Kangaroo Rewards - User API Reference
API Endpoint
https://api.kangaroorewards.comNote. If you are looking for Business API Reference, it is awailable at Business API Reference
Developers can build an app using Kangaroo Rewards API in their language of choice. To simplify integrations, Kangaroo Rewards provides REST Server SDKs and samples available on Github (in PHP).
KangarooRewards-PHP-SDK - the SDK without Oauth2 support (you will need to handle authentication and request an access token using another library, or by making API calls directly to the authentication endpoints)
OAuth2 Kangaroo Rewards - the OAuth2 Client for Kangaroo Rewards API
Build Your First App Following These Steps
-
Step 1: Register an app with Kangaroo Rewards
-
Step 2: Implement Authentication
-
Step 3: Access Kangaroo Rewards REST API
Prerequisite. To get started, you will need a Kangaroo Rewards Developer account with which to build and test your app. Sign up for free at: api.kangaroorewards.com/developers
App capabilities
Your app can do these things and more.
-
Access user account information
-
Update user profile
-
Access Business information including branches, offers, rewards etc.
-
Get user transactions
-
Redeem allowed rewards
Register an app with Kangaroo Rewards
Go to Developer Portal. Click on the “Register a new Application” button. Consider using a service account when registering your app at Developer Portal. i.e. “apps@yourcompany.com” - This will ensure your app continues to work if you or the app creator leaves the company.
Register a New App - Fields | Description |
---|---|
Application name | The app name which will appear on Authorization dialog. |
Grant Type | Select Password Grant - the only currently supported |
Authorization callback URL | This must be the page url where the users will be redirected after authorizing your application. Also, The Access Token will be sent to this page |
Application description | Optional. This is what the users will see on Authentication page |
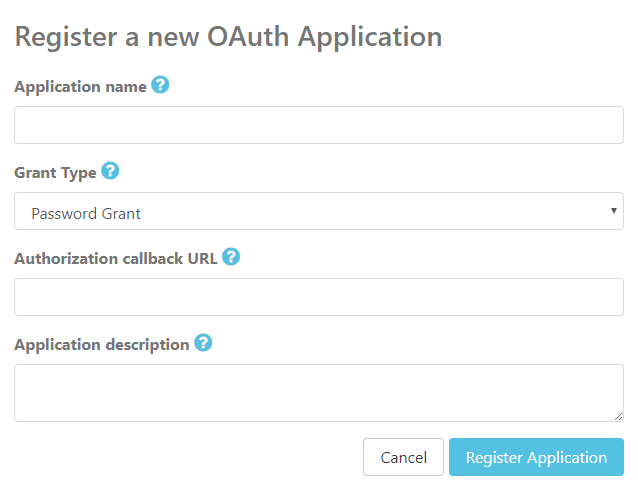
Note: Currently the only supported grant type is Password Grant. Select Password Grant type.
Once the application is created, it will have a Client ID and Client Secret associated to it. The Client ID is public, but Client Secret must not be shared.
Implement Authentication
OAuth 2 is an open standard for authorization that enables third-party applications to obtain limited access to Kangaroo Rewards user accounts, by delegating user authentication to Kangaroo Rewards.
All developers need to register their application before getting started. A registered OAuth application is assigned a unique Client ID and Client Secret. The Client Secret should not be shared.
OAuth - Password Credentials Grant
The Kangaroo Rewards OAuth API supports the Password Credentials Grant flow, where the resource owner has a trust relationship with the client.
This grant type is suitable for clients capable of obtaining the resource owner’s credentials (username and password, typically using an interactive form).
The application may access a Kangaroo Rewards API after it receives the access token.
Note: Your application should always use HTTPS in this scenario.
1. Requesting Tokens
Access Token Request Endpoint:
https://api.kangaroorewards.com/oauth/token
Once you have created an application with type password grant
, you may request an access token by issuing a POST request to
the /oauth/token route with the user’s email address and pin code. If the request is successful, you will receive an access_token
and refresh_token in the JSON response from the server.
Parameter | Values | Description |
---|---|---|
client_id | The client ID you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
client_secret | The client secret you obtain from the Developer Portal when you registered your app | Identifies the client that is making the request. The value passed in this parameter must exactly match the value shown in the Developer Portal |
grant_type | password | For password grant |
username | Email or phone number | The email or phone number |
password | The pin code | The user's pin code |
scope | `user` | Scopes allow the clients to request a specific set of permissions when requesting authorization to access an account |
application_key | The Application Key | The business owner has to provide The Application Key. He can get it from his business portal. |
Example Token Request
$http = new GuzzleHttp\Client;
$response = $http->post('https://api.kangaroorewards.com/oauth/token', [
'form_params' => [
'grant_type' => 'password',
'client_id' => '{YOUR_CLIENT_ID}',
'client_secret' => '{YOUR_CLIENT_SECRET}',
'username' => 'john@example.com',
'password' => 'john-super-password',
'application_key' => '{YOUR_APPLICATION_KEY}',
'scope' => 'user',
],
]);
$token = json_decode((string) $response->getBody(), true);
print_r($token);
A successful response is returned as a JSON array, similar to the following:
{
"access_token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImI5ZTk0NTRkYTRmOTY5Y2U2ZTUxMmFlMTRkZTdkMWJhZWQ1NjUyZTcwNmNiOTk2YTE2MjdhMjg0ZDMzMTM2NDNlZmJlZjhiZWU4YWQxMDg4In0",
"refresh_token":"2riNuUlbhlW1MWKb4Jos9FOYgIzz5ZJtK7rQjIgLDu3XsgblD5Xtn44sFieo",
"expires_in":3920,
"token_type":"Bearer"
}
2. Use Access Token
Now you may use the access token to make requests, on behalf of the user, from the resource server via the API (endpoint: https://api.kangaroorewards.com/users).
