp"
}
}
},
"triggers": {
"type": "array"
},
"actions": {
"type": "array"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `400`"
],
"title: `Invalid Attribute`",
"detail: `Email Already Taken.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `401`"
],
"title: `Unauthorized`",
"detail: `Unauthenticated.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `422`"
],
"title: `Unprocessable Content`",
"detail: `The triggers field is required.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Create a workflowPOST/workflows
Body parameters
Name | Type | Description |
---|---|---|
name | string | Required Between 1 and 255 characters in length |
published_at | string | Required ISO 8601 date. |
expires_at | string | Optional ISO 8601 date. |
targeted_flag | integer | Required (0,1,2) 0 - available for everyone, 1 - targeted customers, 2 - available for tier customers |
audience_filters | array | Required when targeted_flag is 1 . |
tiers | array | Required when targeted_flag is 2 . |
tiers.* | integer | Tier level id |
skip_trigger_validation | boolean | Optional When set to true, validate if the trigger is set up for other workflows. When set to false, skip the validation. |
triggers | array | Required |
triggers.*.main_trigger_id | integer | Required |
triggers.*.frequency | array | Optional |
triggers.*.frequency.freq | string | Required when frequency is present In: hourly,daily,weekly,monthly,yearly,flow |
triggers.*.frequency.interval | integer | Optional Default 1. The trigger needs to occur in X hours/days/months/years. |
triggers.*.frequency.count | Optional Default 1. How many times does the trigger need to occur. |
|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required when the condition is present . |
triggers..conditions..operator | string | Required when the condition is present . In: eq,gt,gte,lt,lte,in,between,intersect,contains. The operator depends on the condition ID. |
triggers..conditions..value | Mixed | Required when the condition is present . Could be string, integer, array, depends on the operator. |
actions | array | Required |
actions.*.main_action_id | integer | Required |
actions.*.name | string | Optional Between 1 and 255 characters in length |
actions.*.action_key | string | Optional The action_key name should be between 1 and 255 characters in length and will be used as the DSL step name. It must be unique among actions. |
actions.*.enabled | boolean | Optional Default: true. |
actions.*.temp_id | integer | Required |
actions.*.temp_parent_id | integer | Required Default: null. The parent action temp id. |
actions.*.frequency | array | Optional |
actions.*.frequency.freq | string | Required when frequency is present In: hourly,daily,weekly,monthly,yearly,flow |
actions.*.frequency.interval | integer | Optional Default 1. The maximum number of times the action will be executed in X hours/days/months/years. |
actions.*.frequency.count | Optional Default 1. The maximum number of times the action will be executed. |
|
actions.*.relations | array | Optional |
actions..relations..entity_id | integer | Required when relation is present Nullable |
actions..relations..entity_type | string | Required when relation is present |
actions..relations..value | string | Optional Depends on the entity_type, sometimes it’s required. |
actions..relations..label | string | Optional |
actions.*.conditions | array | Optional |
actions..conditions..id | integer | Required when the condition is present . |
actions..conditions..main_trigger_id | integer | Optional . If there is multiple triggers, the condition should be for the specific type of trigger, by default is the first trigger’s main_trigger_id |
actions..conditions..operator | string | Required when the condition is present . In: eq,gt,gte,lt,lte,in,between,intersect,contains. The operator depends on the condition ID. |
actions..conditions..value | Mixed | Required when the condition is present . Could be string, integer, array, depends on the operator. |
actions.*.conditions_met | boolean | Required when the action is the child of an conditional split action |
Body parameters specific for branch condition All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 1 |
triggers..conditions..operator | string | Required . In: eq,in. |
triggers..conditions..value | array or string | Required Branch uuid or array of branch uuid. |
Body parameters specific for specific day of the week All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 2 |
triggers..conditions..operator | string | Required . In: eq,in. |
triggers..conditions..value | array or integer | Required Day of the week, in: 1 - Monday, 2 - Tuesday … 7 - Sunday |
Body parameters specific for specific time of the week All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 3 |
triggers..conditions..operator | string | Required . “between”. |
triggers..conditions..value | array | Required H:i time format, ex: [“08:00”, “17:00”] |
Body parameters specific for amount All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 4 |
triggers..conditions..operator | string | Required . In: eq, gt, gte, lt, lte, between. |
triggers..conditions..value | array or numeric | Required |
Body parameters specific for referral status All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 5 |
triggers..conditions..operator | string | Required . In: eq,in. |
triggers..conditions..value | array or numeric | Required 1 - Referral Pending. 2 - Referral condition met. 3 - Referral condition not met |
Body parameters specific for specific pos products or categories All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 6 - specific products, 7 - specific categories |
triggers..conditions..operator | string | Required . In: intersect(one of products/categories in condition.value), contains(all the products/categories in condition.value). |
triggers..conditions..value | array | Required |
triggers..conditions..value.integration_id | string | Required |
triggers..conditions..value.account_id | string | Required |
triggers..conditions..value.resource_id | array | Required array of product/category id. |
Body parameters specific for completed profile All common +
Name | Type | Description |
---|---|---|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required 8 |
triggers..conditions..operator | string | Required . “contains” |
triggers..conditions..value | array | Required . An array of integers representing the index of CRM settings’ fields that require completion. |
Example create a workflow for user sign up
POST /workflows HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"name": "User sign up",
"published_at": "2024-03-10T01:01:01+00:00",
"expires_at": "2024-12-10T01:01:01+00:00",
"targeted_flag": 2,
"tiers": [
29
],
"triggers": [
{
"main_trigger_id": 1,
"frequency": {
"freq": "flow",
"count": 1
},
"conditions": [
{
"id": 1,
"operator": "in",
"value": [
"11ea6d322b6dd08daf33b42e99312acc",
"11ea6d3e304d0189af33b42e99312acc"
]
}
]
}
],
"actions": [
{
"main_action_id": 3,
"temp_id": 1,
"parent_id": null,
"temp_parent_id": null,
"relations": [
{
"entity_id": null,
"label": "point",
"entity_type": "points",
"value": [
100
]
}
]
}
]
}
Example create a workflow for user sign up with conditional split. If the user signup, if it’s from a specific branch and on Monday, Tuesday, user will get 100pts, otherwise he gets 10pts.
POST /workflows HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"expires_at": "2024-12-10T01:01:01+00:00",
"name": "User sign up",
"published_at": "2024-04-22T01:01:01+00:00",
"targeted_flag": 0,
"triggers":
[
{
"main_trigger_id": 1
}
],
"actions":
[
{
"conditions":
[
{
"id": 1,
"operator": "eq",
"value": "11eb82ae6d3f1ec4b847e2865c66d9cc"
},
{
"id": 2,
"operator": "in",
"value":
[
1,
2
]
}
],
"main_action_id": 6,
"temp_id": 1,
"temp_parent_id": null
},
{
"conditions_met": 1,
"main_action_id": 3,
"relations":
[
{
"entity_type": "points",
"label": "points",
"value": 100
}
],
"temp_id": 2,
"temp_parent_id": 1
},
{
"conditions_met": false,
"main_action_id": 3,
"relations":
[
{
"entity_type": "points",
"label": "points",
"value": 10
}
],
"temp_id": 3,
"temp_parent_id": 1
}
]
}
Workflow Resource ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"targeted_flag": 0,
"triggers": [
{
"main_trigger_id": 3,
"frequency": {
"freq": "flow",
"count": 2
},
"conditions": [
{
"id": 8,
"operator": "contains",
"value": "["
}
]
}
],
"actions": [
{
"id": 35,
"action_key: `Reward_Points_17072356685213_45745`": "",
"frequency": {
"freq": "flow",
"count": 2
},
"parent_id": 1,
"temp_parent_id": 2,
"relations": [
{
"entity_id": "null",
"value": 10,
"label": "point",
"entity_type": "points"
}
],
"main_action_id": 4,
"action_key: `Wait_17072356685358_92300`": "",
"temp_id": 49,
"enabled": false
}
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 14,
"name": "Completed profile",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"expires_at": "2028-12-14T01:01:01+00:00",
"workflow_version": {
"id": 47,
"workflow_id": 14,
"external_workflow_revision_id": "000017-5a5",
"created_at": "2024-02-20T01:37:52.000000Z"
},
"triggers": [
{
"id": 29,
"main_trigger_id": 3,
"created_at": "2024-02-20T01:14:40+00:00"
}
],
"actions": [
{
"action_key: `Reward_Points_17072356685213_45745`": "",
"id": 61,
"main_action_id": 4,
"name": "null",
"action_key: `Wait_17072356685358_92300`": "",
"parent_id": "null",
"created_at": "2024-02-20T01:37:52+00:00",
"conditions": "null",
"relations": "null"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The ID"
},
"name": {
"type": "string",
"description": "The name\\"
},
"created_at": {
"type": "string",
"description": "The creation timestamp"
},
"published_at": {
"type": "string",
"description": "The publication timestamp"
},
"expires_at": {
"type": "string",
"description": "The expiration timestamp"
},
"workflow_version": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The workflow version ID"
},
"workflow_id": {
"type": "number",
"description": "The workflow ID"
},
"external_workflow_revision_id": {
"type": "string",
"description": "The external workflow revision ID"
},
"created_at": {
"type": "string",
"description": "The workflow version creation timestamp"
}
}
},
"triggers": {
"type": "array"
},
"actions": {
"type": "array"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `400`"
],
"title: `Invalid Attribute`",
"detail: `Email Already Taken.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `401`"
],
"title: `Unauthorized`",
"detail: `Unauthenticated.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `403`"
],
"title: `Unauthorized`",
"detail: `Unauthorized to perform this action.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `422`"
],
"title: `Unprocessable Content`",
"detail: `The triggers field is required.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Update a workflowPATCH/workflows/{id}
Body parameters
Name | Type | Description |
---|---|---|
name | string | Optional Between 1 and 255 characters in length |
published_at | string | Optional ISO 8601 date. Can not be updated if the workflow is published |
expires_at | string | Optional ISO 8601 date. |
targeted_flag | integer | Required (0,1,2) 0 - available for everyone, 1 - targeted customers, 2 - available for tier customers |
audience_filters | object | Required when targeted_flag is 1 . |
tiers | array | Required when targeted_flag is 2 . |
tiers.* | integer | Tier level id |
triggers | array | Required |
triggers.*.main_trigger_id | integer | Required |
triggers.*.enabled | boolean | Optional Default: true |
triggers.*.frequency | array | Optional |
triggers.*.frequency.freq | string | Required when frequency is present In: hourly,daily,weekly,monthly,yearly,flow |
triggers.*.frequency.interval | integer | Optional Default 1. The trigger needs to occur in X hours/days/months/years. |
triggers.*.frequency.count | Optional Default 1. How many times does the trigger need to occur. |
|
triggers.*.conditions | array | Optional |
triggers..conditions..id | integer | Required when the condition is present . |
triggers..conditions..operator | string | Required when the condition is present . In: eq,gt,gte,lt,lte,in,between,intersect,contains. The operator depends on the condition ID. |
triggers..conditions..value | Mixed | Required when the condition is present . Could be string, integer, array, depends on the operator. |
actions | array | Required |
actions.*.main_action_id | integer | Required |
actions.*.enabled | boolean | Optional Default: true |
actions.*.name | string | Optional Between 1 and 255 characters in length |
actions.*.action_key | string | Optional The action_key name should be between 1 and 255 characters in length and will be used as the DSL step name. It must be unique among actions. |
actions.*.enabled | boolean | Optional Default: true. |
actions.*.temp_id | integer | Required |
actions.*.temp_parent_id | integer | Required Default: null. The parent action temp id. |
actions.*.id | integer | Optional |
actions.*.parent_id | integer | Optional Default: null. The parent action id. |
actions.*.frequency | array | Optional |
actions.*.frequency.freq | string | Required when frequency is present In: hourly,daily,weekly,monthly,yearly,flow |
actions.*.frequency.interval | integer | Optional Default 1. The maximum number of times the action will be executed in X hours/days/months/years. |
actions.*.frequency.count | Optional Default 1. The maximum number of times the action will be executed. |
|
actions.*.relations | array | Optional |
actions..relations..entity_id | integer | Required when relation is present Nullable |
actions..relations..entity_type | string | Required when relation is present |
actions..relations..value | string | Optional Depends on the entity_type, sometimes it’s required. |
actions..relations..label | string | Optional |
Example update a workflow
PATCH /workflows/10 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
{
"targeted_flag": 0,
"triggers": [
{
"main_trigger_id": 3,
"frequency": {
"freq": "flow",
"count": 2
},
"conditions": [
{
"id": 8,
"operator": "contains",
"value": [-2,0,1]
}
]
}
],
"actions": [
{
"main_action_id": 3,
"id": 35,
"action_key": "Reward_Points_17072356685213_45745",
"frequency": {
"freq": "flow",
"count": 2
},
"parent_id": null,
"temp_parent_id": null,
"relations": [
{
"entity_id": null,
"value": 10,
"label": "point",
"entity_type": "points"
}
]
},
{
"main_action_id": 4,
"action_key": "Wait_17072356685358_92300",
"temp_id": 49,
"enabled": false
}
]
}
- id
number
(required) Example: 123The unique ID of the workflow.