The access token may be used until it expires (24 hours after being issued) or is otherwise invalidated (e.g. user revoked or refresh token used).
Example Access Token Use
curl -H "Authorization: Bearer 12345678900987654321-abc34135acde13f13530" https://api.kangaroorewards.com/users/me
Refresh Token Flow
If you would like to request a new access token (and new refresh token), you may do so by sending the authorization server a token refresh request. A typical reason for refreshing a token is that the original access token has expired. A refresh token may only be used once, and using it invalidates the access token that it was issued with.
1. Use Refresh Token
Use the refresh_token in your token refresh request, which is a POST request to the token endpoint with the appropriate parameters.
https://api.kangaroorewards.com/oauth/token
Parameter | Values | Description |
---|---|---|
grant_type | refresh_token |
Must be set to “refresh_token” for an token refresh request. |
refresh_token | def502009d9337d94c8b7d67b05b9d05afb1b6... |
The refresh_token that was received with the original access token. |
client_id | Your client ID | The value passed in this parameter must exactly match the value shown in the Developer Portal |
client_secret | Your client secret | The client secret you obtain from the Developer Portal |
scope | user |
A list of scopes. Currently we support only one scope, “user” |
Token Refresh Response
The refresh token response looks just like the normal access token grant. It includes new access and refresh tokens.
Example Refresh Token Request
curl --request POST \
--url http://api.kangaroorewards.com/oauth/token \
--header 'content-type: application/x-www-form-urlencoded' \
--header 'x-application-key: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9' \
--data 'grant_type=refresh_token&refresh_token=def50200ceef1ff4664d517&client_id=1111&client_secret=cJ57RFk2CibH4o2INJb3L6&scope=user'
Scopes
Scopes limit the type of access that an access token will allow. The Kangaroo Rewards API currently supports one scope, “user”.
Name | Description |
---|---|
user | Manage user account |
Access Kangaroo Rewards REST API
The Kangaroo Rewards API allows you to manage resources in a simple, programmatic way using conventional HTTP requests. The endpoints are intuitive and powerful, allowing you to easily make calls to retrieve information.
All of the functionality that you are familiar with in the Kangaroo Rewards App is also available through the API, allowing you to script the complex actions that your situation requires.
Note: The X-Application-Key header is required for all requests
Requests
Method | Usage |
---|---|
GET | For simple retrieval of information about your account, offers, rewards etc., you should use the GET method. The information you request will be returned to you as a JSON object. The attributes defined by the JSON object can be used to form additional requests. Any request using the GET method is read-only and will not affect any of the objects you are querying. |
POST | To create a new object, your request should specify the POST method. The POST request includes all of the attributes necessary to create a new object. When you wish to create a new object, send a POST request to the target endpoint. |
PUT | To update the information about a resource in your account, the PUT method is available. |
DELETE | To destroy a resource and remove it from your account, the DELETE method should be used. This will remove the specified object if it is found. If it is not found, the operation will return a response indicating that the object was not found (404 error code). |
HTTP Statuses
Along with the HTTP methods that the API responds to, it will also return standard HTTP statuses, including error codes.
In the event of a problem, the status will contain the error code, while the body of the response will usually contain additional information about the problem that was encountered.
In general, if the status returned is in the 200
range, it indicates that the request was fulfilled
successfully and that no error was encountered.
Return codes in the 400
range typically indicate that there was an issue with the request that was sent.
Among other things, this could mean that you did not authenticate correctly, that you are requesting an action that you do not have authorization for, that the object you are requesting does not exist, or that your request is malformed.
If you receive a status in the 500
range, this generally indicates a server-side problem. This means that
we are having an issue on our end and cannot fulfill your request currently.
Responses
When a request is successful, a response body will typically be sent back in the form of a JSON object. An exception to this is when a DELETE request is processed, which will result in a successful HTTP 204 status and an empty response body.
Example Response
{
"data": {
"name": "john"
. . .
}
}
Meta
In addition to the main resource root, the response may also contain a meta object. This object contains information about the response itself. The meta object contains a total key that is set to the total number of objects returned by the request. This has implications on the pagination. The meta object will only be displayed when it has a value. Currently, the meta object will have a value when a request is made on a collection (like transactions).
Pagination
The cursor object is returned as part of the response body when pagination is enabled. By default, 100 objects are returned per page. You can request a different page by appending ?page= to the request. For instance, to navavigate to page 2, you could add ?page=2 to the end of your query
{
"data": {
. . .
},
"cursor": {
"count": 100,
"next": 2,
"next_uri": "/users/{id}/transactions?page=2"
},
"meta": {
"total": 509
}
}
Rate Limit
The number of requests that can be made through the API is currently limited to 60 per minute per OAuth token. The rate limiting information is contained within the response headers of each request. The relevant headers are:
-
X-RateLimit-Limit - The number of requests that can be made per minute.
-
X-RateLimit-Remaining - The number of requests that remain before you hit your request limit.