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `400`"
],
"title: `Invalid Attribute`",
"detail: `Email Already Taken.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"errors": [
[
"status: `403`"
],
"title: `Unauthorized`",
"detail: `Unauthorized to perform this action.`"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"errors": {
"type": "array"
}
}
}
Delete a workflowDELETE/workflows/{id}
Example Delete follow facebook action
DELETE /workflows/10 HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
- id
number
(required) Example: 123The unique ID of the workflow.
Reviews ¶
Reviews ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "61808119-7567-41c5-a183-389bf9819ccb",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 5,
"title": "Good",
"comment": "Great leggings. For size reference, I ordered a medium and am 5’9, 155 lbs, with a long torso.",
"product_id": "09383628200",
"product_title": "null",
"photos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"videos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"type": 1,
"review_source": 1,
"published": false,
"verified_buyer": false,
"review_replies": [
{
"id": "36f04d79-4116-4fb3-8d08-50581be3fa67",
"created_at": "2024-07-11T15:55:03+00:00",
"body": "Reply content",
"public": true
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
List all reviewsGET/reviews
Returns a list of reviews
Query Parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15, Maximum value 100 |
include | string | Optional. Optionally include=review_replies. |
created_at | string | Optional. Filter by created date. See Filtering |
type | integer | Optional. Filter by review type, 1 - product review, 2 - website review. See Filtering |
published | boolean | Optional. Filter by published. See Filtering |
sort | string | Optional. See Sorting |
Filtering
When submitting a GET request, you can ask it to return only reviews that meet specific criteria.
To filter reviews, in the query string, specify one or more fields with an operator, followed by a separator (|
)
and the values for the following:
Fields:
-
created_at
- Filter by created date -
type
- Filter by review type. 1 - Product, 2 - Website -
published
- Filter by published status
Operators:
-
gt
- Greater Than -
gte
- Greater Than or Equal -
lt
- Less Than -
lte
- Less Than or Equal -
eq
- Equal -
between
- Between
Filter Syntax
A single filter uses the form: field=operator|VALUE
Each URL query parameter must be URL encoded.
Sorting
When submitting a GET request, you can sort the reviews.
To sort reviews, in the query string, specify one or more fields with an operator, followed by a separator (,
)
and the fields for the following:
Fields:
-
created_at
- Sort by created date. Ifcreated_at
sorting is not specified, the results will always be sorted by the latest combination with other sorting conditions. -
rating
- Sort by rating -
photos
- Sort reviews by photos, displaying reviews with photos first or last. -
videos
- Sort reviews by videos, displaying reviews with videos first or last. -
verified_buyer
- Sort reviews by verified buyer, displaying reviews with verified buyer review first or last.
Operators:
-
By default without any operator, sort reviews in ascending order.
-
-
- Sort reviews in descending order.
Filter Syntax
A single filter uses the form: sort=operatorFieldName1,operatorFieldName2
Each URL query parameter must be URL encoded.
Examples
GET /reviews?sort=-rating,-created_at&include=review_replies HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImFmODNk
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
Response Body
{
"data": [
{
"id": "61808119-7567-41c5-a183-389bf9819ccb",
"created_at": "2024-06-26T23:13:08+00:00",
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 5,
"title": null,
"comment": "Great leggings. For size reference, I ordered a medium and am 5’9, 155 lbs, with a long torso.",
"product_id": "09383628200",
"product_title": null,
"photos": null,
"videos": null,
"type": 1,
"review_source": 1,
"published": false,
"verified_buyer": false,
"review_replies": [
{
"id": "36f04d79-4116-4fb3-8d08-50581be3fa67",
"created_at": "2024-07-11T15:55:03+00:00",
"body": "Reply - 01",
"public": true
}
]
}
]
}
Review ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 5,
"title": "Good",
"comment": "Very nice and cute.",
"product_id": "5157526",
"product_title": "Shoes",
"product_details": {
"image": "https://product/1234.png",
"price": 26.99,
"description": "1234"
},
"photos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"videos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"type": 1,
"review_source": 1,
"website_url": "https://product/1234.com"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"reviewer_email": {
"type": "string",
"description": "reviewer's email"
},
"reviewer_first_name": {
"type": "string",
"description": "reviewer's first name"
},
"rating": {
"type": "number"
},
"title": {
"type": "string",
"description": "review title"
},
"comment": {
"type": "string",
"description": "review"
},
"product_id": {
"type": "string",
"description": "product id in the integration system"
},
"product_title": {
"type": "string",
"description": "product title"
},
"product_details": {
"type": "object",
"properties": {
"image": {
"type": "string",
"description": "product image url"
},
"price": {
"type": "number"
},
"description": {
"type": "string",
"description": "product description"
}
}
},
"photos": {
"type": "array"
},
"videos": {
"type": "array"
},
"type": {
"type": "number"
},
"review_source": {
"type": "number"
},
"website_url": {
"type": "string"
}
}
}
Headers
Content-Type: No Content
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"reviewer_email": [
"The reviewer email field is required."