-
X-RateLimit-Reset - This represents the time when you are allowed to make requests again. The value
-
is given in Unix epoch time You’ll only get X-RateLimit-Reset if you’ve hit the limit
Example
X-RateLimit-Limit: 60
X-RateLimit-Remaining: 0
X-RateLimit-Reset: 1476571703
Users
Users ¶
User resource allows to list users, create a new user, update user.
This is an object representing an user account. You can retrieve it to see properties on the account like its current e-mail address, settings etc.
A User object has the following attributes:
-
id
(String)
: Unique identifier. -
first_name
(String)
: First Name. -
last_name
(String)
: Last Name. -
email
(String)
: Email address of the user. -
phone
(String)
: Phone number of the user. -
country_code
(String)
: The user’s phone number country, in ISO 3166-1-alpha-2 format. -
language
(String)
: Communication language. Possible valuesen
- English,fr
- French,es
- Spanish. -
gender
(String)
: Possible valuesmale
,female
. -
birth_date
(String)
: The user’s date of birth. -
pin_code
(Number)
: The user’s pin code.
User ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
X-Application-Key: <your_application_key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Headers
Content-Type: application/json
Body
{
"data": [
null
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all usersGET/users{?page}
Retrieve paginated list of users.
NOTE: Currently Not implemented
- page
number
(optional) Default: 1 Example: 2The page number.
User ¶
Headers
Content-Type: application/json
X-Application-Key: <your_application_key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"birth_date": "1963-03-28",
"language": "en",
"gender": "male"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
},
"email": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"language": {
"type": "string",
"description": "Communication language. Possible values `en` - English, `fr` - French, `es` - Spanish"
},
"gender": {
"type": "string",
"description": "User's gender. Can be male or female"
}
},
"required": [
"email"
]
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"first_name": "Battery",
"last_name": "Harris",
"email": "johndoe@example.com",
"phone": "5141234567",
"birth_date": "1963-03-28",
"gender": "male"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"first_name": {
"type": "string",
"description": "User's first name."
},
"last_name": {
"type": "string",
"description": "User's last name."
},
"email": {
"type": "string"
},
"phone": {
"type": "string"
},
"birth_date": {
"type": "string",
"description": "Date of birth"
},
"gender": {
"type": "string",
"description": "User's gender. Can be `male` or `female`"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"code": 400,
"error": "Bad Request",
"message": "Invalid ID supplied"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"code": {
"type": "number"
},
"error": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"email": [
"The email field is required"
],
"phone": [
"The phone field is required"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"email": {
"type": "array"
},
"phone": {
"type": "array"
}
}
}
Create a userPOST/users
Body parameters
Name | Type | Description |
---|---|---|
string | Required if no phone number provided | |
phone | string | Required if no email provided |
country_code | string | The user’s country, in ISO 3166-1-alpha-2 format. |
first_name | string | First Name |
last_name | string | Last Name |
language | string | Communication language. Possible values en - English, fr - French, es - Spanish |
gender | string | Possible values male , female |
birth_date | The user’s date of birth | |
pin_code | The user’s pin code |
Example
POST /users HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe",
"pin_code": 1111
}
Respone Body
{
"data": {
"id": "11e69610410894b2bb02089e01cf89b5",
"email": "johndoe@example.com",
"phone": "",
"first_name": "John",
"last_name": "Doe"
}
}
NOTE: If no PIN provided in request body then a PIN will be automatically generated.
- Currently only PIN is used for authentication. Password is not supported.
User ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
}
}
}
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
},
"included": {
"catalog_items": [
{
"id": "345",
"title": "$20 Discount",
"points": "3000",
"partner_reward": false,
"expires_at": "2027-06-27T02:59:59+00:00"
},
{
"id": "578",
"title": "Redeem 1 week at Palace Resort",
"points": "50000",
"partner_reward": true,
"expires_at": "2027-04-27T02:59:59+00:00"
}
],
"businesses": [
{
"id": "22e696103f8fa5eabb02089e01cf89c1",
"name": "Retrorant",
"loyalty_type": "per_visit",
"about": "Retrorant has cuisines in houses across the city.",
"logo": "57d23093693fe.jpg",
"cover": "5755ae54d57635.61967004.jpg"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
},
"included": {
"type": "object",
"properties": {
"catalog_items": {
"type": "array"
},
"businesses": {
"type": "array"
}
}
}
}
}
Retrieve a userGET/users/{id}{?include}
Example
GET /users/me HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
NOTE: You could also request more information using include parameter. All possible values:
- businesses - includes businesses for a coalition or conglomerate in the response
- branches - includes branches for business in the response
- catalog_items - includes catalog items in the response
- offers - includes published offers in the response
- coupons - includes coupons for user in the response
- giftcards - includes active gift cards in the response
- products - includes published products in the response
- social_media - includes social media links in the response
- coalition - includes coalition object in the response
- balance - includes user balance for business in the response
- user_emails - includes all user’s emails with status in the response
- user_phone_numbers - includes all user’s phone numbers with status in the response
The value of the include parameter MUST be a comma-separated (",") list of relationship names.
Example
https://api.kangaroorewards.com/users/me?include=businesses,catalog_items
NOTE:
coalition
parameter is applicable only for a coalition business not for a regular business
NOTE:
businesses
parameter is applicable only for a coalition or conglomerate business not for a regular business
NOTE: When
balance
is included andX-Application-Key
belongs to a coalition, then each business will have abalance
object that represents the authenticated user’sbalance
for the respective businesses. Also, the response includes the balance object that represents cumulative balance for all the businesses under the coalition
- id
string
(required) Example: meID of the User in form of a string
- include
enum
(optional) Example: businesses,catalog_itemsrelated resources to include in the response
User ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
}
}
}
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
},
"included": {
"user_emails": [
{
"email": "email1@example.com",
"status_code": 33,
"status_text: `EMAIL_VERIFIED`": "Hello, world!",
"created_at": "2017-06-30T15:45:18+00:00"
},
{
"email": "email1@example.com",
"status_code": 32,
"status_text: `EMAIL_NOT_VERIFIED`": "Hello, world!",
"created_at": "2017-06-30T15:43:58+00:00"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
},
"included": {
"type": "object",
"properties": {
"user_emails": {
"type": "array"
}
}
}
}
}
Update a userPATCH/users/{id}
Allows you to update e-mail address, name, phone number, PIN, settings etc.
Example
PATCH /users/me HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"email": "email1@example.com",
"first_name": "John",
"last_name": "Doe",
"pin_code": 1111
}
Example Update Profile Image
PATCH /users/me HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"profile_photo": "photos/user/67062_1500901818_5975f1ba4ad682.41305736.jpg",
}
NOTE: First upload the image by making a POST request to
/users/me/images
and send the image path when updating user profile to save the image path for authenticated user.
- id
string
(required) Example: meID of the User in form of a string
User ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Content-Type: multipart/form-data
Headers
Content-Type: application/json
Body
{
"data": {
"path": "photos\\/user\\/312270_1500856944_5975427069ad22.18650642.jpg"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"path": {
"type": "string"
}
}
}
}
}
Upload profile imagePOST/users/{id}/images
Allows you to upload user profile image
Example
POST /users/me/images HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="image"; filename="image.jpg"
Content-Type: image/jpeg
------WebKitFormBoundary7MA4YWxkTrZu0gW--
Example HTML
<form action="https://api.kangaroorewards.com/users/me/images" enctype="multipart/form-data" method="POST">
<input type="file" name="image" />
<button type="submit"> Upload </button>
</form>
Note: For this endpoint the
Content-Type
header must bemultipart/form-data
and the field name that contains the file isimage
- id
string
(required) Example: meID of the User in form of a string
User ¶
Headers
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e696103f8ff5eabb02089e01cf89b5",
"username": "johndoe",
"email": "johndoe@example.com",
"first_name": "John",
"last_name": "Doe"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"username": {
"type": "string"
},
"email": {
"type": "string"
},
"first_name": {
"type": "string"
},
"last_name": {
"type": "string"
}
}
}
}
}
Verify user credentialsPOST/users/credentials/verification
Allows you to verify user’s email or phone number using the token received in the welcome email or text message
Note: The
Authorization
header is not required, onlyX-Application-Key
header is required
Example. Verify user’s email address.
POST /users/credentials/verification HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"token": "<verification-token>",
"email": "john@example.com"
}
Example. Verify user’s phone number’.
POST /users/credentials/verification HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"token": "<verification-token>",
"phone": "514-111-1111",
"country_code": "CA"
}
User ¶
Headers
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"status_code": 202,
"message": "Accepted",
"description": "You will receive an email with a link to reset your PIN."
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
Reset PIN requestPOST/users/pincode/reset-request
An email or text message will be sent to included email/phone number with a token or verification code.
Note: The
Authorization
header is not required, onlyX-Application-Key
header is required
Body parameters
Name | Type | Description |
---|---|---|
mode | string | Required. token or verification_code |
string | Required if no phone nuber provided | |
phone | string | Required if no email provided |
country_code | string | Required with phone number. The user’s country, in ISO 3166-1-alpha-2 format. |
Example. Reset PIN request with mode token and email
POST /users/pincode/reset-request HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"email": "john@example.com",
"mode": "token"
}
An email will be sent with link and token. The user should click the link to reset his/her PIN code.
Example. Reset PIN request with mode verification code and phone number
POST /users/pincode/reset-request HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"phone": "514-111-1111",
"country_code": "CA",
"mode": "verification_code"
}
A text message will be sent with a verification code. The user should use it to reset his/her PIN code.
User ¶
Headers
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Content-Type: application/json
Headers
Content-Type: application/json
Headers
Content-Type: application/json
Body
{
"verification_code": [
"The selected verification code is invalid."
],
"token": [
"The selected token is invalid."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"verification_code": {
"type": "array"
},
"token": {
"type": "array"
}
}
}
Reset PINPOST/users/pincode/reset
Allows to reset a user’s PIN code. The token or verification code and user credentials is required.
Note: The
Authorization
header is not required, onlyX-Application-Key
header is required
Note: When token provided, the user credentials are not required. User’s email or phone number is required only when verification code provided
Body parameters
Name | Type | Description |
---|---|---|
token | string | Required if no verification_code provided |
verification_code | number | Required if no token provided |
pin_code | number | Required. The new PIN code |
string | Required if no phone nuber provided and verification_code included | |
phone | string | Required if no email provided and verification_code included |
country_code | string | Required with phone number. The user’s country, in ISO 3166-1-alpha-2 format. |
Example. Reset PIN using verification code and phone number.