],
"reviewer_first_name": [
"The reviewer first name field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"reviewer_email": {
"type": "array"
},
"reviewer_first_name": {
"type": "array"
}
}
}
Create a reviewPOST/reviews
Body parameters
Name | Type | Description |
---|---|---|
reviewer_email | string | Required Between 1 and 255 characters in length |
reviewer_first_name | string | Required Between 1 and 255 characters in length |
rating | integer | Required Between 1 and 5 |
title | string | Optional |
comment | string | Required Minimum 15 characters |
product_id | string | Optional Required if the type is 1 - product review |
product_title | string | Optional |
product_details.image | string | Optional product image URL |
product_details.price | numeric | Optional product price |
product_details.description | string | Optional product description |
photos | array | Optional |
photos.*.path | string | Optional review photo url See Uploading Photos |
videos | array | Optional |
videos.*.path | string | Optional review video url See Uploading Videos |
type | integer | Required (1, 2) 1: product review, 2: website review |
review_source | integer | Required (1, 2) The platform where the review was left. 1: website page, 2: review widget |
website_url | string | Required The URL of the website where the review was left. |
Example create a review
POST /reviews HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 4,
"title": "Good",
"comment": "Very nice and cute",
"type": 1,
"review_source": 1,
"website_url": "https://example.com/product/1234",
"product_id": "5157526",
"product_title": "Shoes",
"product_details": {
"image": "https://example.com/1234.png",
"price": 59.99,
"description": "ABC"
},
"photos": [
{
"path": "https://storage.googleapis.com/reviews/11eb82ae6d3bb094b847e2865c66d9cc/668ee22b0db7e_1720640043.jpg?X-Goog-Algorithm=GOOG4-RSA-SHA256&X-Goog-Credential=bigquery-for-dev%40kangaroo-dev-5223a.iam.gserviceaccount.com%2F20240710%2Fauto%2Fstorage%2Fgoog4_request&X-Goog-Date=20240710T193403Z&X-Goog-Expires=900&X-Goog-SignedHeaders=content-type%3Bhost&X-Goog-Signature=8593d255c54cc1341aa4f7f90b12c18d085fb84cbd4631f173eca4033a91ad43e131ec04cb3ab7502156bcdc23dcd69d2dd91eb1a437a5697113f0ccdb9dbe62d8cb99913a067f5e2dad2b56ac5351a1fede30e67609d19a83e403c3c6222659c99bca3dc7d9c1c02cbeb2d0c872352d73be465cca3a6bb31fde7d4e711be248c933291ca36a9353c3fe2e7a3bc56dd6e8d2eca93b8dfbbd480c524e05f25e93f1763a4ab0db4f86497fe631407db63137bf8ee2cc81e8de1f93f5aafb389ebd1dd19cadac05731b0b8c66cf00c22180e8bbc33199f5d57654a127178111921d45b6b98642332b2188825aabad3ced41d0d33ba25c7571b7836fd4a210f8892d"
}
],
"videos": [
{
"path": "https://storage.googleapis.com/reviews/11eb82ae6d3bb094b847e2865c66d9cc/668ee22ba162b_1720640043.mkv?X-Goog-Algorithm=GOOG4-RSA-SHA256&X-Goog-Credential=bigquery-for-dev%40kangaroo-dev-5223a.iam.gserviceaccount.com%2F20240710%2Fauto%2Fstorage%2Fgoog4_request&X-Goog-Date=20240710T193403Z&X-Goog-Expires=900&X-Goog-SignedHeaders=content-type%3Bhost&X-Goog-Signature=7e670a4694dde5fccab7200dd7134538a6af74b15a49095493583f9750e53f04979f46b950b305bc2d12c1370609f2d246c883c283a9c68edc0a474231dc0a5c25bbf3d38a8b54a200f35e8cd4ab8e34e46b457f153bec30cd1c0df9c5dc00f49a6c11e81cd8b7d08a96d685ab100fac846f92d7363ddf9c513c04c62f42cfde1048ace3263fb87f47afcf2efe0273682bc279edf629531f64b794b894783ad63bb7f0f28ebea3c1e83fe83d47a659614e2eab22d7f0121fedd43f9b6c84f5a0ec9d597f87aeab75a9585e7624d57abbe9b960150f4413d430b51cb62363138639263cf1a13fc88a72f06163c6cfa0c0e497804e5ca29e31843600affc751a6c"
}
]
}
Review ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"published": true
}
Headers
Content-Type: not content
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"published": [
"The published field must be true or false."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"published": {
"type": "array"
}
}
}
Update a reviewPATCH/reviews/{id}
Body parameters
Name | Type | Description |
---|---|---|
published | boolean | Required. Publish or unpublish the review |
Example publish a review
PATCH /reviews/61808119-7567-41c5-a183-389bf9819ccb HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"published": true,
}
- id
string
(required) Example: 61808119-7567-41c5-a183-389bf9819ccbThe unique ID of the review.
Upload images and videos ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"file_types": [
"image/jpeg",
"image/png"
]
}
Headers
Content-Type: application/json
Body
{
"data": [
{
"file_type": "image/jpeg",
"url": "https://storage.googleapis.com/reviews/11eb82ae6d3bb094b847e2865c66d9cc/668ee22ba162b_1720640043.mkv?X-Goog-Algorithm=GOOG4-RSA-SHA256&X-Goog-Credential=bigquery-for-dev%40kangaroo-dev-5223a.iam.gserviceaccount.com%2F20240710%2Fauto%2Fstorage%2Fgoog4_request&X-Goog-Date=20240710T193403Z&X-Goog-Expires=900&X-Goog-SignedHeaders=content-type%3Bhost&X-Goog-Signature=7e670a4694dde5fccab7200dd7134538a6af74b15a49095493583f9750e53f04979f46b950b305bc2d12c1370609f2d246c883c283a9c68edc0a474231dc0a5c25bbf3d38a8b54a200f35e8cd4ab8e34e46b457f153bec30cd1c0df9c5dc00f49a6c11e81cd8b7d08a96d685ab100fac846f92d7363ddf9c513c04c62f42cfde1048ace3263fb87f47afcf2efe0273682bc279edf629531f64b794b894783ad63bb7f0f28ebea3c1e83fe83d47a659614e2eab22d7f0121fedd43f9b6c84f5a0ec9d597f87aeab75a9585e7624d57abbe9b960150f4413d430b51cb62363138639263cf1a13fc88a72f06163c6cfa0c0e497804e5ca29e31843600affc751a6c"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"file_types": [
"The file types field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"file_types": {
"type": "array"
}
}
}
Get signed urlsPOST/reviews/signed-urls
Body parameters
Name | Type | Description |
---|---|---|
file_types | array | required |
file_types.* | string | required One of image/jpeg,image/png,image/jpg,image/gif,video/mp4,video/mkv,video/mov,video/avi,video/flv |
Note: To upload images or videos:
- Make a request to get the signed url(see above).
- After getting back the url from the response, upload the file directly to this url. Method: ‘PUT’, with header “content-type:application/octet-stream”, body: the image/video file.
Example get a signed url for uploading images and videos
POST /reviews/signed-urls HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"file_types": [
"image/jpeg",
"video/mkv"
]
}
Response Body
{
"data": [
{
"file_type": "image/jpeg",
"url": "https://storage.googleapis.com/reviews/11eb82ae6d3bb094b847e2865c66d9cc/668ee22b0db7e_1720640043.jpg?X-Goog-Algorithm=GOOG4-RSA-SHA256&X-Goog-Credential=bigquery-for-dev%40kangaroo-dev-5223a.iam.gserviceaccount.com%2F20240710%2Fauto%2Fstorage%2Fgoog4_request&X-Goog-Date=20240710T193403Z&X-Goog-Expires=900&X-Goog-SignedHeaders=content-type%3Bhost&X-Goog-Signature=8593d255c54cc1341aa4f7f90b12c18d085fb84cbd4631f173eca4033a91ad43e131ec04cb3ab7502156bcdc23dcd69d2dd91eb1a437a5697113f0ccdb9dbe62d8cb99913a067f5e2dad2b56ac5351a1fede30e67609d19a83e403c3c6222659c99bca3dc7d9c1c02cbeb2d0c872352d73be465cca3a6bb31fde7d4e711be248c933291ca36a9353c3fe2e7a3bc56dd6e8d2eca93b8dfbbd480c524e05f25e93f1763a4ab0db4f86497fe631407db63137bf8ee2cc81e8de1f93f5aafb389ebd1dd19cadac05731b0b8c66cf00c22180e8bbc33199f5d57654a127178111921d45b6b98642332b2188825aabad3ced41d0d33ba25c7571b7836fd4a210f8892d"
},
{
"file_type": "video/mkv",
"url": "https://storage.googleapis.com/reviews/11eb82ae6d3bb094b847e2865c66d9cc/668ee22ba162b_1720640043.mkv?X-Goog-Algorithm=GOOG4-RSA-SHA256&X-Goog-Credential=bigquery-for-dev%40kangaroo-dev-5223a.iam.gserviceaccount.com%2F20240710%2Fauto%2Fstorage%2Fgoog4_request&X-Goog-Date=20240710T193403Z&X-Goog-Expires=900&X-Goog-SignedHeaders=content-type%3Bhost&X-Goog-Signature=7e670a4694dde5fccab7200dd7134538a6af74b15a49095493583f9750e53f04979f46b950b305bc2d12c1370609f2d246c883c283a9c68edc0a474231dc0a5c25bbf3d38a8b54a200f35e8cd4ab8e34e46b457f153bec30cd1c0df9c5dc00f49a6c11e81cd8b7d08a96d685ab100fac846f92d7363ddf9c513c04c62f42cfde1048ace3263fb87f47afcf2efe0273682bc279edf629531f64b794b894783ad63bb7f0f28ebea3c1e83fe83d47a659614e2eab22d7f0121fedd43f9b6c84f5a0ec9d597f87aeab75a9585e7624d57abbe9b960150f4413d430b51cb62363138639263cf1a13fc88a72f06163c6cfa0c0e497804e5ca29e31843600affc751a6c"
}
]
}
List reviews for the website ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "61808119-7567-41c5-a183-389bf9819ccb",
"created_at": "2024-02-06T16:07:52+00:00",
"published_at": "2024-01-10T01:01:01+00:00",
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 5,
"title": "Good",
"comment": "Great leggings. For size reference, I ordered a medium and am 5’9, 155 lbs, with a long torso.",
"product_id": "09383628200",
"product_title": "null",
"photos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"videos": [
{
"path": "https://storage.googleapis.com/reviews/11exyz"
}
],
"type": 1,
"review_source": 1,
"published": false,
"verified_buyer": false,
"review_replies": [
{
"id": "36f04d79-4116-4fb3-8d08-50581be3fa67",
"created_at": "2024-07-11T15:55:03+00:00",
"body": "Reply content",
"public": true
}
]
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
List published reviewsGET/bff/web/published-reviews
The reviews displayed on the website are filtered and configured by the review app’s settings. This endpoint only returns reviews that match those settings, and the results are sorted accordingly.
Body parameters
Name | Type | Description |
---|---|---|
page | integer | Optional. Provide this to retrieve the next set of results for your original query. Default value page=1 |
per_page | integer | Optional. Provide this to set the number of items in the page. Default value 15, Maximum value 100 |
include | string | Optional. Optionally include=review_replies. |
Example
GET /bff/web/published-reviews?include=review_replies HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
Response Body
{
"data": [
{
"id": "61808119-7567-41c5-a183-389bf9819ccb",
"created_at": "2024-06-26T23:13:08+00:00",
"reviewer_email": "lisa@example.com",
"reviewer_first_name": "Lisa",
"rating": 5,
"title": null,
"comment": "Great leggings. For size reference, I ordered a medium and am 5’9, 155 lbs, with a long torso.",
"product_id": "09383628200",
"product_title": null,
"photos": null,
"videos": null,
"type": 1,
"review_source": 1,
"published": false,
"verified_buyer": false,
"review_replies": [
{
"id": "36f04d79-4116-4fb3-8d08-50581be3fa67",
"created_at": "2024-07-11T15:55:03+00:00",
"body": "Reply - 01",
"public": true
}
]
}
]
}
Review Replies ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"body": "Thanks for your feedback.",
"public": true
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"body": {
"type": "string",
"description": "The reply content"
},
"public": {
"type": "boolean",
"description": "public or private reply"
}
}
}
Headers
Content-Type: No Content
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"body": "The review body field is required.",
"public": "`The public field is required`."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"body": {
"type": "string"
},
"public": {
"type": "string"
}
}
}
Create a review replyPOST/reviews/replies
Body parameters
Name | Type | Description |
---|---|---|
body | string | Required Reply content |
public | boolean | Required If it’s a public reply, it can be listed in the website. If it’s a private reply, the reply will be sent to the customer by email. |
Example create a reply
POST /reviews/2a6c99d6-195c-47a1-86ee-9b3f5ae8bdaf/replies HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"body": "Thanks for your feedback.",
"public": true
}
Reviews app settings ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Headers
Content-Type: application/json
Body
{
"data": {
"id": 1,
"created_at": "2024-06-11T18:40:53+00:00",
"updated_at": "2024-06-21T20:06:21+00:00",
"coupon_auto": true,
"coupon_min_rating": 1,
"coupon_user_segment": 0,
"coupon_min_media": 1,
"coupon_min_photos": 1,
"coupon_min_videos": 1,
"coupon_min_chars": 1,
"coupon_min_title_chars": 1,
"coupon_type": 3,
"coupon_value": 10,
"coupon_prefix": "KR",
"widget_primary_color": "#111111",
"widget_position": "bottom-left",
"widget_published": true,
"allow_photos": true,
"allow_videos": true,
"mute_videos": true,
"carousel_published": true,
"carousel_slides": 3,
"carousel_max_slides": 3,
"carousel_sort": 5,
"carousel_scroll": 1,
"review_auto_publish": true,
"review_auto_publish_min_rating": 4,
"review_auto_request": true,
"review_auto_request_days": 10,
"review_auto_reminder": true,
"review_auto_reminder_days": 12,
"review_max_products\"": 10,
"review_products_option": 0,
"display_customer_name": true,
"display_customer_email": true,
"display_verified_buyer": true,
"word_blocks": [
"keyword1",
"keyword2"
],
"community_enabled": false,
"community_reply_permission": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The id of review app settings"
},
"created_at": {
"type": "string"
},
"updated_at": {
"type": "string"
},
"coupon_auto": {
"type": "boolean",
"description": "Auto send out the coupon by email to the reviewers"
},
"coupon_min_rating": {
"type": "number",
"description": "To be eligible for a coupon, the minimum review rating required"
},
"coupon_user_segment": {
"type": "number",
"description": "0: Any reviewer can be eligible for a coupon, 1: only verified buyer can be eligible for a coupon"
},
"coupon_min_media": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review photos and videos is required in the review."