POST /users/pincode/reset HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"verification_code": 1617,
"phone": 514-111-1111,
"country_code": "CA",
"pin_code": 1111
}
Example. Reset PIN using a token
POST /users/pincode/reset HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"token": "1501011980.5478MTF7djiC7ZbgTqG1VsKycmQkVvpEN18QcyaBh3RSqAniZ",
"pin_code": 1111
}
Example. Reset PIN using verification code and email
POST /users/pincode/reset HTTP/1.1
Host: api.kangaroorewards.com
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"verification_code": 9993,
"email": "john@example.com",
"pin_code": 1111
}
Rewards ¶
Reward ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"custom_rewards": [
{
"id": "123",
"title": "Download Kangaroo App and Earn",
"points": "150"
}
],
"catalog_items": [
{
"id": "345",
"title": "$20 Discount",
"points": "3000",
"partner_reward": false,
"expires_at": "2027-06-27T02:59:59+00:00"
},
{
"id": "578",
"title": "Redeem 1 week at Palace Resort",
"points": "50000",
"partner_reward": true,
"expires_at": "2027-04-27T02:59:59+00:00"
}
]
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"custom_rewards": {
"type": "array"
},
"catalog_items": {
"type": "array"
}
}
}
}
}
List all rewardsGET/users/{user_id}/rewards
Provides the details for all of a business’s rewards.
Example
GET /users/user_id/rewards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"custom_rewards": [
{
"id": 35,
"points": 500,
"title": "Download Kangaroo App and Earn",
"description": "",
"images": []
}
],
"catalog_items": [
{
"id": 135,
"points": 2000,
"title": "$20 Discount",
"description": "",
"images": []
}
]
}
}
- user_id
string
(required) Example: meID of the User
Offers ¶
Offer ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Offer Title",
"points": "3000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
},
{
"id": "112",
"title": "Offer Title 2",
"points": "50000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"multip_factor": 0
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all offersGET/users/{user_id}/offers
Provides the details for all of a business’s offers.
Path Parameters
Name | Type | Description |
---|---|---|
user_id | string | Required. The ID of the user or me - currently auhenticated user |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include the branch object. To do so, specify relationships=branch in your request. |
Example
GET /users/me/offers HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
]
}
]
}
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object in the response
The value of the relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /users/me/offers?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
}
}
- user_id
string
(required) Example: meID of the User
Offer ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "111",
"title": "Offer Title",
"points": "3000",
"type": "free_product",
"description": "Some Offer Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"multip_factor": 2
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"title": {
"type": "string"
},
"points": {
"type": "string"
},
"type": {
"type": "string"
},
"description": {
"type": "string"
},
"expires_at": {
"type": "string"
},
"multip_factor": {
"type": "number"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Offer 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Retrieve an offerGET/users/{user_id}/offers/{offer_id}
Returns a single offer.
Path Parameters
Name | Type | Description |
---|---|---|
me | string | Required. The ID of the user or me - auhenticated user |
offer_id | number | Required. The ID of the offer |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include related objects. To do so, specify for example: relationships=branch in your request. |
Example
GET /users/me/offers/{offer_id}?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
}
}
- user_id
string
(required) Example: meID of the User
- offer_id
string
(required) Example: 123The unique ID of the offer.
Gift Cards ¶
Giftcard ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "111",
"title": "Giftcard Title 1",
"points": "2000",
"type": "giftcard",
"description": "Some Giftcard Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"discount_value": 20,
"real_value": 20
},
{
"id": "112",
"title": "Giftcard Title 2",
"points": "50000",
"type": "giftcard",
"description": "Some Giftcard Description",
"expires_at": "2027-04-27T02:59:59+00:00",
"discount_value": 50,
"real_value": 50
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all Gift CardsGET/users/{user_id}/giftcards
Provides the details for all of a business’s giftcards.
Path Parameters
Name | Type | Description |
---|---|---|
user_id | string | Required. The ID of the user or me - currently auhenticated user |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include the branch object. To do so, specify relationships=branch in your request. |
Example
GET /users/me/giftcards HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "giftcard",
"title": "Giftcard Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
]
}
]
}
NOTE: You could also request more information using relationship parameter. All possible values:
- branch - includes branch object in the response
The value of the relationships parameter MUST be a comma-separated (",") list of relationship names.
Example
GET /users/me/giftcards?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "giftcard",
"title": "Giftcard Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
}
}
- user_id
string
(required) Example: meID of the User
Giftcard ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "111",
"title": "Giftcard Title",
"points": "5000",
"type": "giftcard",
"description": "Some Giftcard Description",
"expires_at": "2027-06-27T02:59:59+00:00",
"discount_value": 50,
"real_value": 50
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"title": {
"type": "string"
},
"points": {
"type": "string"
},
"type": {
"type": "string"
},
"description": {
"type": "string"
},
"expires_at": {
"type": "string"
},
"discount_value": {
"type": "number"
},
"real_value": {
"type": "number"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Giftcard 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Retrieve a Gift CardGET/users/{user_id}/giftcards/{giftcard_id}
Returns a single giftcard.
Path Parameters
Name | Type | Description |
---|---|---|
me | string | Required. The ID of the user or me - auhenticated user |
giftcard_id | number | Required. The ID of the giftcard |
Query Parameters
Name | Type | Description |
---|---|---|
relationships | enum | Optional. You can optionally request that the response include related objects. To do so, specify for example: relationships=branch in your request. |
Example
GET /users/me/giftcards/{giftcard_id}?relationships=branch HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": 123,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "giftcard",
"title": "Giftcard Title",
"description": "",
"images": [
{
"default": true,
"path": "1498064009_594aa4895e8c7.jpg"
}
],
"branch": {
"id": "11e6a34f8e89eb0e9708089e01cf89b5",
"name": "Pizza Nova",
"web_site": null,
"logo": {
"large": null,
"medium": null,
"thumbnail": null
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
}
}
}
}
- user_id
string
(required) Example: meID of the User
- giftcard_id
string
(required) Example: 123The unique ID of the giftcard.