},
"coupon_min_photos": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review photos is required in the review."
},
"coupon_min_videos": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review videos is required in the review."
},
"coupon_min_chars": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of characters is required in the review."
},
"coupon_min_title_chars": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of characters is required in the review title."
},
"coupon_type": {
"type": "number",
"description": "Coupon type:1 - Amount; 2 - Percentage; 3 - FreeShipping"
},
"coupon_value": {
"type": "number"
},
"coupon_prefix": {
"type": "string"
},
"widget_primary_color": {
"type": "string"
},
"widget_position": {
"type": "string",
"description": "`bottom-right`, `bottom-left`, `top-right`, `top-left`, `left-side-top`, `left-side-center`, `left-side-bottom`, `right-side-top`, `right-side-center`, `right-side-bottom`"
},
"widget_published": {
"type": "boolean"
},
"allow_photos": {
"type": "boolean",
"description": "Allow the reviewer to upload the photos"
},
"allow_videos": {
"type": "boolean",
"description": "Allow the reviewer to upload the videos"
},
"mute_videos": {
"type": "boolean",
"description": "Mute the video by default when it is played"
},
"carousel_published": {
"type": "boolean"
},
"carousel_slides": {
"type": "number",
"description": "1,2,3"
},
"carousel_max_slides": {
"type": "number",
"description": "2,3"
},
"carousel_sort": {
"type": "number",
"description": "1-newest first, 2-highest rated first, 3-photos first, 4-videos first, 5-verified buyers first"
},
"carousel_scroll": {
"type": "number",
"description": "1-one slide,2-scroll freely"
},
"review_auto_publish": {
"type": "boolean"
},
"review_auto_publish_min_rating": {
"type": "number",
"description": "The minimum rating of auto publishing a review"
},
"review_auto_request": {
"type": "boolean",
"description": "Auto send out review request email"
},
"review_auto_request_days": {
"type": "number",
"description": "The number of days for auto send out review request email"
},
"review_auto_reminder": {
"type": "boolean",
"description": "Auto send out review reminder email"
},
"review_auto_reminder_days": {
"type": "number",
"description": "The number of days for auto send out reminder email"
},
"review_max_products\"": {
"type": "number",
"description": "The maximum number of products in the review request/reminder email"
},
"review_products_option": {
"type": "number",
"description": "The option for listing the product in the review request/reminder email"
},
"display_customer_name": {
"type": "boolean",
"description": "Display the reviewers name to the website"
},
"display_customer_email": {
"type": "boolean",
"description": "Display the reviewers email to the website"
},
"display_verified_buyer": {
"type": "boolean",
"description": "Display the verified buyer badge to the website"
},
"word_blocks": {
"type": "array",
"description": "A list of words that will automatically cause a review to be unpublished if any of them are included in the review"
},
"community_enabled": {
"type": "boolean",
"description": "Display the verified buyer badge to the website"
},
"community_reply_permission": {
"type": "number",
"description": "Who can reply to the questions. 1: business employee, 2: business employee + verified buyer"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
List reviews app settingsGET/review-app-settings
Example
GET /review-app-settings HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
Response Body
{
"data": {
"id": 1,
"created_at": "2024-06-11T18:40:53+00:00",
"updated_at": "2024-06-21T20:06:21+00:00",
"coupon_auto": true,
"coupon_min_rating": null,
"coupon_user_segment": 0,
"coupon_min_media": null,
"coupon_min_photos": null,
"coupon_min_videos": null,
"coupon_min_chars": null,
"coupon_min_title_chars": null,
"coupon_type": 3,
"coupon_value": 12.15,
"coupon_prefix": null,
"widget_primary_color": "#111111",
"widget_position": "bottom-left",
"widget_published": true,
"allow_photos": true,
"allow_videos": true,
"mute_videos": true,
"carousel_published": true,
"carousel_slides": 3,
"carousel_max_slides": 2,
"carousel_sort": 5,
"carousel_scroll": 1,
"review_auto_publish": true,
"review_auto_publish_min_rating": 4,
"review_auto_request": true,
"review_auto_request_days": 10,
"review_auto_reminder": true,
"review_auto_reminder_days": 12,
"review_max_products": 10,
"review_products_option": 0,
"display_customer_name": true,
"display_customer_email": true,
"display_verified_buyer": true,
"word_blocks": [],
"community_enabled": false,
"community_reply_permission": 1
}
}
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Headers
Content-Type: application/json
Body
{
"data": {
"id": 1,
"created_at": "2024-06-11T18:40:53+00:00",
"updated_at": "2024-06-21T20:06:21+00:00",
"coupon_auto": true,
"coupon_min_rating": 1,
"coupon_user_segment": 0,
"coupon_min_media": 1,
"coupon_min_photos": 1,
"coupon_min_videos": 1,
"coupon_min_chars": 1,
"coupon_min_title_chars": 1,
"coupon_type": 3,
"coupon_value": 10,
"coupon_prefix": "KR",
"widget_primary_color": "#111111",
"widget_position": "bottom-left",
"widget_published": true,
"allow_photos": true,
"allow_videos": true,
"mute_videos": true,
"carousel_published": true,
"carousel_slides": 3,
"carousel_max_slides": 3,
"carousel_sort": 5,
"carousel_scroll": 1,
"review_auto_publish": true,
"review_auto_publish_min_rating": 4,
"review_auto_request": true,
"review_auto_request_days": 10,
"review_auto_reminder": true,
"review_auto_reminder_days": 12,
"review_max_products\"": 10,
"review_products_option": 0,
"display_customer_name": true,
"display_customer_email": true,
"display_verified_buyer": true,
"word_blocks": [
"keyword1",
"keyword2"
],
"community_enabled": false,
"community_reply_permission": 1
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number",
"description": "The id of review app settings"
},
"created_at": {
"type": "string"
},
"updated_at": {
"type": "string"
},
"coupon_auto": {
"type": "boolean",
"description": "Auto send out the coupon by email to the reviewers"
},
"coupon_min_rating": {
"type": "number",
"description": "To be eligible for a coupon, the minimum review rating required"
},
"coupon_user_segment": {
"type": "number",
"description": "0: Any reviewer can be eligible for a coupon, 1: only verified buyer can be eligible for a coupon"
},
"coupon_min_media": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review photos and videos is required in the review."
},
"coupon_min_photos": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review photos is required in the review."
},
"coupon_min_videos": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of review videos is required in the review."
},
"coupon_min_chars": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of characters is required in the review."
},
"coupon_min_title_chars": {
"type": "number",
"description": "To be eligible for a coupon, the minimum number of characters is required in the review title."
},
"coupon_type": {
"type": "number",
"description": "Coupon type:1 - Amount; 2 - Percentage; 3 - FreeShipping"
},
"coupon_value": {
"type": "number"
},
"coupon_prefix": {
"type": "string"
},
"widget_primary_color": {
"type": "string"
},
"widget_position": {
"type": "string",
"description": "`bottom-right`, `bottom-left`, `top-right`, `top-left`, `left-side-top`, `left-side-center`, `left-side-bottom`, `right-side-top`, `right-side-center`, `right-side-bottom`"
},
"widget_published": {
"type": "boolean"
},
"allow_photos": {
"type": "boolean",
"description": "Allow the reviewer to upload the photos"
},
"allow_videos": {
"type": "boolean",
"description": "Allow the reviewer to upload the videos"
},
"mute_videos": {
"type": "boolean",
"description": "Mute the video by default when it is played"
},
"carousel_published": {
"type": "boolean"
},
"carousel_slides": {
"type": "number",
"description": "1,2,3"
},
"carousel_max_slides": {
"type": "number",
"description": "2,3"
},
"carousel_sort": {
"type": "number",
"description": "1-newest first, 2-highest rated first, 3-photos first, 4-videos first, 5-verified buyers first"
},
"carousel_scroll": {
"type": "number",
"description": "1-one slide,2-scroll freely"
},
"review_auto_publish": {
"type": "boolean"
},
"review_auto_publish_min_rating": {
"type": "number",
"description": "The minimum rating of auto publishing a review"
},
"review_auto_request": {
"type": "boolean",
"description": "Auto send out review request email"
},
"review_auto_request_days": {
"type": "number",
"description": "The number of days for auto send out review request email"
},
"review_auto_reminder": {
"type": "boolean",
"description": "Auto send out review reminder email"
},
"review_auto_reminder_days": {
"type": "number",
"description": "The number of days for auto send out reminder email"
},
"review_max_products\"": {
"type": "number",
"description": "The maximum number of products in the review request/reminder email"
},
"review_products_option": {
"type": "number",
"description": "The option for listing the product in the review request/reminder email"
},
"display_customer_name": {
"type": "boolean",
"description": "Display the reviewers name to the website"
},
"display_customer_email": {
"type": "boolean",
"description": "Display the reviewers email to the website"
},
"display_verified_buyer": {
"type": "boolean",
"description": "Display the verified buyer badge to the website"
},
"word_blocks": {
"type": "array",
"description": "A list of words that will automatically cause a review to be unpublished if any of them are included in the review"
},
"community_enabled": {
"type": "boolean",
"description": "Display the verified buyer badge to the website"
},
"community_reply_permission": {
"type": "number",
"description": "Who can reply to the questions. 1: business employee, 2: business employee + verified buyer"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"coupon_min_rating": [
"The coupon min rating may not be greater than 5."
],
"carousel_max_slides": [
"The selected carousel max slides is invalid."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"coupon_min_rating": {
"type": "array"
},
"carousel_max_slides": {
"type": "array"
}
}
}
Update reviews app settingsPATCH/review-app-settings
Body parameters
Name | Type | Description |
---|---|---|
coupon_auto | boolean | Optional Auto send out the coupon by email to the reviewers |
coupon_min_rating | integer | Optional To be eligible for a coupon, the minimum review rating required |
coupon_user_segment | integer | Optional Any reviewer can be eligible for a coupon, 1: only verified buyer can be eligible for a coupon |
coupon_min_media | integer | Optional To be eligible for a coupon, the minimum number of videos and photos is required in the review. |
coupon_min_photos | integer | Optional To be eligible for a coupon, the minimum number of review photos is required in the review. |
coupon_min_videos | integer | Optional To be eligible for a coupon, the minimum number of review videos is required in the review. |
coupon_min_title_chars | integer | Optional To be eligible for a coupon, the minimum number of number of characters is required in the review title. |
coupon_min_chars | integer | Optional To be eligible for a coupon, the minimum number of number of characters is required in the review. |
coupon_type | integer | Optional Coupon type:1 - Amount; 2 - Percentage; 3 - FreeShipping |
coupon_value | number | Optional The discount amount |
coupon_prefix | string | Optional |
widget_primary_color | string | Optional |
widget_position | string | Optional One of bottom-left (string) - bottom-right , bottom-left , top-right , top-left , left-side-top , left-side-center , left-side-bottom , right-side-top , right-side-center , right-side-bottom |
widget_published | boolean | Optional |
allow_photos | boolean | Optional Allow the reviewer to upload the photos |
allow_videos | boolean | Optional Allow the reviewer to upload the videos |
mute_videos | boolean | Optional Mute the video by default when it is played. |
carousel_published | boolean | Optional |
carousel_slides | integer | Optional (1,2,3) |
carousel_max_slides | integer | Optional (2,3) |
carousel_sort | integer | Optional 1-newest first, 2-highest rated first, 3-photos first, 4-videos first, 5-verified buyers first |
carousel_scroll | integer | Optional 1-one slide,2-scroll freely |
review_auto_publish | boolean | Optional |
review_auto_publish_min_rating | integer | Optional The minimum rating of auto publishing a review |
review_auto_request | boolean | Optional Auto send out review request email |
review_auto_request_days | integer | Optional The number of days for auto send out review request email |
review_auto_reminder | boolean | Optional Auto send out review reminder email |
review_auto_reminder_days | integer | Optional The number of days for auto send out reminder email |