Businesses ¶
Business ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": "11e72ab54e056acc90ac089e01cf89b5",
"name": "Home Heating Company",
"loyalty_type: `per_spend`": "Hello, world!",
"about": "About us ...",
"category": {
"id": 3,
"name": "Bars",
"code": "FRONTEND_BUS_CAT_SERVICES"
},
"logo": {
"large": "\\large\\5900f316708678.24867196.jpg",
"medium": "\\medium\\5900f316708678.24867196.jpg",
"thumbnail": "\\thumbnail\\5900f316708678.24867196.jpg"
},
"cover_photo": {
"large": "\\large\\5941858e71c90.jpg",
"medium": "\\medium\\5941858e71c90.jpg",
"thumbnail": "\\thumbnail\\5941858e71c90.jpg"
}
},
{
"id": "12e32ab54e046acc90ac089e01cf89b2",
"name": "Another Company",
"loyalty_type: `per_spend`": "Hello, world!",
"about": "About us ...",
"category": {
"id": 1,
"name": "Services",
"code": "FRONTEND_BUS_CAT_SERVICES"
},
"logo": {
"large": "\\large\\1200f316708678.24867196.jpg",
"medium": "\\medium\\1200f316708678.24867196.jpg",
"thumbnail": "\\thumbnail\\1200f316708678.24867196.jpg"
},
"cover_photo": {
"large": "\\large\\5941858e71c90.jpg",
"medium": "\\medium\\5941858e71c90.jpg",
"thumbnail": "\\thumbnail\\5941858e71c90.jpg"
}
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all businessesGET/users/{user_id}/businesses
Provides the list of businesses.
Note: It will return a list of businesses only for a coalition or conglomerate. For a regular business the list will be always empty.
Example
GET /users/me/businesses HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": "11e72ab54e056acc90ac089e01cf89b5",
"name": "Home Heating Company",
"loyalty_type": "per_spend",
"about": " We specialize in home heating by providing the security and benefit of 24/7 emergency service",
"logo": {
"large": "1493234454_5900f316708678.24867196.jpg",
"medium": "1493234454_5900f316708678.24867196.jpg",
"thumbnail": "493234454_5900f316708678.24867196.jpg"
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
},
"category": {
"id": 2,
"name": "Services",
"code": "FRONTEND_BUS_CAT_SERVICES"
}
}
]
}
- user_id
string
(required) Example: meID of the User
Business ¶
Headers
Content-Type: application/json
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": {
"id": "11e72ab54e056acc90ac089e01cf89b5",
"name": "Home Heating Company",
"loyalty_type: `per_spend`": "Hello, world!",
"about": "About us ...",
"category": {
"id": 3,
"name": "Bars",
"code": "FRONTEND_BUS_CAT_SERVICES"
},
"logo": {
"large": "\\large\\5900f316708678.24867196.jpg",
"medium": "\\medium\\5900f316708678.24867196.jpg",
"thumbnail": "\\thumbnail\\5900f316708678.24867196.jpg"
},
"cover_photo": {
"large": "\\large\\5941858e71c90.jpg",
"medium": "\\medium\\5941858e71c90.jpg",
"thumbnail": "\\thumbnail\\5941858e71c90.jpg"
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"name": {
"type": "string"
},
"loyalty_type: `per_spend`": {
"type": "string"
},
"about": {
"type": "string"
},
"category": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"name": {
"type": "string"
},
"code": {
"type": "string"
}
}
},
"logo": {
"type": "object",
"properties": {
"large": {
"type": "string"
},
"medium": {
"type": "string"
},
"thumbnail": {
"type": "string"
}
}
},
"cover_photo": {
"type": "object",
"properties": {
"large": {
"type": "string"
},
"medium": {
"type": "string"
},
"thumbnail": {
"type": "string"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Business 11e72abc90ac089e01cf89b5 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Retrieve a businessGET/users/{user_id}/businesses/{business_id}
Returns a single business.
Path Parameters
Name | Type | Description |
---|---|---|
business_id | string | Required. The ID of the business |
Query Parameters
Name | Type | Description |
---|---|---|
include | enum | Optional. You can optionally request that the response include the related objects. To do so, specify for example: include=offers,products in your request. |
Example
GET /users/me/businesses/{business_id} HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": "11e72ab54e056acc90ac089e01cf89b5",
"name": "Home Heating Company",
"loyalty_type": "per_spend",
"about": " We specialize in home heating by providing the security and benefit of 24\/7 emergency service",
"logo": {
"large": "1493234454_5900f316708678.24867196.jpg",
"medium": "1493234454_5900f316708678.24867196.jpg",
"thumbnail": "493234454_5900f316708678.24867196.jpg"
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
},
"category": {
"id": 2,
"name": "Services",
"code": "FRONTEND_BUS_CAT_SERVICES"
}
}
}
NOTE: You could also request more information using include parameter. All possible values:
- offers - include business offers in the response
- products - include products in the response
- giftcards - include gift cards in the response
- catalog_items - include Redemption Catalog in the response
- social_media - include social media links in the response
- branches - include business branches in the response
Example with include
GET /users/me/businesses/{business_id}?include=offers,catalog_items,products HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": {
"id": "11e72ab54e056acc90ac089e01cf89b5",
"name": "Home Heating Company",
"loyalty_type": "per_spend",
"about": " We specialize in home heating by providing the security and benefit of 24/7 emergency service",
"logo": {
"large": "1493234454_5900f316708678.24867196.jpg",
"medium": "1493234454_5900f316708678.24867196.jpg",
"thumbnail": "493234454_5900f316708678.24867196.jpg"
},
"cover_photo": {
"large": null,
"medium": null,
"thumbnail": null
},
"category": {
"id": 2,
"name": "Services",
"code": "FRONTEND_BUS_CAT_SERVICES"
}
},
"included": {
"offers": [
{
"id": 574,
"points": 0,
"expires_at": "2017-07-01T03:59:59+0000",
"discount_value": 0,
"multip_factor": 0,
"real_value": 0,
"type": "free_product",
"title": "Offer for Home heating",
"description": "",
"images": []
}
],
"products": [],
"catalog_items": [
{
"id": 579,
"points": 100,
"expires_at": "2027-04-26T19:21:22+00:00",
"amount": 0,
"real_value": 0,
"partner_reward": false,
"type": "redeem_discount_amount",
"title": "1$ Discount",
"description": "",
"images": []
}
]
}
}
- user_id
string
(required) Example: meID of the User
- business_id
string
(required) Example: 123The unique ID of the business.