review_max_products | integer | Optional Maximum: 100. The maximum number of product in the review request/reminder email. |
review_products_option | integer | Optional The option for listing the product in the review request/reminder email. 0: random, 1: least reviewed product, 2: highest priced product, 3:lowest priced product, 4: based on the order |
display_customer_name | boolean | Optional Display the reviewers name to the website |
display_customer_email | boolean | Optional Display the reviewers email to the website |
display_verified_buyer | boolean | Optional Display the verified buyer badge to the website |
word_blocks | array | Optional A list of words that will automatically cause a review to be unpublished if any of them are included in the review |
word_blocks.* | string | Optional |
community_enabled | boolean | Optional Enable community(Q/A) section |
community_reply_permission | integer | Optional (1, 2) Who can reply to the questions 1: business employee, 2: business employee + verified buyer |
Example
PATCH /review-app-settings HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"coupon_auto": true,
"coupon_min_rating": null,
"coupon_user_segment": 0,
"coupon_min_media": null,
"coupon_min_photos": null,
"coupon_min_videos": null,
"coupon_min_chars": null,
"coupon_min_title_chars": null,
"coupon_type": 3,
"coupon_value": 12.15,
"coupon_prefix": null,
"widget_primary_color": "#111111",
"widget_position": "bottom-left",
"widget_published": true,
"allow_photos": true,
"allow_videos": true,
"mute_videos": true,
"carousel_published": true,
"carousel_slides": 3,
"carousel_max_slides": 2,
"carousel_sort": 5,
"carousel_scroll": 1,
"review_auto_publish": true,
"review_auto_publish_min_rating": 4,
"review_auto_request": true,
"review_auto_request_days": 10,
"review_auto_reminder": true,
"review_auto_reminder_days": 12,
"review_max_products": 10,
"review_products_option": 0,
"display_customer_name": true,
"display_customer_email": true,
"display_verified_buyer": true,
"word_blocks": [],
"community_enabled": false,
"community_reply_permission": 1
}
Response Body
{
"data": {
"id": 1,
"created_at": "2024-06-11T18:40:53+00:00",
"updated_at": "2024-06-21T20:06:21+00:00",
"coupon_auto": true,
"coupon_min_rating": null,
"coupon_user_segment": 0,
"coupon_min_media": null,
"coupon_min_photos": null,
"coupon_min_videos": null,
"coupon_min_chars": null,
"coupon_min_title_chars": null,
"coupon_type": 3,
"coupon_value": 12.15,
"coupon_prefix": null,
"widget_primary_color": "#111111",
"widget_position": "bottom-left",
"widget_published": true,
"allow_photos": true,
"allow_videos": true,
"mute_videos": true,
"carousel_published": true,
"carousel_slides": 3,
"carousel_max_slides": 2,
"carousel_sort": 5,
"carousel_scroll": 1,
"review_auto_publish": true,
"review_auto_publish_min_rating": 4,
"review_auto_request": true,
"review_auto_request_days": 10,
"review_auto_reminder": true,
"review_auto_reminder_days": 12,
"review_max_products": 10,
"review_products_option": 0,
"display_customer_name": true,
"display_customer_email": true,
"display_verified_buyer": true,
"word_blocks": [],
"community_enabled": false,
"community_reply_permission": 1
}
}
Questions ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"email": "lisa@example.com",
"first_name": "Lisa",
"body": "What are the key features and benefits of this product?",
"product_id": "5157526",
"type": 1,
"source": 1
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"body": {
"type": "string",
"description": "question"
},
"product_id": {
"type": "string",
"description": "product id in the integration system"
},
"type": {
"type": "number",
"description": "1: product question 2: general question"
},
"source": {
"type": "number",
"description": "1: website 2: widget"
}
}
}
Headers
Content-Type: No Content
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"body": [
"The body field is required."
],
"source": [
"The source field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"body": {
"type": "array"
},
"source": {
"type": "array"
}
}
}
Create a questionPOST/questions
Body parameters
Name | Type | Description |
---|---|---|
string | Optional Between 1 and 255 characters in length |
|
first_name | string | Optional Between 1 and 255 characters in length |
body | string | Required Minimum 15 characters |
product_id | string | Optional Required if the type is 1 - product review |
type | integer | Required (1, 2) 1: product question, 2: website question |
source | integer | Required (1, 2) The platform where the question was left. 1: website page, 2: review widget |
Example create a question
POST /questions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"email": "lisa@example.com",
"first_name": "Lisa",
"body": "What are the key features and benefits of this product?",
"type": 1,
"source": 1,
"product_id": "5157526"
}
Question Replies ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <X-Application-Key>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Store-Domain: <store_domain>;
Body
{
"question_id": "603b051e-9ba0-49cf-a111-b9f622c5c9a6",
"body": "Reply",
"email": "john@example.com"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"question_id": {
"type": "string",
"description": "The question id that your are trying to reply to."
},
"body": {
"type": "string",
"description": "The reply content"
},
"email": {
"type": "string",
"description": "email address"
}
}
}
Headers
Content-Type: No Content
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 401,
"message": "Unauthenticated.",
"description": "Unauthenticated."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 403,
"message": "This action is unauthorized.",
"description": "This action is unauthorized."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"body": [
"The body field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"body": {
"type": "array"
}
}
}
Create a replyPOST/questions/replies
Body parameters
Name | Type | Description |
---|---|---|
body | string | Required Reply content |
string | Optional |
Example create a reply
POST /questions/603b051e-9ba0-49cf-a111-b9f622c5c9a6/replies HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
X-Application-Key: eyXai0XD7SLLzaOeJW
Accept: application/vnd.kangaroorewards.api.v1+json;
Content-Type: application/json
X-Store-Domain: example.com
{
"body": "Reply"
"email": "john@example.com"
}
Generated by aglio on 09 Oct 2024