Transactions ¶
Transaction ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: <your_application_key>
Headers
Content-Type: application/json
Body
{
"data": [
{
"id": 256551,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000"
},
{
"id": 256552,
"amount": 30,
"points": 30,
"name": "Reward",
"created_at": "2017-06-23T17:15:41+0000"
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "array"
}
}
}
List all transactionsGET/users/{user_id}/transactions
Example
GET /users/me/transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
Response Body
{
"data": [
{
"id": 256551,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000"
},
{
"id": 256552,
"amount": 30,
"points": 30,
"name": "Reward",
"created_at": "2017-06-23T17:15:41+0000"
}
]
}
- user_id
string
(required) Example: meID of the User
Transaction ¶
Headers
Authorization: Bearer <access_token>
Accept: application/vnd.kangaroorewards.api.v1+json
Content-Type: application/json
X-Application-Key: <your_application_key>
Body
{
"intent": "redeem",
"catalog_items": [
{
"id": 123,
"quantity": 3
},
{
"id": 124,
"quantity": 1
}
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "string"
},
"catalog_items": {
"type": "array"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": 256559,
"amount": 5.25,
"points": 5,
"name": "Reward",
"created_at": "2017-03-06T19:45:47+0000"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"amount": {
"type": "number"
},
"points": {
"type": "number"
},
"name": {
"type": "string"
},
"created_at": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"intent": [
"The intent field is required."
],
"catalog_items": [
"The catalog items field is required."
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "array"
},
"catalog_items": {
"type": "array"
}
}
}
Create a transactionPOST/users/{user_id}/transactions
A Transaction is a single event where a customer earns or spends points.
Also, you can retrieve a specific transaction or a list of transactions.
Use /users/transactions
resource to create a reward, or redeem transaction.
A reward
transaction will increase the user’s balance.
A redeem
transaction will decrease the user’s balance.
Include catalog_items
object when you want to redeem a “Redemption Catalog” item. To be used for redeem
transaction only.
Body parameters
Name | Type | Description |
---|---|---|
intent | string | Set the intent to: reward or redeem |
catalog_items | object | Required. The Catalog Item to be redeemed |
catalog_items.id | string | Required. The ID of the Catalog Item |
catalog_items.quantity | number | Required. The quantity |
Example Redeem a Catalog Item
POST /users/transactions HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyXai0XD7SLLzaOeJW
Content-Type: application/json
{
"intent": "redeem",
"catalog_items": [
{
"id": 123,
"quantity": 1
}
]
}
- user_id
string
(required) Example: meID of the User
Payments ¶
Payment ¶
Headers
Content-Type: application/json
X-Application-Key: <your_application_key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"intent": "buy_giftcard",
"provider": "paypal",
"giftcard_id": 1234,
"paypal_return_url": "https://example.com/payment/paypal?success=true",
"paypal_cancel_url": "https://example.com/payment/paypal?success=false"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "string"
},
"provider": {
"type": "string"
},
"giftcard_id": {
"type": "number"
},
"paypal_return_url": {
"type": "string"
},
"paypal_cancel_url": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": "PAY-13T29792VT6854806LJVZSYY",
"provider": "paypal",
"status": "created",
"approval_url: `https://www.sandbox.paypal.com/cgi-bin/webscr?cmd=_express": "Hello, world!",
"giftcard": {
"id": 1234,
"title": "50$ Gift Card",
"price": 50,
"currency": "$",
"quantity": 1
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"provider": {
"type": "string"
},
"status": {
"type": "string"
},
"approval_url: `https://www.sandbox.paypal.com/cgi-bin/webscr?cmd=_express": {
"type": "string",
"description": "checkout&token=EC-4863717343954603X` (string)"
},
"giftcard": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"title": {
"type": "string"
},
"price": {
"type": "number"
},
"currency": {
"type": "string"
},
"quantity": {
"type": "number"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"code": 400,
"error": "Bad Request",
"message": "Invalid ID supplied"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"code": {
"type": "number"
},
"error": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Gift Card 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"giftcard_id": [
"The giftcard_id field is required"
],
"intent": [
"The intent field is required"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"giftcard_id": {
"type": "array"
},
"intent": {
"type": "array"
}
}
}
Create a paymentPOST/users/{user_id}/payments
Body parameters
Name | Type | Description |
---|---|---|
intent | string | Required. Must be buy_giftcard |
provider | string | Required. Must be paypal |
giftcard_id | number | Required. The gift card ID the payment will be created for |
paypal_return_url | string | Required. The return url after payment is completed |
paypal_cancel_url | string | Required. The return url for canceling a payment |
Example
POST /users/me/payments HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"intent": "buy_giftcard",
"provider": "paypal",
"giftcard_id": 1192,
"paypal_return_url": "https://example.com/payment/paypal?success=true",
"paypal_cancel_url": "https://example.com/payment/paypal?success=false"
}
Respone Body
{
"data": {
"id": "PAY-13T29792VT6854806LJVZSYY",
"provider": "paypal",
"status": "created",
"approval_url": "https://www.sandbox.paypal.com/cgi-bin/webscr?cmd=_express-checkout&token=EC-4863717343954603X",
"giftcard": {
"id": 1192,
"title": "50$ Gift Card",
"price": 50,
"currency": "$",
"quantity": 1
}
}
}
NOTE: Currently only PayPal provider is supported to handle payments.
Once the payment is created the user must be redirected to approval_url
- user_id
string
(required) Example: meID of the User
Purchases ¶
Purchase ¶
Headers
Content-Type: application/json
X-Application-Key: <your_application_key>
Accept: application/vnd.kangaroorewards.api.v1+json;
Body
{
"intent": "buy_giftcard",
"provider": "paypal",
"giftcard_id": 1234,
"paypal_payment": {
"success": true,
"payment_id": "PAY-3AP80704W8500213CLJVBDWY",
"payer_id": "U72GC72EJ9DYS",
"token": "EC-6P663797EV1432456"
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"intent": {
"type": "string"
},
"provider": {
"type": "string"
},
"giftcard_id": {
"type": "number"
},
"paypal_payment": {
"type": "object",
"properties": {
"success": {
"type": "boolean"
},
"payment_id": {
"type": "string"
},
"payer_id": {
"type": "string"
},
"token": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"data": {
"id": "PAY-13T29792VT6854806LJVZSYY",
"provider": "paypal",
"status": "paid",
"giftcard": {
"id": 1234,
"title": "50$ Gift Card",
"price": 50,
"currency": "$",
"quantity": 1
},
"balance": {
"points": 1000,
"giftcard": 50
}
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"data": {
"type": "object",
"properties": {
"id": {
"type": "string"
},
"provider": {
"type": "string"
},
"status": {
"type": "string"
},
"giftcard": {
"type": "object",
"properties": {
"id": {
"type": "number"
},
"title": {
"type": "string"
},
"price": {
"type": "number"
},
"currency": {
"type": "string"
},
"quantity": {
"type": "number"
}
}
},
"balance": {
"type": "object",
"properties": {
"points": {
"type": "number"
},
"giftcard": {
"type": "number"
}
}
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"code": 400,
"error": "Bad Request",
"message": "Invalid ID supplied"
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"code": {
"type": "number"
},
"error": {
"type": "string"
},
"message": {
"type": "string"
}
}
}
Headers
Content-Type: application/json
Body
{
"error": {
"status_code": 404,
"message": "Gift Card 112 Not Found",
"description": "The requested resource doesn't exist."
}
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"error": {
"type": "object",
"properties": {
"status_code": {
"type": "number"
},
"message": {
"type": "string"
},
"description": {
"type": "string"
}
}
}
}
}
Headers
Content-Type: application/json
Body
{
"giftcard_id": [
"The giftcard_id field is required"
],
"intent": [
"The intent field is required"
]
}
Schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"type": "object",
"properties": {
"giftcard_id": {
"type": "array"
},
"intent": {
"type": "array"
}
}
}
Create a purchasePOST/users/{user_id}/purchases
Body parameters
Name | Type | Description |
---|---|---|
intent | string | Required. Must be buy_giftcard |
provider | string | Required. Must be paypal |
giftcard_id | number | Required. The gift card ID the purchase will be created for |
paypal_payment | object | Required. The payment info |
paypal_payment.success | boolean | Required. The status for payment |
paypal_payment.payment_id | string | Required. The payment ID |
paypal_payment.token | string | Required. The payment token |
Example
POST /users/me/purchases HTTP/1.1
Host: api.kangaroorewards.com
Authorization: Bearer eyJ0eXAiOiJKV1QiLC
Accept: application/vnd.kangaroorewards.api.v1+json;
X-Application-Key: eyCrhTxfOphmbdWSkonGADcgrsCDa
Content-Type: application/json
{
"intent": "buy_giftcard",
"provider": "paypal",
"giftcard_id": 1192,
"paypal_payment": {
"success" : true,
"payment_id": "PAY-3AP80704W8500213CLJVBDWY",
"payer_id": "U72GC72EJ9DYS",
"token": "EC-6P663797EV1432456"
}
}
Respone Body
{
"data": {
"id": "PAY-3AP80704W8500213CLJVBDWY",
"provider": "paypal",
"status": "paid",
"giftcard": {
"id": 1192,
"name": "50$ Gift Card",
"price": 50,
"currency": "$",
"quantity": 1
},
"balance": {
"points": 1000,
"giftcard": 50
}
}
}
NOTE: A create purchase request must be sent only after the payment finished.
- user_id
string
(required) Example: meID of the User
Generated by aglio on 17 Jul 2018